Question
You will write a program that involve Python concepts like classes, decorators, gen- erators and iterators. You will be writing an RSA encryption/decryption system that
You will write a program that involve Python concepts like classes, decorators, gen- erators and iterators. You will be writing an RSA encryption/decryption system that conforms to the following specifications.
Write a class called RSA that will contain all of the functions in the program. In the constructor forRSA initialize an empty list and set variables e and d to 0.
Write a function called inputFunc that reads in the number of entries from the user. Then, read in those many values and add them to the list.
Write a function called printFunc that takes in a number and prints message is followed by the number.
Write a generator function called primeGen that will take a minimum value as a parameter and then yield the next prime numbers. Please note that this input parameter will be quite large and you might want to use long if youre using Python 2.
Write a function called keyGen. This function will read in a minimum value from the user. Then, it will use the primeGen generator to get the next 2 prime numbers and generate the value N and the keys e and d. Print e and N but not the other values.
You would probably also need to write helper functions for the Lowest Common Multiple (LCM), Greatest Common Divisor (GCD) and the Totient function.
Write a function called encrypt that takes in a number as a parameter and returns the RSA encrypted value of the number.
Write a function called decrypt that takes in an encrypted number as a parameter and returns the RSA decrypted value.
Write a decorator function for printFunc that will print The encrypted before the printed message.
Write another decorator function for printFunc that will print The decrypted before the printed message.
Write a function called messages that calls inputFunc and keyGen and then, uses an iterator to iterate through the list and encrypts each of the numbers using the encrypt function. Store the results in another list. Then, go through the second list and print each encrypted number using the decorator for the encrypted message.
Verify your results be decrypting each of the encrypted messages and checking if you get the old value back. Print the decrypted values using the decorator for the decrypted message.
In main, create an RSA object and call the messages function.
This section shows the sample output for the program. Please note that the exceptions raised when the iterator hits the end of the list is not included here, since I checked for the exception while generating the output. Please make sure you do so as well. Also, you might have different values even if you have the same inputs, depending on how e is chosen. As long your decrypted message matched your initial input, you should be fine.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
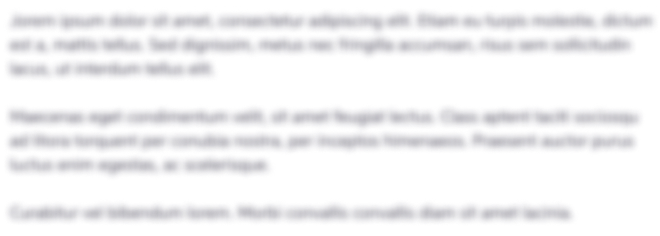
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started