Question
Your task is to take the race track program presented in class and add several parts to it. This is a demonstration of the techniques
Your task is to take the race track program presented in class and add several parts to it. This is a demonstration of the techniques from chapter 3 and chapter 6. You will be creating a class based on the examples given, as well as modifying their access levels (chapter 3). You will also work with the relationship between car object and the engine object (chapter 6). Creating new objects - Add a new class called Motorcycle. It should extend vehicle which will allow it to be a part of the array with other vehicles. Motorcycles have great speed (the horsepower you should set for your motorcycles engine should be 95) but are dangerous and will have a chance to crash. The vehicle class has a blank (abstract) Go() method. To extend Vehicle you must give a definition for Go. Do so like Car, and Truck but add a conditional statement that checks for the motorcycles chance to crash which is 2% per loop do this by checking a random number (math.random() < .02f) and creating another field in motorcycle called hasCrashed to store the result. If the motorcycle crashes then it should not progress in the race any further. The motorcycle is allowed to participate in future races in the season however (so hasCrashed should be reset for each new race). There should be 25 races in a season. Add a loop to make 25 races happen. At the end of the season output the win totals of each vehicle as well as the vehicle with the most wins. Encapsulation and access modifiers (ownership of data) - Next we need to redefine the way passengers are kept track of in all vehicles. First passengers, Vin, and RaceProgress should be a private variable which can only be accessed by appropriate accessor and mutator methods (which you will have to add). VIN should be made a final variable since there is never any reason to change it (hint do we need a mutator method for this field?). Finally, we should add a new final variable for the maxOccupancy of the vehicle (do not add it to the subclasses). The max occupancy should be checked in the mutator method for passengers. The max occupancy should be defined as:
o Car = 5 o Truck = 3 o Motorcycle = 1 If more passengers than are allowed attempt to pile into a vehicle the program should indicated this to the user and continue with the max passenger amount. This can be accomplished by using the super call in the subclasss (car, truck, motorcycle) constructors to pass in their specific value. There are so rules here that I am forcing you to learn when it comes to creating final variables (specifically that the maxOccupancy must be set by the working constructor of Vehicle). If you run into problems, first try to make simple examples to try and learn these basics, and if you continue to run into errors reach out to your instructor for help. Note: you do not need to make fields/variables in other classes private (like engine for example). I am only going to look at Vehicle when grading this step and figure if you can do it correctly for one class then you should be able to do it for others. Aggregation vs Composition - Currently the engine variable is an aggregation relationship to the vehicle class your job is to convert the relationship to a composition one. You have 2 options to complete this that you will demonstrate: With the car class create copy of the engine within the constructor (you may write a copy constructor for the Engine class to accomplish this) and store that appropriately. The vehicle class already takes an Engine into its constructor. You will need to modify this so that it creates a deep copy (again, using the copy constructor) and stores that instead of the direct reference to the passed in Engine. With the truck class change the constructor so that an int for the horsepower is passed into the truck constructor instead of the entire engine (t his is the only data needed to create an engine) use this to create the engine within the vehicle (truck can pass the int up into vehicle if you add a constructor for this in vehicle). Since truck/Vehicle creates its own engine within the object it is safer code than creating it in main or who-knows-where. The motorcycle can be left however you choose and doesnt have to have its Engine modified.
/** * This is the program driver (where the program starts) * It is in charge of creating the race and it's participants and telling them to "go" in the race. * @author vjl8401 */ public class RaceTrack //driver { public final static int raceDuration = 1000; //store the length of the race (can be accessed anywhere in code) public static void main(String arg[]) { //Instantiating my object instances Engine engine = new Engine(67); Vehicle c1 = new Car(1,engine,2); //this one I'm storing as the base class (vehicle) Car c2 = new Car(2,new Engine(94),4); //this one I store as the sub class (car). There are differences but they do not come into play here Truck t1 = new Truck(3,new Engine(87),2,250); //This is an array Vehicle[] allVehicles = new Vehicle[3]; //placing the vehicles into an array allVehicles[0] = c1; //polymorphism ("isa") allVehicles[1] = c2; //remember just because I store these as vehicles doesn't mean that the allVehicles[2] = t1; //methods for them has changed. Each still stores its own go method. //infinite loop (well without the base case it is) while (true) //this will run until a race participant crosses the finish line (passes raceDuration) { int max=0; //tell the cars to "go" one by one for (int i=0; i
Step by Step Solution
There are 3 Steps involved in it
Step: 1
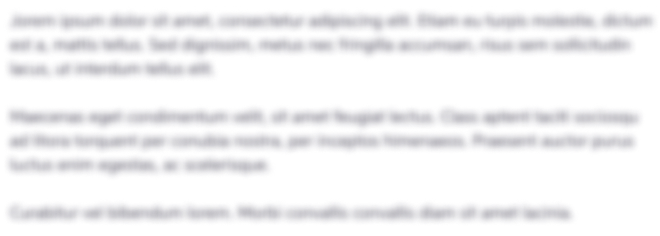
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started