Question
*Change arraylist code below to arrays* Take the Helper class of the previous assignment, and change it to use an array of 10 BestFriend objects,
*Change arraylist code below to arrays*
Take the Helper class of the previous assignment, and change it to use an array of 10 BestFriend objects, instead of anarrayList of BestFriend objects. Do all of the requirements of the assignment, but simulate "removing" the element by moving all the following elements up to previous index, and then moving nulls to the last element of the array that was moved to a previous index. You may wish to create a new method for this: shrinkArray(). You may need to pass parameter(s) to this method.
When the array becomes too small to fit a new BestFriend object, define a new array with double the size of the original array, move everything from the original array to the new array, and then reset the reference of the original array to now point to the new array. You may wish to create a new method for this: expandArray(); You may need to pass parameter(s) to this method.
********************************************************
import java.util.*;
public class BestFriend
{
private static int number = 0;
private int iD;
private String firstName;
private String lastName;
private String nickName;
private String cellPhoneNumber;
// constructor
public BestFriend(String aFirstName, String aLastName, String aNickName, String aCellPhoneNumber) {
firstName = aFirstName;
lastName = aLastName;
nickName = aNickName;
cellPhoneNumber = aCellPhoneNumber;
number++;
iD = number;
}
// orerride the toString() function which returns the String if the object is printed
public String toString() {
return iD + " " + firstName + " " + lastName + " " + cellPhoneNumber + " "+ ". " + nickName;
}
// setter methods
// set the first name
void setFirstName(String first) {
firstName = first;
}
// set the last name
void setLastName(String last) {
lastName = last;
}
// set the nick name
void setNickName(String nick) {
nickName = nick;
}
// set the cell phone number
void setCellPhoneNumber(String phone) {
cellPhoneNumber = phone;
}
public boolean equals(Object another) {
// if another is an object of class BestFriend
if (another instanceof BestFriend)
{
// type cast another to BestFriend class
BestFriend anotherBFF = (BestFriend) another;
// if the objects are equal
if (firstName.equalsIgnoreCase(anotherBFF.firstName) && lastName.equalsIgnoreCase(anotherBFF.lastName)) {
return true;
}
}
// if the objects are not equal
return false;
} //getter methods
public static int getNumber() {
return number;
}
public int getiD() {
return iD;
}
public String getFirstName() {
return firstName;
}
public String getLastName() {
return lastName;
}
public String getNickName() {
return nickName;
}
public String getCellPhoneNumber() {
return cellPhoneNumber;
}
}
*******************************************
import java.util.*;
public class BestFriendSimulation {
private static ArrayList
// function to display the menu to the user
public static void displayMenu()
{
String MainMenu = "Please Enter Option Number Of your Choice" + " " + "1.) Add BestFriend Contact " + " " + "2.) Change a BestFriend Contact" + " " + "3.) Remove a BestFriend from Phonebook " + " " + "4.) Display all the information in phonebook" + " " + "5.) Exit " + " ";
System.out.println(MainMenu);
}
// main method
public static void main(String arg[]) {
// create a Scanner class object to get user input
Scanner scanner = new Scanner(System.in);
// create an object of class BestFriendHelper
BestFriendHelper helper = new BestFriendHelper();
int option = 0;
// infinite loop
while (true) {
// display the menu
displayMenu();
option = scanner.nextInt();
// if the entered option is invalid
if (option <= 0 || option > 5) {
System.out.println("Invalid Input! Enter input between 1 to 5");
// go to the beginning of the loop
continue;
}
// if user enetered 1
if (option == 1) {
// call the add() method
helper.add();
// if user enetered 2
} else if (option == 2) {
// call the helper() method
helper.change();
// if user enetered 3
} else if (option == 3) {
// call the remove method
helper.remove();
// if user enetered 4
} else if (option == 4) {
// call the display method
helper.display();
// if user enetered 5
} else if (option == 5) {
System.out.println("Exiting");
// break out of the loop
break;
}
}
}
}
*****************************************************
import java.util.ArrayList;
