Question
7) The code for the add method for the ArrayBag class is shown below. What is the missing code? def add(self, item): self.items[len(self)] = item
7)
The code for the add method for the ArrayBag class is shown below. What is the missing code?
def add(self, item):
self.items[len(self)] = item
0.37 Points
-
self.items +=1
-
self.len = self.items
-
self = self + 1
-
self.size += 1
8)
The code for the __iter__ method is shown below. What is the missing code?
def __iter__(self):
while cursor < len(self):
yield self.items[cursor]
cursor += 1
0.37 Points
-
cursor = len(self)
-
cursor = self.size
-
cursor = 1
-
cursor = 0
9)
What method does Python run when it sees the in operator used with a collection?
0.37 Points
-
_iter_
-
_eq_
-
_add_
-
_contains_
10)
The __eq__ method in the AbstractCollection class compares pairs of items in two collections.
0.37 Points
-
True
-
False
11)
A primary purpose of using inheritance is to eliminate redundant code.
0.37 Points
-
True
-
False
12)
Programming languages such as Java include a collection framework more extensive than that of Python.
0.37 Points
-
True
-
False
13)
When a programmer calls the iter function on a collection, the collection's iterator object is returned.
0.37 Points
-
True
-
False
14)
If a programmer calls the next function on an iterator object and there is no current item in the sequence, the next function returns the Null item.
0.37 Points
-
True
-
False
15)
In the case of the AbstractCollection class, which of the following methods should NOT be included in this class?
0.38 Points
-
count
-
add
-
isEmpty
-
_len_
16)
When creating the AbstractCollection class, which methods must you modify to provide default behavior?
0.38 Points
-
_init_ and _len_
-
isEmpty and add
-
_len_ and count
-
_str_ and _eq_
17)
When a programmer calls the next function on an iterator object, what happens if there is no current item in the sequence?
0.38 Points
-
the None item is returned
-
the compiler generates an error
-
a StopIteration exception is raised
-
the previous item is returned
18)
In the following code for the ArrayBag class __contains__ method, what is the missing code?
def __contains__(self, item):
left = 0
right = len(self) - 1
while left <= right:
midPoint = (left + right) // 2
if self.items[midPoint] == item:
return True
elif self.items[midPoint] > item:
right = midPoint - 1
else:
return False
0.38 Points
-
right = midPoint + 1
-
left = midPoint + 1
-
right = left + 1
-
left = midPoint - 1
19)
In the following code for the __add__ method in the ArraySortedBag class, what is the missing code?
def __add__(self, other):
result = ArraySortedBag(self)
for item in other:
return result
0.38 Points
-
result.add(item)
-
add.item(result)
-
result = result + 1
-
item.add(result)
20)
Access to a stack is restricted to the bottom end.
0.38 Points
-
True
-
False
21)
Stacks adhere to a LIFO protocol.
0.38 Points
-
True
-
False
22)
The operation for removing an item from the stack is called push.
0.38 Points
-
True
-
False
23)
With a stack, you always access the item that has been most recently added.
0.38 Points
-
True
-
False
24)
The operator in a postfix expression follows the operands.
0.38 Points
-
True
-
False
25)
The operator in an infix expression follows the operands.
0.38 Points
-
True
-
False
26)
A stack structure is a built-in part of the Python language.
0.38 Points
-
True
-
False
27)
A stack structure is a built-in part of the Python language.
0.38 Points
-
True
-
False
28)
When using a stack to evaluate the balance of brackets and parentheses in an expression, what is the final step?
0.38 Points
-
at the end of the expression, if a final closing bracket is found, the brackets balance
-
at the end of the expression, if the stack is full, the brackets balance
-
at the end of the expression, if the stack is empty, the brackets do not balance
-
at the end of the expression, if the stack is empty, the brackets balance
29)
What is the function of the peek method in a stack implementation?
0.38 Points
-
to return the top item and remove it from the stack
-
to return the top item without removing it
-
to return the bottom item without removing it
-
to return the bottom item and remove it from the stack
30)
In the algorithm to evaluate a postfix expression, what does the algorithm do when an operator is encountered?
