Question
You will need to implement lookup, which returns a boolean indicating whether the target was found, insert, which adds an item to the BST, remove,
You will need to implement lookup, which returns a boolean indicating whether the target was found, insert, which adds an item to the BST, remove, which removes an item from the BST, and pre-, in-, post-, and level-order traversals which prints the tree in the appropriate order. For example, in C++, the function headers would be the following:
class BST { Node *root; public: BST() { // ... } bool lookup(int target) { // ... } void insert(int data) { // ... } void preOrder() { // ... } void postOrder() { // ... } void inOrder() { // ... } void levelOrder() { // ... } void remove(int target) { // ... } };
Input Format
The first line of the input will be an integer n indicating how many commands follow it. The next n lines will consist of one of 7 commands ("insert", "lookup", "delete", "preorder", "postorder", "levelorder", and "inorder"). The commands "insert", "lookup", and "delete" will be followed by a space and an integer to perform the command on. Note that the argument to "delete" may not be in the BST, in which case you should do nothing. At the end of the input there will be a blank line. Your program should initialize an empty BST and perform the commands given by the input, in sequence, on it. For example, valid input might be:
20 lookup 5 insert 5 lookup 5 insert 18 insert 3 insert 12 preorder insert 19 lookup 20 delete 20 lookup 20 postorder insert 25 insert 6 insert 1 inorder lookup 18 delete 18 lookup 18 postorder
Constraints
1 <= n <= 100 (You will be asked to perform between 1 and 100 commands, inclusive, on your BST, per test case.) You can also assume there will be no invalid input. You can assume the BST will consist only of positive integers with no duplicates.
Output Format
For the "lookup" command, print the line "0" if the target is not found and the line "1" if it is found. For the traversal ("preorder", "postorder", "inorder", "levelorder") commands, print a line containing the space-separated traversal (the line will end in a space). For the "insert" and "delete" commands, print nothing. For example, the appropriate output for the sample input is:
0 1 5 3 18 12 0 0 3 12 19 18 5 1 3 5 6 12 18 19 25 1 0 1 3 6 12 25 19 5
Sample Input 0
20 lookup 5 insert 5 lookup 5 insert 18 insert 3 insert 12 preorder insert 19 lookup 20 delete 20 lookup 20 postorder insert 25 insert 6 insert 1 inorder lookup 18 delete 18 lookup 18 postorder
Sample Output 0
0 1 5 3 18 12 0 0 3 12 19 18 5 1 3 5 6 12 18 19 25 1 0 1 3 6 12 25 19 5
Here's what I have so far please help
#include
#include
using namespace std;
struct Node {
int data;
Node *left;
Node *right;
};
class BST {
Node *root;
public:
BST() {
root = nullptr;
}
bool lookup(int target) {
}
void insert(int data) {
}
void preOrder() {
}
void postOrder() {
}
void inOrder() {
}
void levelOrder() {
}
void takeOut(int target) {
}
};
int main()
{
int n;
cin >> n;
cin.ignore();
for (int i = 0; i < n; i++) {
string rawInput;
vector
string word = "";
getline(cin, rawInput);
for (int j = 0; j < rawInput.size(); j++) {
if (rawInput[j] == ' ') {
words.push_back(word);
word = "";
} else {
word = word + rawInput[j];
if (j == rawInput.size()-1) {
words.push_back(word);
}
}
}
// if (words[0] == "insert") {
// insert(words[1]);
// } else if (words[0] == "lookup") {
// lookup(words[1]);
// } else if (words[0] == "delete") {
// takeOut(words[1]);
// } else if (words[0] == "prorder") {
// preOrder();
// } else if (words[0] == "postorder") {
// postOrder();
// } else if (words[0] == "levelorder") {
// levelOrder();
// } else if (words[0] == "inorder") {
// inOrder();
// }
}
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
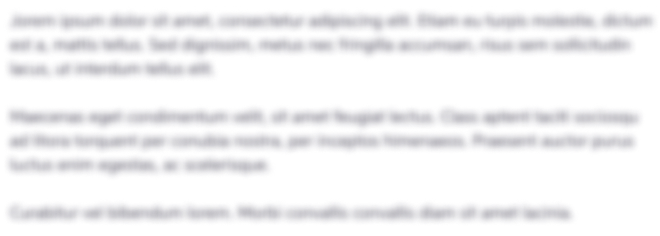
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started