import java.util.Scanner;
public class BestFriendHelper {
// create an object to store the objects fo type BestFriend
private ArrayList
// constructor
public BestFriendHelper() {
// initialize arraylist
best = new ArrayList
}
// function to add data to ArrayList
public void add() {
// create Scanner object to get user input
Scanner scanner = new Scanner(System.in);
System.out.println("Please Enter First Name");
// read the next element
String first = scanner.next();
System.out.println("Please Enter Last Name");
// read the next element
String last = scanner.next();
System.out.println("Please Enter Nick Name");
// read the next element
String nick = scanner.next();
System.out.println("Please Enter Cell Phone Number");
// read the next element
String number = scanner.next();
// create new BestFriend object and add to best object
BestFriend b = new BestFriend(first, last, nick, number); /* Note: This section of the code sorts the list out in alphabetical order, however this causes the order in which the names were inputted, to be lost. */ if (best.size() == 0) {
// adding as the first object
best.add(b);
} else if (b.getFirstName().compareTo(best.get(0).getFirstName()) < 0) {
/**
* Adding before current first object
*/
best.add(0, b);
} else {
// a flag to denote if the object is added or not
boolean added = false;
// looping through all objects
for (int i = 0; i < best.size() - 1; i++) {
/**
* checking if the object can be added to the middle of current
* pair of elements (between positions i and i+1)
*/
if (b.getFirstName().compareTo(best.get(i).getFirstName()) >= 0
&& b.getFirstName().compareTo(
best.get(i + 1).getFirstName()) < 0) {
best.add(i + 1, b);
// added to the middle
added = true;
break;
}
}
// finally, if not added, adding to end
if (!added) {
best.add(b);
}
}
}
public void change() {
// create Scanner object to get user input
Scanner scanner = new Scanner(System.in);
int AS = 0;
System.out.println("Enter First Name Of Friend You Want To Change");
// read the next element
String first = scanner.next();
System.out.println("Enter Last Name Of Friend You Want To Change");
// read the next element
String last = scanner.next();
// create an object of class bestfriend
BestFriend BF = new BestFriend(first, last, "", "");
// store the size
AS = best.size();
// if there is no element in BF
if (AS == 0) {
System.out.print("List is Empty");
return;
}
for (int i = 0; i < AS; i++) {
if (best.get(i).equals(BF)) {
System.out.println("Please Enter New First Name");
// read next element
first = scanner.next();
System.out.println("Please Enter New Last Name");
// read next element
last = scanner.next();
System.out.println("Please Enter New Nick Name");
// read next element
String nick = scanner.next();
System.out.println("Please Enter New Cell Phone Number");
// read next element
String number = scanner.next();
// update the fields of i th element in best
best.get(i).setFirstName(first);
best.get(i).setLastName(last);
best.get(i).setNickName(nick);
best.get(i).setCellPhoneNumber(number);
// break out of loop
break;
}
// if the element is not found
else if (i == AS - 1) {
System.out.println("No Such Friend Exists");
break;
}
}
}
public void remove() {
int AS = 0;
// create Scanner object to get user input
Scanner scanner = new Scanner(System.in);
System.out.println("Enter First Name Of Friend You Want To Remove");
// read next element
String first = scanner.next();
System.out.println("Enter Last Name Of Friend You Want To Remove");
// read next element
String last = scanner.next();
// create object of type BestFriend class
BestFriend BF = new BestFriend(first, last, "", "");
// store the size
AS = best.size();
// if there is no element in BF
if (AS == 0) {
System.out.println("List is Empty");
return;
}
for (int i = 0; i < AS; i++)
{
// if the current element is the required element to be removed
if (best.get(i).equals(BF)) {
// remove the element
best.remove(i);
// break out of loop
break;
}
// if the element is not found
else if (i == AS - 1) {
System.out.println("No Such Friend Exists");
// break out of loop
break;
}
}
}
public void display() {
int AS = best.size();
// if the list is empty
if (AS == 0) {
System.out.println("List is Empty");
return;
}
for (int i = 0; i < AS; i++) {
System.out.println(best.get(i).toString());
}
}
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
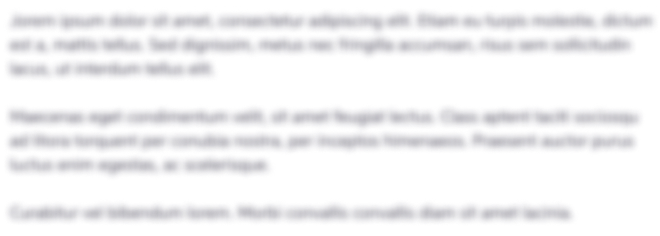
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started