Question
Application Requirements General Requirements This application must be written using the following Java Secure Coding Guidelines (as covered in class): No subclassing allowed (code reuse
Application Requirements General Requirements This application must be written using the following Java Secure Coding Guidelines (as covered in class):
No subclassing allowed (code reuse is to be done via composition)
Classes can only be instantiated using static constructor methods, e.g. getInstance(). Normal constructors must be declared as private Objects must be immutable (editing an object means replacing it with a new instance)
User input to the application must be validated. It must not be possible to store invalid attribute values in objects used in the system. Attempting to do so should display an error on-screen, and redisplay the menu to the user
The application must run under the supervision of a SecurityManager with an appropriate policy file to allow data saving and loading
The BaseComputer, LaptopComputer and DesktopComputer classes must be accessed from a sealed .jar file called Domain.jar when the application is run
Exceptions must be sanitized of sensitive data, e.g. file names and paths
Development Platform It is recommended to develop this application in the Kali VM using gedit as the code editor.
This will help you understand the structure of Java code for an application like this, i.e. how packages relate to subdirectories, etc., as well as the compilation and packaging of a Java application from the command line.
Using an IDE hides these details from you as a developer, and these will be important at times.
NOTE: Do not submit an IDE-based project in completion of this assignment, i.e. from NetBeans or any other IDE. See the Application submission structure section at the end of this document for details on expected submitted code structure). Submitting an IDE-based solution will result in a decreased mark for this assignment!
Runtime Platform The application must run in a terminal window in the Kali VM provided for the course.
The current directory in the terminal when the command line below is executed must be /root/ManageComputers. The application must run using the following command line: java -cp .:Domain.jar -Djava.security.manager -Djava.security.policy=security.policy ManageComputers 17 of 19 In the /root/ManageComputers directory, when the command line is executed, must be the following files:
The Domain.jar file (a sealed .jar file that contains the classes in the domain package classes: BaseComputer, LaptopComputer and DesktopComputer)
The security.policy file is a SecurityManager policy file that contains the permission settings for the application (specify one permission stating that it can read and write the /root/assign1Data directory, and a separate one that states it can perform read, write and delete operations on any files in that directory)
The ManageComputers.class file Application Structure The following files must exist in your solution:
ManageComputers.java: this contains the main() method for your application, and other methods as required o When run the main() method creates the ArrayList used to store the computer data for the application. This is where user-entered data, and data loaded from a file, is stored and accessed by the application
domain package:
o BaseComputer.java: holds the CPU type (String), RAM size (int) and disk size (int) for any computer o LaptopComputer.java: holds an instance of BaseComputer, and additionally the screen size (int) for the laptop
o DesktopComputer.java: holds an instance of BaseComputer, and additionally the GPU type (String) for the desktop MANIFEST.MF: contains the information used to seal the domain package
security.policy: the SecurityManager policy file containing permissions allowing the application to work with files in the /root/assign1Data directory Data Storage and Retrieval Computer data (i.e. for LaptopComputer and DesktopComputer objects) must be stored in serialized form on a drive.
The data must be held in files in /root/assign1Data. Each laptop and desktop object in the ArrayList maintained by the application must be stored separately in its own serialized file in the assign1Data directory (named 1.txt, 2.txt, 3.txt etc.).
Any String attribute data must be encrypted so that it cannot be easily read in the serialized data files.
Numeric attribute data is not easily readable in serialized files and does not have to be explicitly encrypted. Unsaved data in memory will be lost if the user closes the application without saving it. 18 of 19 Data in memory will be completely replaced with data loaded from the drive when the user chooses to load data. The assign1Data directory will be completely cleared of any existing files, and have new files written into it from the ArrayList, when the user chooses to save the in-memory application data. Object String Data Encryption When serializing objects (during a save operation), any String attribute data should be encrypted by shifting all of the characters in the String one place to the right in the alphabet (a Caesar Cipher approach to data encryption). Looking directly at the contents of the serialized object files on the drive should not show the unencrypted String data in the files. When deserializing object data (during a load operation) the String character data loaded from the files should be shifted one place to the left (to decrypt the String data). Hint: The best way to do this is to implement private readObject() and writeObject() methods in any class that is going to be serialized/deserialized. Application Submission Structure Your submission for this assignment must follow the following standard structure: An overall file called ITSCassign1.zip which contains: o A subdirectory called source. This contains all of the source code files for your solution (including correct subdirectories for packages, etc.), MANIFEST.MF and security.policy file. All the files required to build your application must be located here o Another subdirectory called ManageComputers. This directory must contain the compiled ManageComputers.class file, the pre-built Domain.jar file, and the security.policy file It must be possible to change into this directory and enter the command line specified earlier to run your application without further work (you can assume that the /root/assign1Data directory already exists)
**You are tasked with writing a console-based (text-based) Java application called ManageComputers that manages information about computers for a company. The company owns laptop and desktop computers and needs to store, display and manage the data about the specifications for the machines. You will use principles from the Java Secure Coding Guidelines document in the development of this application.**
(0) Showing the menu When the application is run it should display the following menu: The user can enter a number for their selection, e.g. 3 to list the details of all the computers stored in the system.
(1) Listing the Computers (List) If the user enters 3 at the menu: 3 of 19 In this case there is no computer data available to the system yet, so the application simply redisplays the menu again. In the event that there is data for a computer(s) in the system then it should display as follows: In the example above there is data for a laptop and a desktop computer that can be displayed. The computers are numbered based upon the order they were stored in the system. Note that the data for laptop and desktop computers is a little different, i.e. laptops specify screen size as a specification detail whereas desktops specify GPU type instead.
sample output:
Step by Step Solution
There are 3 Steps involved in it
Step: 1
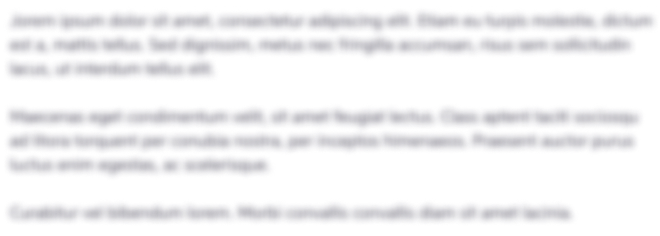
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started