Question
You will write a function to access a file of earthquake data and create a list of the magnitudes of the earthquakes in the file,
You will write a function to access a file of earthquake data and create a list of the magnitudes of the earthquakes in the file, then another function to analyze the magnitudes data. Finally, you will write a function to report the count, mean, median, mode, and frequency occurrences of the earthquake magnitudes.
Write three new functions: equake_readf, equake_analysis, and equake_report.
Function equake_readf will have one parameter, fname, a string, which is the name of the earthquake file. equake_readf should open file fname and create and return a list of the earthquake magnitudes from this file.
Function equake_analysis will have one parameter, magnitudes, the list of earthquake magnitudes (the list returned by equake_readf). equake_analysis will call functions from the data analysis file (project 6-2) to determine the mean, median, and mode of the data in the magnitudes list, and then return this result as a tuple.
Function equake_report will have two parameters, mmm (the tuple returned by equake_analysis) and magnitudes (the list returned by equake_readf), and return None. It will report the number (count) of earthquakes, and the mean, median, and mode of magnitudes. It will also call frequencyTable to report the number of occurrences of each item in magnitudes. When you have written and tested these, write function main to call functions equake_readf, equake_analysis, and equake_report. main returns None. main should look similar to this:
def main():
'''()-> None
Calls: equake_readf, equake_analysis, equake_report Top level function for earthquake data analysis. Returns None.
'''
#fname = 'equakes25f.txt'
fname = 'equakes50f.txt'
#fname = 'equakes_short.txt'
emags = equake_readf(fname)
mmm = equake_analysis(emags)
equake_report(emags, mmm)
return None
Include code in your .py file to call the main function.
given files:
equakes50f.txt
time,latitude,longitude,depth,mag,magType,nst,gap,dmin,rms,net,id,updated,place,type,horizontalError,depthError,magError,magNst,status,locationSource,magSource 2010-07-28T16:12:05.610Z,43.756,-125.815,10,5.2,mwc,193,143.9,,0.93,us,usp000hh0t,2017-08-01T16:34:36.951Z,"off the coast of Oregon",earthquake,,,,,reviewed,us,gcmt 1993-12-04T22:15:19.720Z,42.2915,-122.0086667,4.797,5.1,md,126,113,,0.11,uw,uw10316468,2017-04-13T22:06:07.852Z,"Oregon",earthquake,0.468,0.56,0.04,7,reviewed,uw,uw 1993-09-21T05:45:35.230Z,42.3575,-122.0583333,8.53,6,md,234,128,,0.08,uw,uw10313838,2018-02-23T23:02:14.153Z,"Oregon",earthquake,0.4,0.85,0.02,3,reviewed,uw,uw 1993-09-21T03:28:55.630Z,42.3161667,-122.0266667,8.56,5.9,md,201,129,,0.09,uw,uw10313718,2018-01-16T17:56:02.876Z,"Oregon",earthquake,0.441,0.93,0.02,3,reviewed,uw,uw 1993-03-25T13:34:35.440Z,45.0351667,-122.6065,19.608,5.6,md,40,67,0.2067,0.39,uw,uw10306313,2017-05-10T20:02:37.829Z,"Oregon",earthquake,0.934,2.84,,2,reviewed,uw,uw 1980-05-18T15:32:11.430Z,46.2073333,-122.188,1.51,5.7,md,18,62,0.008296,0.22,uw,uw10084803,2018-01-25T18:47:04.065Z,"Mount St. Helens area, Washington",earthquake,0.682,0.56,0.08,1,reviewed,uw,uw 