Question
a few questions that you have to answer and an implementation you have to code. Write the answer in the __place indicated in this file__.
a few questions that you have to answer and an implementation you have to code. Write the answer in the __place indicated in this file__.
question:
You need to develop a multithreaded application that interacts with the user even if it is performing another task in the background. For example, the app could be downloading a file from the internet and still allows you to play the guess the number game.
async.cpp :
#include
std::random_device e; std::uniform_int_distribution<> dist(0, 5); //function download "simulates" the action of downloading // a file. It does this by sleeping for //@time seconds. After that it returns //@value int download(int time,int value) { std::this_thread::sleep_for(std::chrono::seconds(time)); return value; } int main() { int x; /* total correct guesses */ int sum = 0; /* total generated numbers */ int total = 0; /*call function download asynchronously*/
/* play guess correct number while waiting for the result of download*/ std::cout << "waiting... ";
do { ++total; /* wait 100 milliseconds for download */
std::cout << "enter a number "; int g = dist(e); std::cin >> x; if (g == x) { std::cout << "you guessed correct "; ++sum; } else { std::cout << " you guessed " << x << " value is " << g << " "; } //should exit the while when download returns } while (1); std::cout << "correct " << sum <<" out of "< } Read the file **main.cpp** in the folder __async__. The function download "simulates" a task that takes time (e.g. downloading a large file) by sleeping for a certain time. After that it returns a value. In the main you need to start the function __download__ asynchronously and while it is performing its task you play the guess the number game until __download__ finishes. Using c++ async and futures to implement the above behavior.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
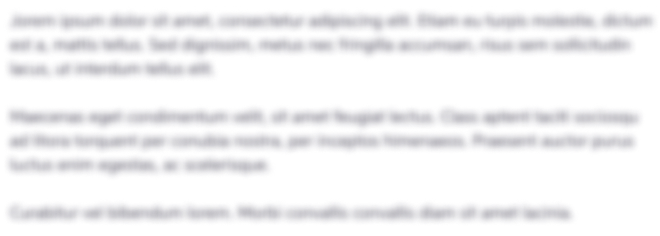
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started