Question
C++ Add a copy constructor to the FeetInches class. This constructor should accept a FeetInches object as an argument. The constructor should assign to the
C++
Add a copy constructor to the FeetInches class. This constructor should accept a FeetInches object as an argument. The constructor should assign to the feet attribute the value in the arguments feet attribute, and assign to the inches attribute the value in the arguments inches attribute. As a result, the new object will be a copy of the argument object.
Next, add a multiply member function to the FeetInches class. The multiply function should accept a FeetInches object as an argument. The argument objects feet and inches attributes will be multiplied by the calling objects feet and inches attributes, and a FeetInches object containing the result will be returned.
#ifndef FEETINCHES_H
#define FEETINCHES_H
#include
using namespace std;
class FeetInches; // Forward Declaration
// Function Prototypes for Overloaded Stream Operators
ostream &operator << (ostream &, const FeetInches &);
istream &operator >> (istream &, FeetInches &);
class FeetInches
{
private
int feet;
int inches;
void simplify();
public:
FeetInches(int f = 0, int i = 0)
{ feet = f;
inches = i;
simplify(); }
void setFeet(int f)
{ feet = f; }
void setInches(int i)
{ inches = i;
simplify(); }
int getFeet() const
{ return feet; }
int getInches() const
{ return inches; }
FeetInches operator + (const FeetInches &);
FeetInches operator (const FeetInches &);
FeetInches operator ++ ();
FeetInches operator ++ (int);
bool operator > (const FeetInches &);
bool operator < (const FeetInches &);
bool operator == (const FeetInches &);
friend ostream &operator << (ostream &, const FeetInches &);
friend istream &operator >> (istream &, FeetInches &);
};
#endif
Instructions
1. Redefine stream operators
2. Include Overloaded constructors functions
3. Include Mutators functions
4. Include Accessors functions
5. Implement a menu using switch and case
6. Redefine relation operators ( >,=,<=)
Step by Step Solution
There are 3 Steps involved in it
Step: 1
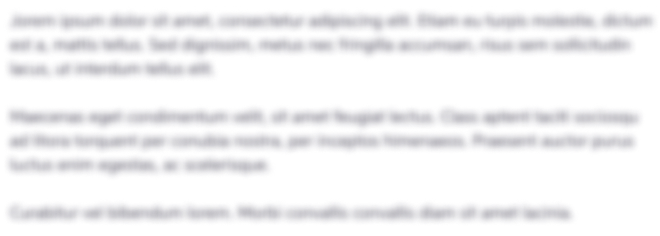
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started