Question
You work for a concert promoter. Since some of the patrons of past events have been complaining about the moshers in the mosh pit the
You work for a concert promoter. Since some of the patrons of past events have been complaining about the moshers in the mosh pit the promoter has given you the job to determine the maximum number of moshers in the mosh pit so that a reserved area can be created for the moshers. The number of moshers that can fit into a mosh pit depends on the pit size, the average size of a mosher, the separation between the moshers, and the north, south, east, and west thickness of the mosh pit barricade to contain the moshers. Your program will need to get the following input from the user:
The length and width, in feet, of the mosh pit. Assume these are the maximum (outside) dimensions of the barricade.
The thickness of the north, south, east, and west sides of the barricade
The separation between moshers (and a barricade side if applicable)1
The average size of the mosher2
1 To get the space between moshers prompt the user for a separation value:
A separation of A or a means 2.5 foot distance between moshers
A separation of B or b means 1.5 foot distance between moshers
A separation of C or c means 0.5 foot distance between moshers
A separation of anything else means exit the program.
NOTE: Use a char variable type for the prompt response. See http://www.cplusplus.com/reference/cctype/tolower/ (Links to an external site.)Links to an external site.
2 To get the average size of a mosher (assume all moshers in the mosh pit will be the same size):
A mosher size of L or l means each mosher takes up a 1.5 foot by 1.5 foot space
A mosher size of XL or xl means each mosher takes up a 2.5 foot by 2.5 foot space
A mosher size of XXL or xxl means each mosher takes up a 3.5 foot by 3.5 foot space
A mosher size of anything else means exit program.
NOTE: Use a string data type for the prompt response. See http://stackoverflow.com/questions/313970/stl-string-to-lower-case (Links to an external site.)Links to an external site.
To ease your calculations assume that the moshers (depicted below) are initially arranged in rows and columns in a grid like fashion and that the distance between an end mosher and the barricade is the separation distance.
Also even though the moshers are not square assume they take up a square space.
Finally the program should run in a continuous loop (unless users press a key to exit See executable example program MoshPit.zip).
See http://support.microsoft.com/kb/99261 (Links to an external site.)Links to an external site. on how to clear a DOS screen if needed.
See http://www.cplusplus.com/reference/cmath/round/ (Links to an external site.)Links to an external site. on how to use floor() to round down (since fractional numbers for final counts of moshers doesnt make sense).
Example calculation:
So as an example lets calculate the number of moshers that would fit east to west in a mosh pit. Suppose the user entered a separation of "A" (2.5 feet) and a mosher size of "L" (1.5 feet). The space one mosher would occupy (east to west) would be 1.5 feet plus 2.5 feet of separation (1.25 feet on each side of the mosher), or a total of 4 feet. Also assume the user entered east-west barricade walls of a thickness of 1 foot each (2 feet total). Finally assume the user entered a required east-west width of 18 feet. Now the calculations. The available space for moshers is: 18 feet - 2 feet = 16 feet. Each mosher needs 4 feet of space. Therefore the total number of moshers that will fit in the pit will be 16 feet /4 feet = 4 moshers.
The MoshPit Program:
The MoshPit program must be implemented as follows. A MoshPit class and a main function defined as follows:
MoshPit::MoshPit() - A constructor that initializes all of its data members.
void MoshPit::GetMoshPitDimensions() - Member function that prompts the user for the dimensions of the mosh pit and store the results in the class' data members. Notice the function signature takes no arguments and returns no values.
void MoshPit::GetMosherSeperation() - Member function that prompts the user for the "mosher seperation" and store the results in the class' data member(s). Notice the function signature takes no arguments and returns no values.
void MoshPit::GetMosherSize() - Member function that prompts the user for the "size of the mosher" and store the results in the class' data member(s). Notice the function signature takes no arguments and returns no values.
void MoshPit::CalculateMoshPit() - Member function that uses the classes private data members to perform the mosh pit calculations. Notice the function signature takes no arguments and returns no values.
void MoshPit::DisplayResults() - Member function that uses the classes private data members to display the results to the user. Notice the function signature takes no arguments and returns no values.
int MoshPit::QuitProgram() - Member function that is called when ever the program needs to terminate. Notice the function signature takes no arguments and returns an integer value.
int Main() - Non-member function used to instantiate the MoshPit class and call it's member functions.
