Question
Your professor needs your help to decipher some secret message in a sentence. The secret message is formed by taking the first letter of each
Your professor needs your help to decipher some secret message in a sentence. The secret message is formed by taking the first letter of each word in the sentence, in the order of their appearance. Write the following EXACT function:
/** * FUNCTION SIGNATURE: string getSecretMessage(string str) * PURPOSE: get secret message by taking the first character from each word in the input sentence, in the order they appear * PARAMETER: * str, the input sentence * RETURN VALUE: * The secret message */
Examples:
Input str: "professor can code"
Secret Message: "pcc"
(Note: input consists of 3 words separated by single space. Taking the first character of each word gets you "pcc")
Input str: "professor can code"
Secret Message: "pcc"
(Note: input consists of 3 words separated by multiple spaces. Taking the first character of each word gets you "pcc")
Input str: " "
Secret Message: ""
(Note: input is " " - there are no words there. The secret message is "" since there are no words in the input sentence)
Constraints / Assumptions:
For this problem, you may use ANY C/C++ libraries
Your input sentence is a collection of words consisting of only lowercase English characters ('a' - 'z') and spaces (' ').
There may be multiple spaces between words.
A sentence may consist of only spaces
If there is no secret message to be generated, the function returns ""
Failure to follow the same exact function signature receives -1 logic point deduction
A sentence will contain between 1 and 50 characters
Your main() function isn't graded; only the above-referenced function is
Failure to follow the same exact function signature receives -1 logic point deduction
You can test your function in the following fashion, for example:
int main() { cout << getSecretMessage("professor can code") << endl; // prints "pcc" cout << getSecretMessage(" ") << endl; // prints nothing cout << getSecretMessage("pasadena") << endl; // prints "p" ... return 0; }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
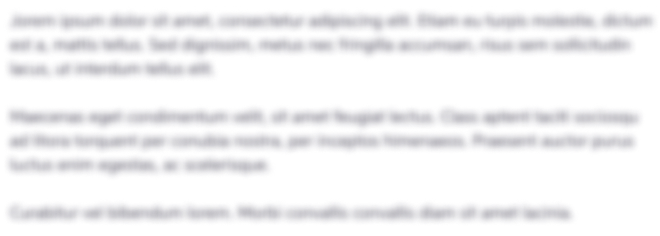
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started