Question
CPU Scheduling Assignment Overview One of the main tasks of an operating system is scheduling processes to run on the CPU. In this assignment, you
CPU Scheduling Assignment
Overview
One of the main tasks of an operating system is scheduling processes to run on the CPU. In this assignment, you will build a program which schedules simulated CPU processes. Your simulator program will implement three of the CPU scheduling algorithms discussed in this course. The simulator selects a process to run from the ready queue based on the scheduling algorithm chosen at runtime. Since the assignment intends to simulate a CPU scheduler, it does not require any actual process creation or execution. When the CPU scheduler chooses the next process, the simulator will simply print out which process was selected to run at that time. The simulator output is similar to the Gantt chart style.
Design
1.Write a class called Process which stores the ID, arrival time, and CPU burst length of a process, all are integers. You can also add data members to keep track of information in order to compute the statistics about the process such as its wait time, response time, and turnaround time. The methods of the Process class are the get and set methods for each data member, the constructor for the class, and any method needed to compute the statistics for that process.
2.Write a class called Scheduler to simulate a CPU scheduler for an operating system. The scheduler contains the ready queue and the ready queue is a circular linked list. Youll use the circular linked list you implemented for assignment 1. The only operations the scheduler performs is add and remove. The add operation adds a process into the ready queue into its appropriate spot within the ready queue according to the CPU scheduling algorithm implemented. The remove operation removes a process from the ready queue according to the CPU scheduling algorithm implemented. Youll implement the CPU scheduling algorithms: First Come First Serve, Shortest Remaining Time First which is the preemptive version of Shortest Job First, and Round Robin. Here is a perfect use of inheritance. If needed, you can add an additional method to the circular linked list class to help in the use of the ready queue for a particular CPU scheduling algorithm.
3.Create a driver class and make the name of the driver class Assignment2 and it should only contain only one method:
public static void main(String args[]).
The main method receives, via the command line arguments, the name of the CPU scheduler that your simulator program will execute. If Round Robin is the CPU scheduler chosen then the time quantum value is also received via a command line argument. The main method opens the file assignment2.txt reading in the entire set of processes and initiates execution of the simulator program. Assume there is only a single processor with only one core. The main method itself should be fairly short.
The command to launch your program using First Come First Serve scheduling: java Assignment2 FCFS
The command to launch your program using Shortest Remaining Time First scheduling: java Assignment2 SRTF
The command to launch your program using Round Robin scheduling with a time quantum of 10: java Assignment2 RR 10
4.The input to your program will be read from a plain text file called assignment2.txt. This is the statement youll use to open the file:
FileInputStream fstream = new FileInputStream("assignment2.txt");
Assuming youre using Eclipse to create your project, you will store the input file assignment2.txt in the parent directory of your source code (.java files) which happens to be the main directory of your project in Eclipse. If youre using some other development environment, you will have to figure out where to store the input file.
Each line in the file represents a process, 3 integers separated by spaces. The process information includes the process ID, arrival time, and CPU burst length. Arrival time is the time at which the scheduler receives the process and places it in the ready queue. You can assume arrival times of the processes in the input file are in non-decreasing order. Process IDs are unique. Arrival times may be duplicated, which means multiple processes may arrive at the same time. The following table is an example of a three process input file. The text in the top row of the table is just to label the value in each column and would not appear in the input file. Remember, the integers on each line are separated by a space or spaces.
Process ID | Arrival Time | CPU Burst Length |
1 | 0 | 10 |
2 | 0 | 20 |
3 | 3 | 5 |
5.For the output, the example below best describes what your program should produce. Your program will not be tested on this sample input but a different sample input.
Here is the example input:
1 0 10
2 0 9
3 3 5
4 7 4
5 10 6
6 10 7
Here is the output produced for the above example input given the command java Assignment2 FCFS to execute the program:
Scheduling algorithm: First Come First Serve ============================================================
| 0> process | 1 | is | running |
| 1> process | 1 | is | running |
| 2> process | 1 | is | running |
| 3> process | 1 | is | running |
| 4> process | 1 | is | running |
| 5> process | 1 | is | running |
| 6> process | 1 | is | running |
| 7> process | 1 | is | running |
| 8> process | 1 | is | running |
| 9> process | 1 | is | running |
| 10> process | 1 | is | finished.... |
| 10> process | 2 | is | running |
| 11> process | 2 | is | running |
| 12> process | 2 | is | running |
| 13> process | 2 | is | running |
| 14> process | 2 | is | running |
| 15> process | 2 | is | running |
| 16> process | 2 | is | running |
| 17> process | 2 | is | running |
| 18> process | 2 | is running |
| 19> process | 2 | is finished.... |
| 19> process | 3 | is running |
| 20> process | 3 | is running |
| 21> process | 3 | is running |
| 22> process | 3 | is running |
| 23> process | 3 | is running |
| 24> process | 3 | is finished.... |
| 24> process | 4 | is running |
| 25> process | 4 | is running |
| 26> process | 4 | is running |
| 27> process | 4 | is running |
| 28> process | 4 | is finished.... |
| 28> process | 5 | is running |
| 29> process | 5 | is running |
| 30> process | 5 | is running |
| 31> process | 5 | is running |
| 32> process | 5 | is running |
| 33> process | 5 | is running |
| 34> process | 5 | is finished.... |
| 34> process | 6 | is running |
| 35> process | 6 | is running |
| 36> process | 6 | is running |
| 37> process | 6 | is running |
| 38> process | 6 | is running |
| 39> process | 6 | is running |
| 40> process | 6 | is running |
| 41> process | 6 | is finished.... |
| 41> All processes finished...... |
===========================================================
Average CPU usage: | 100.00% |
Average waiting time: | 14.17 |
Average response time: | 14.17 |
Average turnaround time: | 21.00 |
============================================================
6.Tip: Make your program as modular as possible, not placing all your code in one .java file. You can create as many classes as you need in addition to the classes described above. Methods should be reasonably small following the guidance that "A function should do one thing, and do it well."
7.Do NOT use your own packages in your program. If you see the keyword package on the top line of any of your .java files then you created a package. Create every .java file in the src folder of your Eclipse project.
8.Do NOT use any graphical user interface code in your program!
|
|
|
|
|
|
|
|
|
|
|
|
Step by Step Solution
There are 3 Steps involved in it
Step: 1
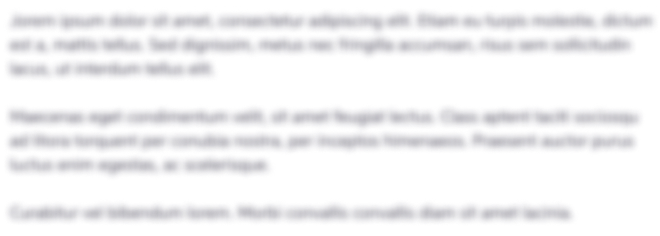
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started