Do not modify the function signatures and do not use global variables. Use member variables instead.
A screen shot of the program follows.
Here is MoshPit_Shell.cpp to start with.
////////////////////////////////////////////////////////////////////////
/////
// MoshPit.cpp : This is one shell of a program.
//////////////////////////////////////////////////////////////////////////
/////
#include "stdafx.h"
#include
#include
#include
#include
using namespace std;
//////////////////////////////////////////////////////////////////////////
/////
//
//////////////////////////////////////////////////////////////////////////
/////
class MoshPit
{
public:
MoshPit(); //Constructor
void GetMoshPitDimensions();
void GetMosherSeperation();
void GetMosherSize();
void CalculateMoshPit();
void DisplayResults();
int QuitProgram();
private:
// The class' data members go here....
};
//////////////////////////////////////////////////////////////////////////
/////
//
//////////////////////////////////////////////////////////////////////////
/////
MoshPit::MoshPit()
{
// Initialize the class' data members here....
}
//////////////////////////////////////////////////////////////////////////
/////
//
//////////////////////////////////////////////////////////////////////////
/////
void MoshPit::GetMosherSeperation()
{
// Member function that prompts the user for the "mosher seperation"
and
// store the results in the class' data member(s).
}
//////////////////////////////////////////////////////////////////////////
/////
//
//////////////////////////////////////////////////////////////////////////
/////
void MoshPit::GetMosherSize()
{
// Member function that prompts the user for the "size of the
mosher" and
// store the results in the class' data member(s).
}
//////////////////////////////////////////////////////////////////////////
/////
//
//////////////////////////////////////////////////////////////////////////
/////
void MoshPit::CalculateMoshPit()
{
// Member function that uses the classes private data members to
perform
// the mosh pit calculations.
}
//////////////////////////////////////////////////////////////////////////
/////
//
//////////////////////////////////////////////////////////////////////////
/////
int MoshPit::QuitProgram()
{
cout
system("pause");
exit(1);
}
//////////////////////////////////////////////////////////////////////////
/////
//
//////////////////////////////////////////////////////////////////////////
/////
void MoshPit::GetMoshPitDimensions()
{
system("cls");
cout
********************"
//// Prompt user for length of mosh pit
cout
////
cout
////
//// Prompt the user for the thickness of the barricade.
cout
North side of the barricade: ";
////
cout
South side of the barricade: ";
////
cout
side of the barricade: ";
////
cout
side of the barricade: ";
////
cout
}
//////////////////////////////////////////////////////////////////////////
/////
//
//////////////////////////////////////////////////////////////////////////
/////
void MoshPit::DisplayResults()
{
// Member function that uses the classes private data members to
display the results to the user
system("pause");
}
//////////////////////////////////////////////////////////////////////////
/////
//
//////////////////////////////////////////////////////////////////////////
/////
int main()
{
// Create an instance of the class.
MoshPit thePit;
// Notice that there is no exiting the loop here. Exiting the
program
// happens in "QuitProgram()"
while (true)
{
thePit.GetMoshPitDimensions();
thePit.GetMosherSeperation();
thePit.GetMosherSize();
thePit.CalculateMoshPit();
thePit.DisplayResults();
};
return 0;
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
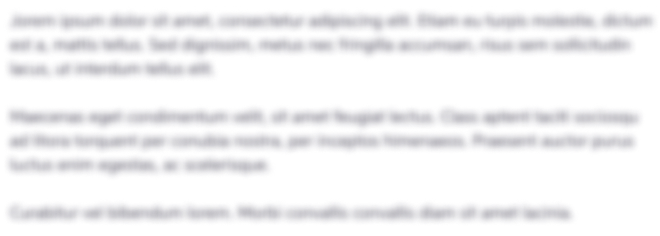
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started