Question
Your assignment is to implement a class called Employee (there is no main method). An Employee has a name (a String), a salary (a double)
Your assignment is to implement a class called Employee (there is no main method). An Employee has a name (a String), a salary (a double) and number of years of experience. (an integer). In addition, your class Employee must have a static instance variable to keep track of number of employees. The class Employee must include the following constructors and methods.
Method header | Method Description |
public Employee( ) | Default constructor sets the name to "???, the salary to 0.0 and years of experience to 0 |
public Employee (String name, double salary, int years) | Constructs an Employee given the name as a String, salary as double and years of experience as an integer |
public Employee (String str) | Constructs an Employee given a string and using methods in class String or Scanner to extract name, salary: years of experiences. For Example the string "Bond:70000:20" |
public String getName( ) | Returns the name of the employee |
public double getSalary( ) | Returns the employee's salary. |
public int getYears() | Returns the employees years of experience |
public String setName(String st) | This is a mutator method that can be used to change the name of employee |
public void setYears() | A method to be run annually; increments the years of experiences annually |
public void setYears(int numYears) | This is a mutator method that can be used to change the number of years of experience. |
public void raiseSalary (double byPercent) | Takes one parameter and raises the employee's salary by that percent |
public Employee moreExperience(Employee e) | Compares the two employees number of years of experience and returns the Employee who is more experienced |
public static int getNumEmployees() | Returns the number of employees |
public String toString( ) | Returns a String with information on each Employee (etc. name, salary, and years of experiences). The salary needs to be formatted with currency format. |
Save the Employee class in a file called Employee.java and use the test driver called Assignment6.java, which has the main method to create new Employee objects and to test your class. You need to complete this class. A sample output is shown below.
Sample output: User input is in RED
Enter name of the employee? Grace Hopper Enter salary for Grace Hopper? 89000 Enter number of years for Grace Hopper? 8
Command Options
----------------------------------- 'a': prints info 'b': raise salary 'c': increment the years of experience 'd': who has more experience
'e': number of employees 'f': years of experience '?': Displays the help menu 'q': quits
Please enter a command or type ? a
Name: Grace Hopper Salary: $89,000.00 Years of Experience: 8
Name: Bond Salary: $70,000.00 Years of Experience: 20
Please enter a command or type ? b Enter the amount of raise: 2
Name: Grace Hopper Salary: $90,780.00 Years of Experience: 8
Name: Bond Salary: $71,400.00 Years of Experience: 20
Please enter a command or type ? c Year has been added.
Please enter a command or type ? a
Name: Grace Hopper Salary: $90,780.00 Years of Experience: 9
Name: Bond Salary: $71,400.00 Years of Experience: 21
Please enter a command or type ? d Bond has more experience.
Please enter a command or type ? e They are 2 employees.
Please enter a command or type ? f Enter years of experience: 35
Please enter a command or type ? a Name: Grace Hopper
Salary: $90,780.00 Years of Experience: 35
Name: Bond Salary: $71,400.00 Years of Experience: 21
Please enter a command or type ? d Grace Hopper has more experience.
Please enter a command or type ? q
Test Driver:
import java.util.*; import java.text.*; public class Assignment6 { public static void main(String[] args) { Scanner console = new Scanner(System.in); String choice; char command; // Create a new Employee object System.out.print("Enter name of the employee? "); String name = console.nextLine(); System.out.print("Enter salary for " + name + "? "); double salary = console.nextDouble(); System.out.print("Enter number of years for " + name + "? "); int years = console.nextInt(); //use the constructors in class Employee Employee e1 = new Employee(name, salary, years); Employee e2 = new Employee("Bond:70000:20"); // print the menu printMenu(); do { // ask a user to choose a command System.out.print(" Please enter a command or type ?"); choice = console.next().toLowerCase(); command = choice.charAt(0); switch (command) { case 'a': // print the info for each employee // complete the code here break; case 'b': //raise employee's salary System.out.print("Enter the amount of raise: "); double raise = console.nextDouble(); //complete the code here break; case 'c': //increment the number of years by 1 e1.setYears(); e2.setYears(); System.out.println("Year has been added."); break; case 'd': //find who has more experience Employee who = e1.moreExperience(e2); System.out.print(who.getName() + " has more experience."); break; case 'e': //number of employees //complete the code here break; case 'f': //years of experience System.out.print("Enter years of experience: "); years = console.nextInt(); e1.setYears(years); break; case '?': printMenu(); break; case 'q': break; default: System.out.println("Invalid input"); } } while (command != 'q'); }// end main public static void printMenu() { System.out.print(" Command Options " + "----------------------------------- " + "'a': prints info " + "'b': raise salary " + "'c': increment the years of experience " + "'d': who has more experience " + "'e': number of employees " + "'f': years of experience " + "'?': Displays the help menu " + "'q': quits "); } // end of the printMenu method }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
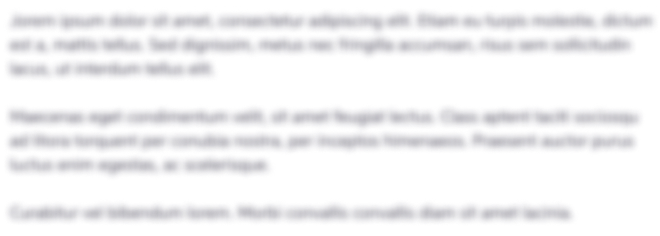
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started