Question
Can someone edit my code to the appropriate requirements and fix any errors included? Please keep it in C#. I have the instructions listen then
Can someone edit my code to the appropriate requirements and fix any errors included? Please keep it in C#. I have the instructions listen then included my code. Thank you for your help.
Concept Summary: 1. Object Oriented Programming Concepts 2. Abstract classes 3. Polymorphism Objective: Consider the scenario at a high school football ticket stand. Tickets for the games are sold at various times and have different prices depending on how early the ticket is purchased. In this programming assignment you will need to create four classes and a driver program. The parent class called Ticket should be an abstract class. Three classes, should extend the Ticket class and be called AdvanceBooking, CurrentBooking and DiscountBooking. Finally, create a test class called Driver. The rules for purchasing tickets are: CurrentBookings are for tickets purchased for games happening today. They cost $75 each AdvancedBookings are for tickets purchased for games happening in the future. If the game is less than 15 days in the future, tickets cost $50 each, if they are 15 or more days in the future, they cost $25 each. (Note if the NoOfDays =0 the program transfers control to the CurrentBookings class. Finally, students can purchase discount tickets for $10, as long as they are for games at least 1 day in the future otherwise they are $75. Assignment Requirements: The Ticket abstract class is the parent class that all other classes will extend. This class holds all common operations such that the subclasses can implement the methods specified here. This class should have the following methods: A constructor which generates a ticket with a ticketNumber (Integer). The class should have a variable called nextTicketNumber which should be initialized to 1. When each ticket is created, they should be assigned the next integer regardless of the type of ticket. A getPrice( ) method which returns the ticket price (In ticket it is an abstract method) An override of the toString / toString method that prints the type of ticket, ticket number, and price of ticket. The CurrentBooking class is a subclass that allows for tickets to be purchased on the day of the game. When created, each ticket should be assigned the next ticket number, and have their price set to $75. The AdvanceBooking class is a subclass that represents tickets purchased in advance. In order to create an AdvancedBooking, the number of days till the game (numDaysUntilGame an integer variable) must be passed in at the time the ticket is created. Like CurrentBookings, AdvancedBookings will be assigned the next ticket number. If the game is 15 days or more in the future, the ticket will cost $25. If the game is in less than 15 days, the ticket will cost $50. The DiscountBooking class is a subclass that allows for students to purchase tickets in advance. In order to create a DiscountBooking object, the number of days until the game (numDaysUntilGame an integer variable) must be passed in at the time the ticket is created. Like all other ticket types, the next ticket number will be assigned. If the game is today, the ticket price is the normal $75, however, if the game is any other day, it will cost $10. Driver Program The driver program should have a menu of options for a person to purchase and see tickets. The program should keep going until the user selects exit. The menu has the following options: 1. Sell a Ticket for a future gam 2. Sell a Ticket for todays game 3. Sell a Discount Ticket 4. Print All Tickets 5. Exit All input/output statements should be in the driver program only. All tickets should have a unique ticket number. As tickets are created, regardless of which type of ticket they are, they should be stored in an arraylist. If option 2 or 3 are selected, youll need to ask the user how many days in the future the game is. If option 4 is selected, it should print out all tickets which have been sold by simply calling print on the object name. As long as you successfully overrode toString/ToString it should print out a message including the ticket type, ticket number and price. Demonstrate polymorphism when creating the tickets and storing them in the ArrayList. Sample Output: Choose from the following: 1. Sell a Ticket for a future game 2. Sell a Ticket for today's game 3. Sell a Discount Ticket 4. Print All Tickets 5. Exit 1 How many days until the game? 16 You sold: Ticket number 1 with a price of $25 for a game in 16 days. Choose from the following: 1. Sell a Ticket for a future game 2. Sell a Ticket for today's game 3. Sell a Discount Ticket 4. Print All Tickets 5. Exit 3 How many days until the game? 14 You sold: Ticket number 2 with a price of $10 is a discount ticket for a game in 14 days. Choose from the following: 1. Sell a Ticket for a future game 2. Sell a Ticket for today's game 3. Sell a Discount Ticket 4. Print All Tickets 5. Exit 2 You sold: Ticket number 3 with a price of $75 for today's game Choose from the following: 1. Sell a Ticket for a future game 2. Sell a Ticket for today's game 3. Sell a Discount Ticket 4. Print All Tickets 5. Exit 3 How many days until the game? [0 for today] 7 You sold: Ticket number 4 with a price of $10 is a discount ticket for a game in 7 days. Choose from the following: 1. Sell a Ticket for a future game 2. Sell a Ticket for today's game 3. Sell a Discount Ticket 4. Print All Tickets 5. Exit 3 How many days until the game? [0 for today] 0 You sold: Ticket number 5 with a price of $75 is a discount ticket for a game in 0 