Question
Class: Course Description: Here's a template for Course.java as well (attached image) . Complete the Course.java class. The methods and constructors you are to work
Class: Course Description: Here's a template for Course.java as well (attached image). Complete the Course.java class. The methods and constructors you are to work on are the following:
-
Course (constructor to initialize name and Faculty data fields)
-
addStudent( method to add a Student object to the roster data field)
-
getGPAs( method that returns an array of doubles whose elements are the GPA of each Student object in roster)
-
getStudents( method that returns an array of Strings that represent Student names, first and last )
-
getClassGrades( method that returns an array of integers whose elements are the grade for each Student object for this Course)
The toString method has been implemented for you, such that the following output is display when TestCourse.java is executed:
Sample Output: (Note that the Student objects to be added to the Course are randomized)
Student Name: Tara Downs Email: TDowns@somedomain.com Classes: [PHYS 200, ENGR 212, CS 231, MATH 194] Grades: [67, 97, 65, 88] Student Name: Dennis Ortiz Email: DOrtiz@somedomain.com Classes: [MATH 194, ENGR 212, CS 231, PHYS 200] Grades: [84, 87, 72, 92] Student Name: Olivia Little Email: OLittle@somedomain.com Classes: [ENGR 212, MATH 194, PHYS 200, CS 231] Grades: [72, 75, 100, 74] Student Name: Tara Dale Email: TDale@somedomain.com Classes: [ENGR 212, MATH 194, CS 231, PHYS 200] Grades: [81, 74, 60, 66] Student Name: Nicolas Reid Email: NReid@somedomain.com Classes: [ENGR 212, CS 231, PHYS 200, MATH 194] Grades: [89, 63, 92, 69] Student Name: Jason Donovan Email: JDonovan@somedomain.com Classes: [MATH 194, PHYS 200, ENGR 212, CS 231] Grades: [80, 95, 80, 60] Student Name: Trizten Reid Email: TReid@somedomain.com Classes: [PHYS 200, MATH 194, CS 231, ENGR 212] Grades: [76, 100, 95, 73] Student Name: Maria Cooks Email: MCooks@somedomain.com Classes: [PHYS 200, CS 231, MATH 194, ENGR 212] Grades: [73, 93, 96, 68] Student Name: Oscar Ortiz Email: OOrtiz@somedomain.com Classes: [CS 231, ENGR 212, MATH 194, PHYS 200] Grades: [83, 91, 61, 82] Student Name: John Lopez Email: JLopez@somedomain.com Classes: [CS 231, MATH 194, PHYS 200, ENGR 212] Grades: [61, 63, 72, 74] CS 231 Faculty: Octavio Ortiz MS in Aerospace Engineering Department: Math, Engineering and Comp Sci Name: Tara Downs Grade: 65 GPA: 2.250000 Name: Dennis Ortiz Grade: 72 GPA: 3.000000 Name: Olivia Little Grade: 74 GPA: 2.500000 Name: Tara Dale Grade: 60 GPA: 1.750000 Name: Nicolas Reid Grade: 63 GPA: 2.250000 Name: Jason Donovan Grade: 60 GPA: 2.750000 Name: Trizten Reid Grade: 95 GPA: 3.000000 Name: Maria Cooks Grade: 93 GPA: 2.750000 Name: Oscar Ortiz Grade: 83 GPA: 2.750000 Name: John Lopez Grade: 61 GPA: 1.500000
In the Sample Output, the grade each Student object has in CS 231 is printed at the end (from Course.java toString). It can be cross-referenced with each Student object's classes and grades array. That is, John Lopez has a 61 in CS 231. CS 231 is the first element in his classes array, thus the first element in his grades array is retrieved by getClassGrades() from Course.java.
Here is the template:
import java.util.ArrayList;public class Course_Template{ //Students (ArrayList) and one Faculty (1) private String name; private ArrayList
//Get names of Student objects in roster String[] studentNames = getStudents(); //Get Student objects' grades for this Course int[] classGrades = getClassGrades(); //Get Student objects' gpas double[] gpas = getGPAs(); //Add Faculty object to str String str = this.name + " " + this.teacher + " "; //Append every Student object in roster to str (Name: some name Grade: some grade GPA: some GPA) for( int i = 0; i < getStudents().length; i++ ){ str += String.format("Name: %-15s Grade: %3d GPA: %4f ", studentNames[i], classGrades[i], gpas[i] ); } //return String when Course object is referenced return str; }}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
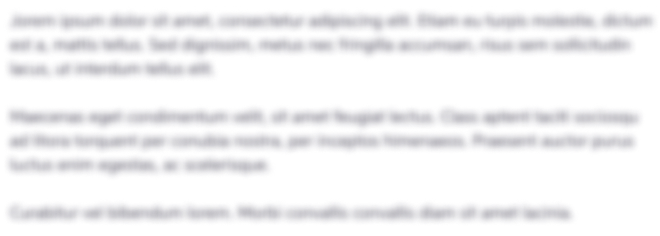
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started