1980-04-22T19:28:18.710Z,46.2026667,-122.182,-0.523,5,md,21,57,0.03886,0.18,uw,uw10084513,2016-07-24T21:26:43.420Z,"Mount St. Helens area, Washington",earthquake,0.439,0.62,0.07,1,reviewed,uw,uw 1980-04-18T21:16:02.120Z,46.208,-122.1825,-0.223,5,md,22,59,0.04013,0.19,uw,uw10082403,2016-07-24T21:26:24.290Z,"Mount St. Helens area, Washington",earthquake,0.46,0.73,0.09,1,reviewed,uw,uw 1980-04-14T13:49:03.760Z,46.2035,-122.1973333,0.515,5.2,md,21,55,0.0289,0.19,uw,uw10080808,2016-07-24T21:26:05.760Z,"Mount St. Helens area, Washington",earthquake,0.042,0.05,0.13,1,reviewed,uw,uw 1980-04-08T19:29:02.910Z,46.2098333,-122.1958333,-0.561,5.1,md,19,63,0.03255,0.39,uw,uw10077923,2016-07-24T21:25:35.450Z,"Mount St. Helens area, Washington",earthquake,1.374,1.85,0.07,1,reviewed,uw,uw 1976-12-19T19:00:59.500Z,42.752,-125.603,15,5.4,mb,,,,,us,usp0000kuc,2014-11-06T23:21:38.664Z,"offshore Oregon",earthquake,,,,,reviewed,us,us 1975-07-29T01:48:16.200Z,43.687,-126.103,33,5.2,mb,,,,,us,usp0000c7e,2014-11-06T23:21:31.509Z,"off the coast of Oregon",earthquake,,,,,reviewed,us,us 1973-06-16T14:43:47.500Z,44.98,-125.774,33,5.6,mb,,,,,us,usp000026k,2015-05-13T18:53:08.000Z,"off the coast of Oregon",earthquake,,,,,reviewed,us,us
equakes25f.txt
time,latitude,longitude,depth,mag,magType,nst,gap,dmin,rms,net,id,updated,place,type,horizontalError,depthError,magError,magNst,status,locationSource,magSource 2018-10-01T00:31:15.910Z,42.0223333,-124.2715,16.22,2.99,ml,12,173,0.05672,0.27,uw,uw61424827,2018-10-02T03:31:16.224Z,"3km SSE of Brookings, Oregon",earthquake,1.11,0.96,0.204,10,reviewed,uw,uw 2018-09-25T13:18:50.110Z,45.6766667,-122.8965,22.71,2.56,ml,43,38,0.1266,0.11,uw,uw61422757,2018-10-01T21:06:21.040Z,"8km S of Scappoose, Oregon",earthquake,0.18,0.41,0.101,23,reviewed,uw,uw 2018-09-17T00:08:26.690Z,45.1358333,-122.9721667,40.89,2.83,ml,45,30,0.1447,0.24,uw,uw61419862,2018-10-08T02:55:33.040Z,"6km WNW of Gervais, Oregon",earthquake,0.31,0.38,0.183,25,reviewed,uw,uw 2018-09-08T14:17:50.380Z,42.2935,-124.6693333,21.05,2.76,ml,9,264,0.2409,0.12,uw,uw61426681,2018-10-06T19:01:11.040Z,"23km WSW of Gold Beach, Oregon",earthquake,0.88,0.33,0.265,9,reviewed,uw,uw 2018-08-30T23:38:18.160Z,44.8213333,-122.2221667,3.78,2.57,ml,4,255,1.001,0.08,uw,uw61424371,2018-11-02T14:37:24.040Z,"21km ENE of Mill City, Oregon",earthquake,10.57,31.61,0.376,2,reviewed,uw,uw 2018-08-24T10:48:50.260Z,44.228,-125.4033,10,2.8,ml,,177,1.087,0.96,us,us1000ggsm,2018-11-01T17:50:57.040Z,"107km WNW of Florence, Oregon",earthquake,4.9,2,0.039,86,reviewed,us,us 2018-07-25T21:06:21.610Z,44.4363,-126.0199,10,2.9,ml,,215,1.576,1.32,us,us2000gdvn,2018-10-09T16:43:29.040Z,"155km W of Waldport, Oregon",earthquake,7.1,2,0.046,62,reviewed,us,us 2018-07-11T12:09:45.720Z,44.613,-124.3475,25.41,3,ml,17,229,0.2154,0.08,uw,uw61395262,2018-10-02T16:40:34.040Z,"23km W of Newport, Oregon",earthquake,0.41,0.53,0.242,12,reviewed,uw,uw 2018-06-07T15:55:26.410Z,43.7227,-125.5501,10,2.6,ml,,227,0.893,0.84,us,us1000ekk8,2018-08-29T16:35:14.040Z,"108km WNW of Barview, Oregon",earthquake,4.5,2,0.045,66,reviewed,us,us 2018-05-28T02:08:28.730Z,46.0455,-122.1278333,12.12,2.97,ml,48,35,0.06258,0.15,uw,uw61379272,2018-08-28T16:27:27.040Z,"28km