days. Choose from the following: 1. Sell a Ticket for a future game 2. Sell a Ticket for today's game 3. Sell a Discount Ticket 4. Print All Tickets 5. Exit 1 How many days until the game? 22 You sold: Ticket number 6 with a price of $25 for a game in 22 days. Choose from the following: 1. Sell a Ticket for a future game 2. Sell a Ticket for today's game 3. Sell a Discount Ticket 4. Print All Tickets 5. Exit 1 How many days until the game? 1 You sold: Ticket number 7 with a price of $10 for a game in 1 days. Choose from the following: 1. Sell a Ticket for a future game 2. Sell a Ticket for today's game 3. Sell a Discount Ticket 4. Print All Tickets 5. Exit 4 Here are all the tickets you sold: Ticket number 1 with a price of $25 for a game in 16 days. Ticket number 2 with a price of $10 is a discount ticket for a game in 14 days. Ticket number 3 with a price of $75 for today's game Ticket number 4 with a price of $10 is a discount ticket for a game in 7 days. Ticket number 5 with a price of $75 is a discount ticket for a game in 0 days. Ticket number 6 with a price of $25 for a game in 22 days. Ticket number 7 with a price of $10 for a game in 1 days. Choose from the following: 1. Sell a Ticket for a future game 2. Sell a Ticket for today's game 3. Sell a Discount Ticket 4. Print All Tickets 5. Exit 6 Invalid Choice Choose from the following: 1. Sell a Ticket for a future game 2. Sell a Ticket for today's game 3. Sell a Discount Ticket 4. Print All Tickets 5. Exit 5
Code I have right now that isn't correct (Please do not combine files, I need each class to be a seperate file):
using System;
namespace TicketAssignment { public abstract class Ticket { private int ticketNumber; private static int nextTicketNumber = 1;
public Ticket() { ticketNumber = nextTicketNumber++; } public Ticket(int t) { ticketNumber = t; }
internal abstract int Price { get; }
internal virtual int TicketNumber { get { return ticketNumber; } } internal virtual int NextTicketNumber { get { return nextTicketNumber; } }
public override string ToString() { return "Ticket Number: " + ticketNumber; } } }
using System; using System.Collections.Generic; using System.Text;
namespace TicketAssignment { public class PurchaseTicket { public static void Main(string[] args) { Scanner scnr = new Scanner(System.in);
List
while (input != 5) { displayMenu(); input = scnr.Next();
switch (input) { case 1: Console.WriteLine("How many days until the game?"); temp = new AdvanceBooking(scnr.Next()); tickets.Add(temp); Console.WriteLine(temp); break; case 2: temp = new CurrentBooking(); tickets.Add(temp); Console.WriteLine(temp); break; case 3: Console.WriteLine("How many days until the game? [0 for today]"); temp = new DiscountBooking(scnr.Next()); tickets.Add(temp); Console.WriteLine(temp); break; case 4: Console.WriteLine("Here are all the tickets you sold:"); foreach (Ticket t in tickets) { Console.Write(t); } break; case 5: break; default: Console.WriteLine("Invalid Choice"); break; } }
scnr.close(); }
internal static void displayMenu() { Console.WriteLine("Choose from the following:"); Console.WriteLine("1. Sell a Ticket for a future game"); Console.WriteLine("2. Sell a Ticket for today's game"); Console.WriteLine("3. Sell a Discount Ticket"); Console.WriteLine("4. Print All Tickets"); Console.WriteLine("5. Exit"); } } }
using System; using System.Collections.Generic; using System.Text;
namespace TicketAssignment { public class DiscountBooking : Ticket { private int numDaysUntilGame, ticketPrice;
public DiscountBooking() : base() { numDaysUntilGame = 0; } public DiscountBooking(int n) : base() { numDaysUntilGame = n; if (numDaysUntilGame > 0) { ticketPrice = 10; } else { ticketPrice = 75; } }
internal virtual int Price { get { return ticketPrice; } set { ticketPrice = value; } } internal virtual int NumDaysUntilGame { get { return numDaysUntilGame; } }
internal virtual int DaysUntil { set { numDaysUntilGame = value; } }
public override string ToString() { return "Ticket number " + base.TicketNumber + " with a price of $" + Price + " is a discount ticket for a game in " + numDaysUntilGame + " days. "; } } }
using System; using System.Collections.Generic; using System.Text;
namespace TicketAssignment { public class CurrentBooking : Ticket {
private int ticketPrice;
public CurrentBooking() : base() { ticketPrice = 75; } public CurrentBooking(int p) : base() { ticketPrice = p; } public CurrentBooking(int t, int p) : base(t) { ticketPrice = p; }
internal virtual int Price { get { return ticketPrice; } set { ticketPrice = value; } }
public override string ToString() { return "You sold: Ticket number " + base.TicketNumber + " with a price of $" + Price + " for today's game. "; } } }
using System; using System.Collections.Generic; using System.Text;
namespace TicketAssignment { public class AdvanceBooking : Ticket { private int numDaysUntilGame, ticketPrice;
public AdvanceBooking() : base() { numDaysUntilGame = 0; ticketPrice = 0; } public AdvanceBooking(int n) : base() { numDaysUntilGame = n; if (numDaysUntilGame > 15) { ticketPrice = 25; } else { ticketPrice = 50; } }
internal virtual int Price { get { return ticketPrice; } set { ticketPrice = value; } } internal virtual int NumDaysUntilGame { get { return numDaysUntilGame; } }
internal virtual int DaysUntil { set { numDaysUntilGame = value; } }
public override string ToString() { return "You sold: Ticket number " + base.TicketNumber + " with a price of $" + Price + " for a game in " + numDaysUntilGame + " days. "; } } }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
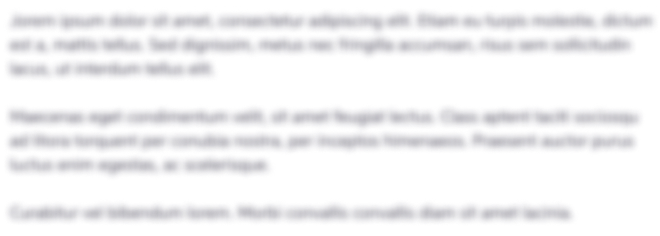
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started