Answered step by step
Verified Expert Solution
Question
1 Approved Answer
Below is the current code I have. How do I edit it so I can input multiple variables for my objective function and input a
Below is the current code I have. How do I edit it so I can input multiple variables for my objective function and input a gradient rather than a D vector for the derivative function?
For example, I want my objective function to be fxx xx xx
import numpy as np
#Algorithm Bracketing Phase for Line Search Algorithm
#The bracketing phase finds an interval within which we are certain
#to find a point that satisfies the strong Wolfe conditions.
def linesearchbracketingphi phiprime, alphainit, mu mu sigma:
#Inputs:
#phi objective function
#phiprime objective function derivative
#alphainit initial step size
#mudecrease factor
a #Define Initial Bracket this is the initial point as well?
phi phi
phiprime phiprime
a alphainit
phi phi
phiprime phiprime #Used in Pinpoint
first True
#Iteration Loop to Find Suitable Step Size to satisfy Wolfe conditions
while True:
phi phia #Calculate value for step point
phiprime phiprimea #Calculate derivative for step point
#if step point is greater than sufficient decrease line OR greater than initial point
if phi phi mu a phiprime or not first and phi phi:
astar pinpointphi phiprime, a a #run pinpoint function
return astar
phiprimeabs npabsphiprime
#if magnitude of slope of step point is close enough to zero then exit line search
if phiprimeabs mu phiprime:
return a
#if magnitude of slope of step point is too great, call pinpoint function
elif phiprime :
astar pinpointphi phiprime, a a
return astar
#Shift all values over to search again
a a
phi phi
phiprime phiprime
a sigma a
first False
#Algorithm Pinpoint function for the line search algorithm
#The pinpointing phase finds a point that satisfies the strong Wolfe
#conditions within the interval provided by the bracketing phase.
def pinpointphi phiprime, alow, ahigh, mu mu:
k
while True:
# Cubic interpolation Call function to locate potential minimum between a and a
ap cubicinterpolationphi phiprime, alow, ahigh
phip phiap
phipprime phiprimeap
#Check wolfe conditions and update interval accordingly.
#If conditions are met, return step size
if phip phi mu ap phi:
ahigh ap
elif phipprime mu phi:
return ap
elif phipprime ahigh alow:
ahigh alow
alow ap
k
#Cubic Interpolation Choses points within the interval, connects the points using cubic polynomial fit
#and finds the minimum of the three points to return the interpolated step size.
def cubicinterpolationphi phiprime, alow, ahigh:
# Perform cubic interpolation using polyfit
alphavalues nparrayalow, alow ahigh ahigh #Bisection Method array of points
phivalues nparrayphialpha for alpha in alphavalues #Find phi values of three points
phiprimevalues nparrayphiprimealpha for alpha in alphavalues #Find sloped of three points
# Fit a cubic polynomial to the data
coefficients nppolyfitalphavalues, phivalues,
# Find the minimum of the cubic polynomial
ap coefficients coefficients
return ap #return minimum point between original a and a as calcuulated by cubic interpolation
#
# Define your phi and phiprime functions
#Objective Function
def phialpha:
return npsumalpha
#Objective Function Derivative
def phiprimealpha:
return alpha
# Set initial parameters
#Initial Step Size
alphainit
#Decrease Factor
sigma
# Perform line search bracketing
optimalstep linesearchbracketingphi phiprime, alphainit, mu mu sigma
printOptimal Step:", optimalstep
Step by Step Solution
There are 3 Steps involved in it
Step: 1
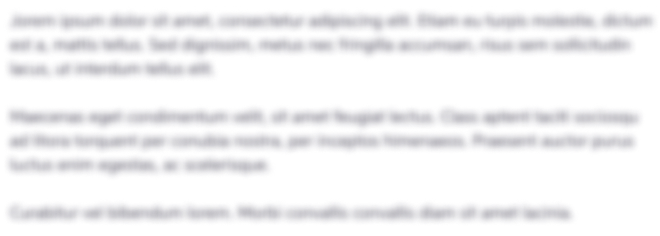
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started