0.38 Points
-
pop the resulting value from the stack
-
push the preceding operands onto the stack
-
push the operator onto the stack
-
apply the operator to the two preceding operands
31)
If the portion of the postfix expression scanned so far is 7 6 2 +, what is the result when the operator is applied to the operands?
0.38 Points
-
13
-
9
-
8
-
15
32)
What is the resulting postfix expression from the following infix expression? (12 + 5) * 2 - 3
0.38 Points
-
12 5 2 + * 3 -
-
12 5 2 + 3 - *
-
12 5 + 2 3 * -
-
12 5 + 2 * 3 -
33)
Which of the following begins in a predefined starting state and then moves from state to state in search of a desired ending state?
0.38 Points
-
memory management algorithm
-
postfix to infix converter
-
infix to postfix converter
-
backtracking algorithm
34)
In the following code that defines the push method for the array-based stack, what is the missing code?
def push (self, item):
self.size += 1
0.38 Points
-
items[size] = len(self.item)
-
item = self.items[self.size + 1]
-
self.items[len(self)] = item
-
self.items += 1
35)
In the linked implementation of a stack, what type of helper function simplifies the __iter__ method?
0.38 Points
-
random
-
binary
-
recursive
-
sequential
Saved
36)
Queues are linear collections.
0.38 Points
-
True
-
False
Saved
37)
A checkout line at a grocery store is an example of a queue.
0.38 Points
-
True
-
False
38)
In a priority queue, items waiting in the queue the longest are always popped next.
0.38 Points
-
True
-
False
39)
The list data structure in Python cannot be used to emulate a queue because there is no pop method.
0.38 Points
-
True
-
False
40)
Performing a pop operation on an empty queue throws an exception.
0.38 Points
-
True
-
False
41)
Simulation programs can use a range of integer values to mimic variability in the simulation.
0.38 Points
-
True
-
False
42)
The ready queue contains processes waiting to use the CPU.
0.38 Points
-
True
-
False
43)
What happens to processes on the ready queue in a round-robin CPU scheduling scheme?
0.38 Points
-
they are pushed and put in the priority queue
-
they are pushed to the end of the queue
-
they are popped and allocated memory
-
they are popped and given a slice of CPU time
44)
In a linked implementation of a queue, what does the add operation do?
0.38 Points
-
adds a node to the tail
-
inserts a node in the middle
-
adds a node to the head
-
creates a new instance of the queue
45)
In the following code for the add method for a linked queue implementation, what is the missing code?
def add(self, newItem):
newNode = Node(newItem, None)
if self.isEmpty():
self.front = newNode
else:
self.rear.next = newNode
self.size += 1
0.38 Points
-
self.rear = newNode
-
self.rear -= 1
-
self.front = self.next
-
self.rear.prev = None
46)
What is the solution to achieving good performance for both the add and pop methods in the array implementation of a queue?
0.38 Points
-
using an insert function instead of add
-
using a front pointer that advanced through the array
-
using a circular array implementation
-
using a fixed front pointer to index position 0
47)
What can you do if items in a priority queue cannot use the comparison operators?
0.38 Points
-
remove them in FIFO order
-
put them in the back of the queue
-
user a wrapper class
-
remove them from the queue
48)
In the following code for the __eq__ method for the Comparable class, what is the missing code?
def __eq__(self, other):
if self is other: return True
if type(self) != type(other):
return self.priority == other.priority
0.38 Points
-
return False
-
return self.priority
-
return type(other)
-
return other
49)
In the linked priority queue, what is the time and space analysis for the add method?
0.38 Points
-
O(n2)
-
O(n)
-
exponential
-
logarithmic
Step by Step Solution
There are 3 Steps involved in it
Step: 1
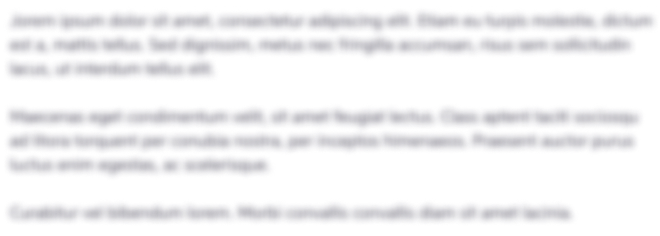
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started