Answered step by step
Verified Expert Solution
Question
1 Approved Answer
Complete the method sortByState() and sortByWhom2vote() package Apps; import ADTs.ListADT; import Algorithms.Search; import Algorithms.Sort; import DataStructures.SinglyLinkedList; import java.util.ArrayList; import java.util.Arrays; import java.util.Collection; import java.util.Collections; import
Complete the method sortByState() and sortByWhom2vote()
package Apps; import ADTs.ListADT; import Algorithms.Search; import Algorithms.Sort; import DataStructures.SinglyLinkedList; import java.util.ArrayList; import java.util.Arrays; import java.util.Collection; import java.util.Collections; import java.util.Comparator; /** * Vote management * @author ITSC 2214 Q * */ public class VoteMan { private ListADT votes; public VoteMan() { readVotes(); } // private helper method to initialize data private void readVotes() { votes = new SinglyLinkedList(); //(String name, String state, int ID, String who2Vote) votes.addFirst(new Vote("Jason Aldean", "NC", 1, "Peter Pan")); votes.addFirst(new Vote("Panic at the Disco", "NC", 2, "Harry Potter")); votes.addFirst(new Vote("Phish", "FL", 1, "Harry Potter")); votes.addFirst(new Vote("Carrie Underwood", "NC", 3, "Peter Pan")); votes.addFirst(new Vote("Bonnie Raitt", "SC", 1, "Peter Pan")); votes.addFirst(new Vote("Needtobreathe", "FL", 2, "Harry Potter")); votes.addFirst(new Vote("Dolly Parton", "SC", 2, "Harry Potter")); votes.addFirst(new Vote("Trans-Siberian Orchestra", "FL", 3, "Harry Potter")); votes.addFirst(new Vote("Twney One Pilots", "NC", 4, "Harry Potter")); } /** * Examine whether the given vote information can be found */ public void existVote(String state, int ID, String whom2vote) { Vote target = new Vote("", state, ID, whom2vote); int foundIndex = -1; try { foundIndex = Search.linearSearch(votes, target); } catch (Exception ex) { ex.getMessage(); } if (foundIndex != -1) { System.out.println("Linear Search. Found vote at the index "); System.out.println("t" + foundIndex); } else { System.out.println("Linear Search. Didn't find vote"); } } /** * Sorts the votes stored by this VoteMan by states, * ascending.Doesn't print or return anything. */ public void sortByState() { System.out.println("Selection sort by states: " + this.toString()); //TODO sort votes using selection sort based on voting states Sort.insertionSort(votes, Vote.Comparators.STATE); System.out.println("Insertion sort by states: " + this.toString()); Sort.mergeSort(votes, 0, votes.size() - 1, Vote.Comparators.STATE); System.out.println("Merge sort by states: " + this.toString()); } /** * Sorts the votes stored by this VoteMan by whom2vote */ public void sortByWhom2vote() { System.out.println("Selection sort by whom2vote: " + this.toString()); //TODO sort votes using selection sort based on whom to vote Sort.insertionSort(votes, Vote.Comparators.WHOM2VOTE); System.out.println("Insertion sort by whom2vote: " + this.toString()); Sort.mergeSort(votes, 0, votes.size() - 1, Vote.Comparators.WHOM2VOTE); System.out.println("Merge sort by whom2vote: " + this.toString()); } /** * Retrieve all vote information */ @Override public String toString() { //return Arrays.toString(concertTickets); String list = new String(); try { for (int i = 0; i < votes.size(); i++) { list = list.concat("t" + votes.get(i).toString() + "" ); } } catch (Exception ex){ ex.getMessage(); } return list; } public static void main(String argv[]){ VoteMan voteman = new VoteMan(); voteman.sortByState(); voteman.sortByWhom2vote(); voteman.existVote("FL", 1, "Harry Potter"); } }
Step by Step Solution
★★★★★
3.49 Rating (152 Votes )
There are 3 Steps involved in it
Step: 1
To complete the sortByState and sortByWhom2vote methods in the VoteMan class we need to utilize sort...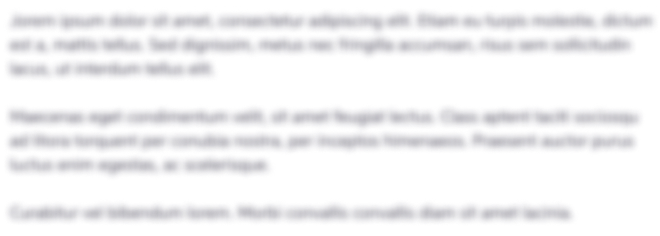
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started