ENE of Amboy, Washington",earthquake,0.24,0.63,0.172,26,reviewed,uw,uw 2018-04-26T08:44:33.720Z,43.7127,-125.5646,10,3.8,mb,,175,1.004,0.91,us,us1000dsjw,2018-07-25T21:36:57.040Z,"108km WNW of Barview, Oregon",earthquake,4.1,2,0.141,13,reviewed,us,us 2018-04-15T03:45:41.250Z,44.9455,-122.7558333,18.38,3.08,ml,36,41,0.1508,0.23,uw,uw61363027,2018-07-07T02:25:23.040Z,"6km SSE of Silverton, Oregon",earthquake,0.33,1.1,0.172,24,reviewed,uw,uw 2018-03-09T08:48:28.940Z,44.2056,-124.6819,10,2.8,ml,,148,0.434,1.28,us,us1000d3fy,2018-06-08T05:12:16.040Z,"52km WNW of Florence, Oregon",earthquake,2.1,2,0.056,42,reviewed,us,us 2018-03-06T19:42:46.760Z,44.476,-120.2706667,-1.19,2.69,ml,9,311,0.4931,0.3,uw,uw61371261,2018-06-08T05:12:12.040Z,"11km SW of Prineville, Oregon",explosion,5.07,31.61,0.052,5,reviewed,uw,uw 2018-02-04T22:37:32.230Z,45.1558333,-124.3488333,30.15,3.06,ml,21,155,0.3731,0.41,uw,uw61366781,2018-04-25T01:39:48.040Z,"30km W of Pacific City, Oregon",earthquake,0.83,2.68,0.117,6,reviewed,uw,uw 2017-12-14T01:24:26.830Z,45.0353333,-122.5993333,17.37,3.96,ml,53,40,0.1769,0.19,uw,uw61335997,2018-10-28T05:34:08.405Z,"12km S of Molalla, Oregon",earthquake,0.22,0.82,0.21,28,reviewed,uw,uw 2017-12-06T23:16:01.720Z,43.9998,-124.8511,20.99,2.8,ml,,157,0.535,0.59,us,us2000c1e4,2018-02-28T02:32:30.040Z,"60km W of Florence, Oregon",earthquake,3.2,4.5,0.047,60,reviewed,us,us 2017-11-11T07:41:04.640Z,42.1398333,-121.6926667,6.88,2.58,ml,10,125,0.1036,0.08,uw,uw61327512,2018-01-31T03:04:48.040Z,"8km SSE of Altamont, Oregon",earthquake,0.31,0.42,0.114,8,reviewed,uw,uw 2017-10-16T05:28:09.430Z,45.9166667,-123.4611667,24.31,3.38,ml,43,46,0.06977,0.22,uw,uw61342436,2018-01-11T02:22:03.040Z,"21km WNW of Vernonia, Oregon",earthquake,0.3,0.82,0.201,26,reviewed,uw,uw 2017-10-10T02:45:37.050Z,45.3206667,-121.6861667,6.84,2.75,ml,25,45,0.02276,0.16,uw,uw61339596,2018-01-09T02:14:53.040Z,"23km E of Mount Hood Village, Oregon",earthquake,0.24,0.23,0.188,23,reviewed,uw,uw 2017-09-26T22:21:58.680Z,44.2518333,-123.9531667,24.14,2.77,ml,20,98,0.1208,0.28,uw,uw61311237,2017-12-22T21:56:23.040Z,"21km SSE of Waldport, Oregon",earthquake,0.45,1.16,0.136,14,reviewed,uw,uw 2017-09-05T15:48:09.530Z,44.3418333,-124.4218333,25.72,2.82,ml,13,248,0.235,0.21,uw,uw61303577,2017-12-07T20:00:13.040Z,"24km WSW of Waldport, Oregon",earthquake,1.56,0.96,0.285,7,reviewed,uw,uw 2017-08-26T20:44:09.900Z,44.6125,-124.3365,25.73,2.54,ml,19,120,0.2076,0.1,uw,uw61299252,2017-11-22T03:43:34.040Z,"22km W of Newport, Oregon",earthquake,0.3,0.53,0.125,10,reviewed,uw,uw 2017-07-11T23:40:24.420Z,44.3798333,-122.4308333,12.4,2.52,ml,22,80,0.1137,0.55,uw,uw61281277,2017-10-06T23:56:15.040Z,"24km E of Sweet Home, Oregon",earthquake,0.84,4.32,0.275,15,reviewed,uw,uw 2017-07-09T00:27:13.900Z,46.1635,-123.1088333,23.09,2.51,ml,29,70,0.05635,0.17,uw,uw61280487,2017-10-06T23:56:10.040Z,"8km W of West Longview, Washington",earthquake,0.37,0.56,0.113,24,reviewed,uw,uw
Step by Step Solution
There are 3 Steps involved in it
Step: 1
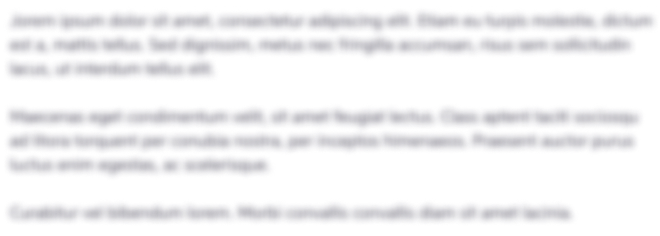
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started