Question
C++ Plinko With functions Here is my code that needs to be modified with the Lab 6 requirements. A new code needs to be created
C++ Plinko With functions
Here is my code that needs to be modified with the Lab 6 requirements. A new code needs to be created from my code.
CODE
#include #include #include #include #include using namespace std; int main() {
int i = 0; int j = 0; int slotChange = 0; int menuOption = 0; int numChips = 0; int chipSlotStart = 0; double chipSlot = 0.0; double winnings = 0.0; double winningTotal = 0.0; double averageTotal = 0.0; const double SLOT_CHANGE_VAL = 0.5; const int SEED_VAL = 42; const int LOWER_BOUND = 0; const int UPPER_BOUND = 8; const int CHIP_SLOT_PRECISION = 1; const int GAME_QUIT = 0; srand(SEED_VAL);
cout << "Welcome to the Plinko simulator!" << endl << endl; do { cout << "Menu: Please select one of the following options:" << endl << endl; cout << "0 - Quit the program" << endl << "1 - Drop a single chip into one slot" << endl << "2 - Drop multiple chips into one slot" << endl << "3 - Drop multiple chips into each slot" << endl << endl; cout << "Enter your selection now: "; cin >> menuChoice; cout << endl; //game exit option if (menuOption == GAME_QUIT) {} //game option one else if (menuChoice == 1) { cout << "*** Drop a single chip ***" << endl << endl; cout << "Which slot do you want to drop the chip in (0-8)? " << endl;
cin >> slotNumber; //chip value must be between 0-8
}
//game option 2: multiple chips else if (menuOption == 2) { cout << "*** Drop multiple chips ***" << endl << endl; cout << "How many chips do you want to drop (>0)? " << endl; cin >> numChips;
if (numChips > 0) { cout << "Which slot do you want to drop the chip in (0-8)? " << endl;
cin >> chipSlotStart; winningTotal = 0;
//calculates final chip location for each chip double ComputeWinnings(double location) { double prize; const double PRIZE_0 = 100.00; const double PRIZE_1 = 500.00; const double PRIZE_2 = 1000.00; const double PRIZE_3 = 0.00; const double PRIZE_4 = 10000.00; const double PRIZE_5 = 0.00; const double PRIZE_6 = 1000.00; const double PRIZE_7 = 500.00; const double PRIZE_8 = 100.00;
if (location == 0) { prize = PRIZE_0; } else if (location == 1) { prize = PRIZE_1; } else if (location == 2) { prize = PRIZE_2; } else if (location == 3) { prize = PRIZE_3; } else if (location == 4) { prize = PRIZE_4; } else if (location == 5) { prize = PRIZE_5; } else if (location == 6) { prize = PRIZE_6; } else if (location == 7) { prize = PRIZE_7; } else if (location == 8) { prize = PRIZE_8; }
return prize;
} double singleChip(chipSlotStart, numChip) { double location = chipSlotStart; if (chipSlotStart >= 0 && chipSlotStart <= 8) { for (int i = 0; i < 12; i++) { double randm = rand() % 2; if (random < 1) { location = location - 0.5; } else { location = location + 0.5; } if (location < 0) { location = 0.5; } else if (location > 8) { location = 7.5; } } } return location; } double multipleChips(chipSlotStart, numChips) { double winningTotal = 0; double locaation = chipSlotStart; if (numChips > 0) { if (chipSlotStart >= 0 && i < numChips; i++) { location = singleChip(chipSlotStart, numChips) winningTotal = winningTotal + ComputWinnings(location); //cout << location; } } } //total and average winnings outputs double averageTotal = winningTotal / numChips; cout << "Total winnings on " << numChips << " chips: " << fixed << setprecision(2) << winningTotal << endl;
cout << "Average winnings per chip: " << fixed << setprecision(2) << averageTotal << endl << endl; } else { cout << "Invalid slot." << endl << endl; }
} else { cout << "Invalid number of chips." << endl << endl; } }
else /*if ((menuOption != GAME_QUIT) && (menuOption != 1) && (menuOption != 2))*/ { cout << "Invalid selection. Please enter 0, 1, 2, or 3" << endl << endl; }
} while (menuOption != GAME_QUIT);
cout << "Goodbye!";
return 0; }
LAB 6 PORTION
Part 1 - Refactoring (27 points)
"Refactor" your Lab 4 code (i.e. preferably do not rewrite Lab 4 from scratch) using functions. Wikipedia explains that "Code refactoring is the process of restructuring existing computer code... Advantages include improved code readability and reduced complexity... Typically, refactoring applies a series of standardised basic micro-refactorings, each of which is (usually) a tiny change in a computer program's source code that ... preserves the behaviour of the software". In the end we really cannot tell if you refactored your lab 4 code or just re-wrote it from scratch, but we encourage you to start with your code for lab 4 and move the code around to achieve a simpler, better program. Before refactoring, you are strongly encouraged to COPY your Lab 4 code into a new solution for Lab 6 (rather than to edit your Lab 4 code directly). This way, if your Lab 6 code gets too scrambled, you still have your Lab 4 code from which to start over. In particular we are expecting to see functions for:
Computing the amount of prize money won for a single chip given the slot the chip ended up in-- this function must be defined as "double ComputeWinnings(int slot) {... your code here ...}. This just needs to implement the fact that if the chip ends up in slot 0 you get $100.00, if it ends up in slot 1 your get $500.00, etc. (See the original Plinko lab for the complete list of these values).
Simulating one chip falling-- you will have to use your discretion on how you set this up, but it should make both the "Drop a single chip into one slot" option and the next function (Simulating multiple chips falling) easier to write. This function must make use of the "ComputeWinnings" function described above.
Simulating multiple chips falling-- similarly this function should make both the "Drop multiple chips into one slot" option and a new "Drop multiple chips into each slot" option (described below) easier to write. This function must make use of the "Simulating one chip falling" function described above.
Your Lab 6 solution must include the major features of Lab 4, however do not print the path for the single drop case.
Add a new menu item for "Drop multiple chips into each slot", but you do not have to implement it yet. You will implement it in Part 3.
We will also change the error handling, so do not worry about that for Part 1.
Part 2 - Better Error Handling (27 points)
Add a function for getting the number of chips and a function for getting the slot number. Within these functions check for user input error and reprompt until the user provides valid input. You must be able to recognize erroneous input like "-1", "Not even a number", and in the case of a slot number, invalid input like "9". You may assume that there will be no real-valued inputs like "2.3" Note that due to the improved error handling approach, some of the error messages have been modified.
Add a similar function for the menu too, reprompting if bad input is given.
Part 3 - Functions and the Power of Code Reuse (21 points)
In this part of the lab, you will take advantage of functions to add an additional menu option to your Plinko program, without having to duplicate code from the other menu options.
Add a new menu option 3 that allows the user to drop the same number of chips into each of the 9 slots. For example, if the user enters 5, you simulate dropping 5 chips into each of the 9 slots.
Prompt the user to enter one number, which is the number of chips to drop into every slot. If the number of chips is non-positive, reprompt until you get valid input (hint: use the function you wrote in Part 2)
For each slot, report the total and average amount of money won for all chips dropped into that slot.
Implementation
Just as in the previous Plinko lab, in order to ensure that your random path matches the auto-grader you MUST:
Upon encountering a peg, a chip has a 50/50 chance of going left or right. Use "rand() % 2" to get a random value. If you get 0 decrease the chip position value, and if you get 1 increase the position value.
Note that in some cases the directions is forced and no call to ran() is needed. For example, this happens when the chip is in position 0, since the chip can not move to -0.5, it must go to 0.5. Likewise when in position 8, the chip can not go to position 8.5, so it must go to 7.5. In these cases you must not call rand(). Extra calls to rand() will get your program out of sync relative to what is required and cause your program to produce output that will not match the test files. Check first to see if you are in one of these edge cases and handle it appropriately, if not, then call rand() to get a random offset, as described above.
You must set the random seed in the main function. Normally we would use "srand(time(0))" to get (more nearly) random behavior. However, in this lab you must use srand(42) at the start of main. Note also that you may get different behavior on different computers, so if you are running this lab somewhere other than on zybooks, you may not get the output shown below.
Be careful to use constants in place of the magic values described above.
