Question
You will work with 4 classes in this lab. Employee.java (Completed) HourlyEmployee.java SalariedEmployee.java HumanResource.java (Completed) Employee is the superclass, and both HourlyEmployee and SalariedEmployee extend
You will work with 4 classes in this lab.
Employee.java (Completed)
HourlyEmployee.java
SalariedEmployee.java
HumanResource.java (Completed)
Employee is the superclass, and both HourlyEmployee and SalariedEmployee extend Employee class.
(1) Complete the definition of SalariedEmployee class following the instructions in the code.
(2) To allow this company always pays its employees weekly, the getWeeklyPay() method is added to Employee, HourlyEmployee and SalariedEmployee. The method in Employee class has been completed. You need to complete the method definition in HourlyEmployee class and SalariedEmployee class.
(3) In HumanResource.java, an ArrayList of Employee objects are created, and two HourlyEmployee objects and two SalariedEmployee objects are added to the array list. Run the program and check out the output to understand how polymorphism works.
Employee.java (Completed):
public class Employee { private String name; private String department; private String id; // Default constructor. Set protected variables to the empty string or 0 public Employee() { name = ""; department = ""; id = ""; } // Constructor with parameters to set the private variables public Employee(String name, String department, String id) { this.name = name; this.department = department; this.id = id; } public String getName() { return name; } public String getDepartment() { return department; } public String getId() { return id; } public void setDepartment(String department) { this.department = department; } public double getWeeklyPay() { return 0.0; } public String toString() { return "Name: " + name + ", Department: " + department + ", ID: " + id; } }
HourlyEmployee.java
import java.util.Scanner; public class HourlyEmployee extends Employee{ private double hourlyRate; public HourlyEmployee() { hourlyRate = 0.0; } public HourlyEmployee(String name, String department, String id, double rate) { super(name, department, id); hourlyRate = rate; } public double getHourlyRate() { return hourlyRate; } public void setHourlyRate(double rate) { hourlyRate = rate; } @Override public double getWeeklyPay() { double pay = 0.0; int hours; Scanner scnr = new Scanner(System.in); System.out.println("Enter the total number of hours worked:"); hours = scnr.nextInt(); /*Complete this method definition by asking user to enter the number of hours worked. If there is no overtime (worked less than or equal to 40 hours), we use the regular hourlyRate to calculate the weekly payment. Otherse, the overtime rate is 1.5 time of the regular hourly rate.*/ } @Override public String toString() { String result = super.toString(); result += ", Hourly Rate: $" + hourlyRate; return result; } }
SalariedEmployee.java
public class SalariedEmployee extends Employee{ private double annualSalary; /*FIXME(1) Complete the default constructor to set annualSalary to 0.0*/ public SalariedEmployee(salary) {
} /*FIXME(2) Complete the argumented constructor to set each field properly Note that you can call the super class constructor to initialize the fields defined in the super class*/ public SalariedEmployee(String name, String department, String id, double salary) { } /*FIXME(3) Complete the definition of the accessor for annualSalary*/ public double getAnnualSalary() { } /*FIXME(4) Complete the definition of the mutator for annualSalary*/ public void setAnnualSalary(double salary) { } /*FIXME(5) Complete the definition of getweeklyPay(). Assume that there are 52 weeks per year.*/ @Override public double getWeeklyPay() { } /*FIXME(6) Complete the definition of the toString method, which will return a String includes name, department, id, and annualSalary of the employee Note that you can call the toString() method defined in the superclass. Example: Name: Kevin Johnson, Department: Accounting, ID: kjohnson1, Annual Salary: $60000.0*/ @Override public String toString() { } }
HumanResource.java (Completed)
import java.util.ArrayList; public class HumanResource{ public static void main(String[] args) { ArrayList
Step by Step Solution
There are 3 Steps involved in it
Step: 1
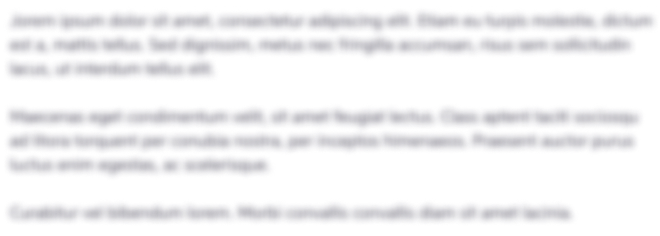
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started