Question
C++ data structures assignment, please help me. Please provide code text and a screenshot of the output if need to add more information please let
C++ data structures assignment, please help me. Please provide code text and a screenshot of the output if need to add more information please let me know. the code does not have to be perfect I just need something that will run. Thank You!
RecursionTest.cpp
// RecursionTest.cpp : This file contains the 'main' function. Program execution begins and ends there.
//
#include "pch.h"
#include
#include
using namespace std;
string RecurseCommas(int left, int right)
{
if( left == 0 )
return to_string(right);
else
return RecurseCommas(left / 1000, left % 1000) + "," + to_string(right);
}
string PutCommas(int x)
{
return RecurseCommas(x / 1000, x % 1000);
}
int main()
{
std::cout
}
// Run program: Ctrl + F5 or Debug > Start Without Debugging menu
// Debug program: F5 or Debug > Start Debugging menu
// Tips for Getting Started:
// 1. Use the Solution Explorer window to add/manage files
// 2. Use the Team Explorer window to connect to source control
// 3. Use the Output window to see build output and other messages
// 4. Use the Error List window to view errors
// 5. Go to Project > Add New Item to create new code files, or Project > Add Existing Item to add existing code files to the project
// 6. In the future, to open this project again, go to File > Open > Project and select the .sln file
RecursiveTest.cpp
// RecursiveTest.cpp : Defines the entry point for the console application.
//
#include "stdafx.h"
#include
#include
using namespace std; // ONLY IN CPP FILES
void PrintBackwardsRecursive(string tWhat)
{
// English definition
// Print the rest of the letters, then print the first.
if( tWhat.length() > 1)// Stops infinite recursion, and sometimes horrible crashes. (Base case)
PrintBackwardsRecursive(tWhat.substr(1));
cout
}
void PrintBackwardsDriver(string wat)
{
PrintBackwardsRecursive(wat);
}
int main()
{
string word = "Monkeypants";
// Yes, I know printing backwards could be done in a loop, this is academical
PrintBackwardsDriver(word);
return 0;
}
MAZE.H
#pragma once
#include
#include
#include
class Maze
{
public:
class Cell
{
friend Maze;
Cell* mNorth = nullptr; // While this "hardcodes" four directions, it is hella easier and 99% of mazes are flat and square
Cell* mSouth = nullptr;
Cell* mEast = nullptr;
Cell* mWest = nullptr;
bool mIsExit = false;
bool mProcessed = false;// Prevent loops Treat finding a processed cell as just not there. Like a dead end.
};
private:
bool MazeRecursive( Cell* tCurrent, std::stack<:string>* tPath );
Cell* mStart;
public:
Maze();// Making a constructor with a file name would be cool for the extra credit.
~Maze();
std::stack<:string> SolveMaze();
};
Maze.cpp
#include "Maze.h"
Maze::Maze()
{
// Making a test maze by hand to make testing easy without giving away the extra credit of the maze maker.
mStart = new Cell;
mStart->mNorth = new Cell;
mStart->mNorth->mNorth = new Cell;
mStart->mEast = new Cell;
mStart->mEast->mEast = new Cell;
mStart->mEast->mEast->mNorth = new Cell;
mStart->mEast->mEast->mNorth->mNorth = new Cell;
mStart->mEast->mEast->mNorth->mNorth->mIsExit = true;
// This is a big U
}
Maze::~Maze()
{
// Totally leaks
}
bool Maze::MazeRecursive( Maze::Cell* tCurrent, std::stack<:string>* tPath )
{
// This is the main part. Just keep in mind the single sentence recursive definition:
// "To exit a maze, I take a step and if I'm not done I exit the maze."
// Use Processed to prevent loops. The last cell is marked IsExit.
