Question
C# LinkedList with three data fields I'm trying to write a LinkedList program that will take three data fields (two strings and one double), as
C# LinkedList with three data fields I'm trying to write a LinkedList program that will take three data fields (two strings and one double), as properties for book keeping (author, title, price). Bascially, each node (book) should have three data fields, author name, title of book, and price. The class should have an Add, Remove, and PrintAll method. In the main program, the console should ask for user input to add, remove, or print the properties of nodes in the list. Mostly, I am confused on how I should implement the MultiLinkedListOfBooks class. Here is the code I have so far.
using System; using System.Collections.Generic; using System.Linq; using System.Text; using System.Threading.Tasks;
namespace Book_Keeper { public class Program { static void Main(string[] args) { UserInterface ui = new UserInterface(); ui.RunProgram(); } }
public enum MenuOptions { QUIT = 1, ADD_BOOK = 2, PRINT = 3, REMOVE_BOOK = 4, RUN_TESTS = 5 }
public enum ErrorCode { OK, DuplicateBook, BookNotFound }
public class UserInterface { MultiLinkedListOfBooks myList; public void RunProgram() { int choice; myList = new MultiLinkedListOfBooks(); do //main do-while loop { Console.WriteLine("Your options:"); Console.WriteLine("{0} : End the program", (int)MenuOptions.QUIT); Console.WriteLine("{0} : Add a book", (int)MenuOptions.ADD_BOOK); Console.WriteLine("{0} : Print all books", (int)MenuOptions.PRINT); Console.WriteLine("{0} : Remove a Book", (int)MenuOptions.REMOVE_BOOK); Console.WriteLine("{0} : RUN TESTS", (int)MenuOptions.RUN_TESTS); if (!Int32.TryParse(Console.ReadLine(), out choice)) { Console.WriteLine("You need to type in a valid, whole number!"); continue; } switch ((MenuOptions)choice) { case MenuOptions.QUIT: Console.WriteLine("Thank you for using the multi-list program!"); break; case MenuOptions.ADD_BOOK: this.AddBook(); break; case MenuOptions.PRINT: myList.PrintAll(); break; case MenuOptions.REMOVE_BOOK: this.RemoveBook(); break; case MenuOptions.RUN_TESTS: AllTests tester = new AllTests(); tester.RunTests(); break; default: Console.WriteLine("I'm sorry, but that wasn't a valid menu option"); break; } } while (choice != (int)MenuOptions.QUIT); }
public void AddBook() { Console.WriteLine("ADD A BOOK!"); Console.WriteLine("Author name?"); string author = Console.ReadLine(); Console.WriteLine("Title?"); string title = Console.ReadLine(); double price = -1; while (price < 0) { Console.WriteLine("Price?"); if (!Double.TryParse(Console.ReadLine(), out price)) { Console.WriteLine("I'm sorry, but that's not a number!"); price = -1; } else if (price < 0) { Console.WriteLine("I'm sorry, but the number must be zero, or greater!!"); } } ErrorCode ec = myList.Add(author, title, price); //Error checking code goes here }
public void RemoveBook() { Console.WriteLine("REMOVE A BOOK!"); Console.WriteLine("Author name?"); string author = Console.ReadLine(); Console.WriteLine("Title?"); string title = Console.ReadLine(); ErrorCode ec = myList.Remove(author, title); //Error checking code goes here } }
public class MultiLinkedListOfBooks { private BookNode head; //private data fields private string AUTHOR; private string TITLE; private double PRICE; private int SIZE;
public MultiLinkedListOfBooks() //initial constructor { this.head = null; this.AUTHOR = null; this.TITLE = null; this.PRICE = 0.00; this.SIZE = 0; }
public int getSize() //returns size of the linked list { return this.SIZE; }
public class BookNode { public string author; //stores the book properties public string title; public double price; public BookNode next;
public BookNode(string a, string t, double p) //constructor with parameters { author = a; title = t; price = p; } }
public void PrintAll() //prints all books, or if list is empty, say so { Console.WriteLine("Title: " + TITLE); Console.WriteLine("Author: " + AUTHOR); Console.WriteLine("Price: " + PRICE); }
public ErrorCode Add(string author, string title, double price) { if (head == null) //if list is empty { BookNode b = new BookNode(AUTHOR, TITLE, PRICE); head = b; } else { BookNode nb = new BookNode(AUTHOR, TITLE, PRICE); nb.next = head; head = nb; } return ErrorCode.DuplicateBook; //if book is already in list }
public ErrorCode Remove(string author, string title) { return ErrorCode.BookNotFound; //if not an exact match } }
class AllTests { public void RunTests() {
} } }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
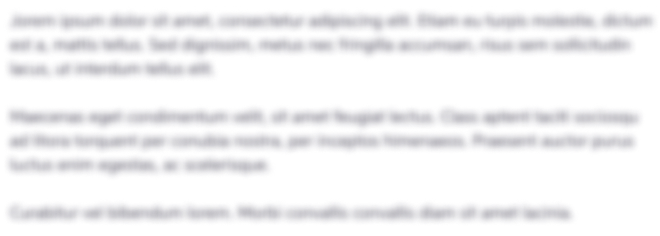
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started