Answered step by step
Verified Expert Solution
Question
1 Approved Answer
you will write a program that can represent TV shows and movies. You can think of it as a very simple version of imdb.com. You
you will write a program that can represent TV shows and movies. You can think of it as a very simple version of imdb.com. You will use inheritance and have a superclass called Production and two subclasses, TvShow and Movie.
I have provided the tester code you can use to test your program once you are finished. Make sure to name all classes and methods EXACTLY as you see here, otherwise the tester code will not work with your code. Points will be taken off if you use different classmethod names than what is specified. You can verify that you have named everything correctly by running the tester code.
Production class points
Create a class called Production. It should have:
instance variables all of them should be private:
title String
genre String
year int
numStars int
numVotes int
actors ArrayList of Strings
constructor
It should have parameters for the title, genre, and year instance variables in that order and set the values of those instance variables to their corresponding parameters
It should also initialize numStars and numVotes to and actors to a new ArrayList.
You can also do this part in the instance variable section. Either way is fine.
Getter methods getTitle, getGenre, and getYear
You do not need any other gettersetter methods
addActor method
one String parameter the name of an actor
no return value
the method should add the actor's name the parameter to the actors ArrayList
acceptVote method
one int parameter the number of stars somebody voted for it
no return value
the method should add the number of stars the parameter to the numStars instance variable
it should also increment the numVotes instance variable
computeScore method
no parameters
returns a double value
if there are no votes yet, return
otherwise, compute and return the score numStars divided by numVotes
Hint: you will need to use casting here to avoid the Java division quirk
toString method
Write the toString method so that when a Production object is printed, the following are displayed on separate lines you can use the
escape characters for the separate lines:
title
genre
year
actors
the score from the computeScore method
For example, it might look like this:
The Lion King
drama
Matthew Broderick, Jonathan Taylor Thomas, James Earl Jones, Whoopi Goldberg
TvShow class points
Create a class called TvShow. It should be a subclass of Production and have the following:
instance variables both private
network String
numSeasons int
constructor
It should have parameters for title, genre, year, network, and numSeasons in that order and set all the instance variables to their corresponding parameters.
Getter methods getNetwork and getNumSeasons
addSeason method:
no parameters
no return value
the method should increase the number of seasons by
recommendShow method:
one TvShow parameter the TvShow you will compare the current show with
no return value
It should compare the scores of the current show with the other show the parameter that was passed in and, whichever show has the higher score, it should print a message saying "You should watch" followed by the title of the show.
For example, it might say: You should watch Seinfeld
Movie class points
Create a class called Movie. It should be a subclass of Production and have the following:
instance variables both private:
rating String
this is the rating of the movie, which will either be GPGPG or R
runtime int
this is how long the movie is in minutes
constructor
it should have parameters for title, genre, year, rating, and runtime in that order and set all the instance variables to their corresponding parameters
Getter methods getRating and getRuntime
isOldEnoughToWatch method
one int parameter the age of the person who wants to watch the movie
boolean return value
the method should return true or false based on whether the person is old enough to watch the movie, considering their age and the rating of the movie:
anyone can watch a G or PG movie regardless of how old they are
a person must be at least to watch a PG movie
a person must be at least to watch an R movie
Step by Step Solution
There are 3 Steps involved in it
Step: 1
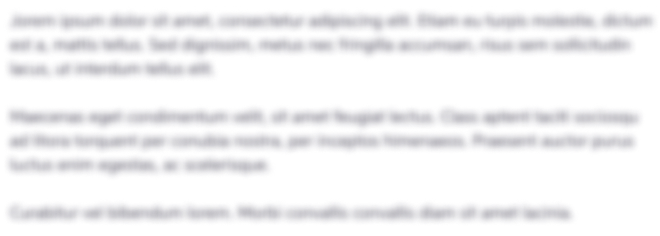
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started