// "tPath" is there for you to push the path as you move. If
// it turns out you didn't find the exit that direction then pop it back off. The return value
// of true-false is how you communicate success backwards.
return false;//stub
}
std::stack<:string> Maze::SolveMaze()// Driver
{
// Don't need to change this.
std::stack<:string> tAnswer;
MazeRecursive( mStart, &tAnswer );
return tAnswer;
}
1) Cells in a maze are connected in both directions. If you forget that, the default maze only works by luck. They happen to be connected in a way that leads to the exit. 2) You never go backwards! Ever ever. If copy three of the func calls a fifth copy on the north pointer, when the fifth copy finds it is stuck it just ends. Then the fourth picks up where it left off as if it never went that way. It doesn't make a sixth copy backwards. 3) Inside a single instance of the function, Current will NOT change. When you want to go north, you don't physically move your cell to the north. Current is the cell. If you want to do something with the cell to the north, they are tCurrent->mNorth. In a huge maze with 50 copies of the function on the call stack, each version would have a different Current pointer. Each cell has one. 5-13 #5) We want to escape a maze using recursion. We can't use a loop because it forks. We also need the actual resulting path. With apologies to the book author, their answer is stupid. Instead of tracking the places you need to check, just try them. If they don't work out, then forget them. The difference is I'm using backtracking, and they are using breadth search. (We're doing tree theory stuff next week.) pseudocode: Return true if we are the exit Push self on answer stack and mark self processed. for each exit, Recurse on it if any recurse returns true, yay! just keep returning true all the way back. if none return true, pop us off answer stack and die quietly Big extra credit: Write the destructor. Warning: comments may lie. Small Extra credit: Load a text file and make a maze out of it. A sample text file is in the handouts. Border is guaranteed to be solid X's. You are responsible for testing your own code! My constructor maze doesn't even have pointers going both ways. It is NOWHERE NEAR a real test. SP21: Steal idea to only push the letter inside the "true" instead of pushing it always and popping in the "false". I swear you can do the 13 with only MazeRecursive and Main You can do the next with just the destructor. But if you need to be invasive for the last part, go ahead AS LONG as you aren't changing the core functionality. (No Djikstra, no BFS, etc) 1) Cells in a maze are connected in both directions. If you forget that, the default maze only works by luck. They happen to be connected in a way that leads to the exit. 2) You never go backwards! Ever ever. If copy three of the func calls a fifth copy on the north pointer, when the fifth copy finds it is stuck it just ends. Then the fourth picks up where it left off as if it never went that way. It doesn't make a sixth copy backwards. 3) Inside a single instance of the function, Current will NOT change. When you want to go north, you don't physically move your cell to the north. Current is the cell. If you want to do something with the cell to the north, they are tCurrent->mNorth. In a huge maze with 50 copies of the function on the call stack, each version would have a different Current pointer. Each cell has one. 5-13 #5) We want to escape a maze using recursion. We can't use a loop because it forks. We also need the actual resulting path. With apologies to the book author, their answer is stupid. Instead of tracking the places you need to check, just try them. If they don't work out, then forget them. The difference is I'm using backtracking, and they are using breadth search. (We're doing tree theory stuff next week.) pseudocode: Return true if we are the exit Push self on answer stack and mark self processed. for each exit, Recurse on it if any recurse returns true, yay! just keep returning true all the way back. if none return true, pop us off answer stack and die quietly Big extra credit: Write the destructor. Warning: comments may lie. Small Extra credit: Load a text file and make a maze out of it. A sample text file is in the handouts. Border is guaranteed to be solid X's. You are responsible for testing your own code! My constructor maze doesn't even have pointers going both ways. It is NOWHERE NEAR a real test. SP21: Steal idea to only push the letter inside the "true" instead of pushing it always and popping in the "false". I swear you can do the 13 with only MazeRecursive and Main You can do the next with just the destructor. But if you need to be invasive for the last part, go ahead AS LONG as you aren't changing the core functionality. (No Djikstra, no BFS, etc)
Step by Step Solution
There are 3 Steps involved in it
Step: 1
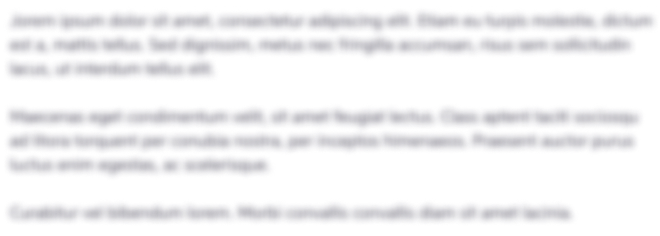
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started