Question
Consider the following (partial) class definition: public class Account { public final int accountNo; private double balance; public Account(double openingBalance) { ... } public double
Consider the following (partial) class definition:
public class Account { public final int accountNo; private double balance; public Account(double openingBalance) { ... } public double getBalance() { return balance; } public void deposit(double amount) { requirePositive(amount); balance += amount; } public void withdraw(double amount) { requirePositive(amount); requireSufficientBalance(amount); balance -= amount; } private static void requirePositive(double amt) { if (amt <= 0.0) { throw new IllegalArgumentException("Negative amount: " + amt); } } private void requireSufficientBalance(double amt) { if (amt > balance) { throw new InsufficientFundsException("Insufficient funds: " + amt); } } ... }
Write a new class, ChequingAccount, that extends Account by adding the following method:
- writeCheque(double amt)
The method tries to withdraw that amount, plus a small fee (let's say $0.50, for now). There must be enuf money in the account to cover the amount of the cheque AND the fee. For example, if the balance is $100, a cheque for $100 will bounce, because there needs to be $100.50 in the account to cover the cheque and its fee.
Write only the methods that are needed to make sure the ChequingAccount can be created (with its initial balance), cheques written, money can be deposited and withdrawn, and the balance returned.
For example, the following code:
ChequingAccount oldie = new ChequingAccount(100.00); // initial balance: 100 oldie.writeCheque(50.00); // balance: 49.50 (50 cent fee for cheques!) oldie.deposit(100.00); // balance: 149.50 oldie.withdraw(10.00); // balance: 139.50 System.out.println("Balance: $" + oldie.getBalance()); oldie.writeCheque(200.00); // insufficient funds!!!
Should produce this output:
Balance: $139.5
and then crash with an InsufficientFundsException.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
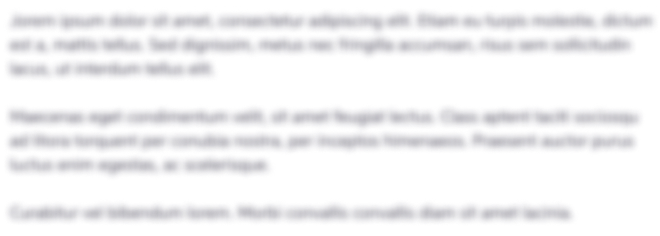
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started