If you think about it for a minute you might realize that you could use a single function for the error testing in Part 2. Obviously it would need additional parameters to work as required in each case, but it is an interesting possibility. You may do so if you wish.
Items Graded by the TA's Inspection (25 points)
Adherence to style guidelines. Note this is the first time that the function section of the style guide comes into play, so read that section over carefully before beginning the lab.
New functions for
Computing the amount of prize money won for one chip given the slot it ended up in (called "ComputeWinnings") (5 point deduction if missing)
Simulating one chip falling (must use "ComputeWinnings") (5 point deduction if missing)
Simulating multiple chips falling (must use "Simulate one chip falling") (5 point deduction if missing)
New functions for menu, slot and chip input, with error handling. It could be a single function with additional parameters as noted in the implementation section (5 point deduction if missing)
Notes
The test cases start with just the input part, so you can start there and then build your solution step by part. Also, you can test your code as many times as you like.
Remember to review the functions section in the style guide. Note that one of the function style expectations is "Make sure that your naming of functions and parameters are sufficiently clear that you rarely need a comment header to describe the function and parameters."
Sample Run
Sample Input
56 1 10 5 2 -3 345 0 3 500 0
Sample Output
Welcome to the Plinko simulator! Menu: Please select one of the following options: 0 - Quit the program 1 - Drop a single chip into one slot 2 - Drop multiple chips into one slot 3 - Drop multiple chips into each slot Enter your selection now: 56 Invalid selection. Please enter 0, 1, 2 or 3 Enter your selection now: 1 *** Drop single chip *** Which slot do you want to drop the chip(s) in (0-8)? 10 Invalid slot, please try again. Which slot do you want to drop the chip(s) in (0-8)? 5 Winnings: $1000.00 Menu: Please select one of the following options: 0 - Quit the program 1 - Drop a single chip into one slot 2 - Drop multiple chips into one slot 3 - Drop multiple chips into each slot Enter your selection now: 2 *** Drop multiple chips *** How many chips do you want to drop (>0)? -3 Invalid number of chips, please try again. How many chips do you want to drop (>0)? 345 Which slot do you want to drop the chip(s) in (0-8)? 0 Total winnings on 345 chips: 274400.00 Average winnings per chip: 795.36 Menu: Please select one of the following options: 0 - Quit the program 1 - Drop a single chip into one slot 2 - Drop multiple chips into one slot 3 - Drop multiple chips into each slot Enter your selection now: 3 *** Sequentially drop multiple chips *** How many chips do you want to drop (>0)? 500 Total winnings on slot 0 chips: 413900.00 Average winnings per chip: 827.80 Total winnings on slot 1 chips: 487500.00 Average winnings per chip: 975.00 Total winnings on slot 2 chips: 813300.00 Average winnings per chip: 1626.60 Total winnings on slot 3 chips: 1105500.00 Average winnings per chip: 2211.00 Total winnings on slot 4 chips: 1132800.00 Average winnings per chip: 2265.60 Total winnings on slot 5 chips: 1114100.00 Average winnings per chip: 2228.20 Total winnings on slot 6 chips: 898300.00 Average winnings per chip: 1796.60 Total winnings on slot 7 chips: 538900.00 Average winnings per chip: 1077.80 Total winnings on slot 8 chips: 383700.00 Average winnings per chip: 767.40 Menu: Please select one of the following options: 0 - Quit the program 1 - Drop a single chip into one slot 2 - Drop multiple chips into one slot 3 - Drop multiple chips into each slot Enter your selection now: 0 Goodbye!
Step by Step Solution
There are 3 Steps involved in it
Step: 1
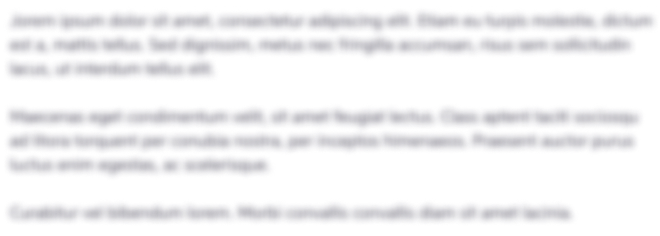
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started