Question
Create a program that reads in attendees' information, and create an event seating with a number of rows and columns specified by a user. Then
Create a program that reads in attendees' information, and create an event seating with a number of rows and columns specified by a user. Then it will attempt to assign each attendee to a seat in at the event. Using C++ Programming only.
Create a class Attendee. Create attendee.cpp and attendee.h files. It should contain two variables, lastName (char [30]) and firstName (char [30]). Both should be private. In addition, the following functions should be defined. All of them are public
Method | Description |
---|---|
Attendee ( ) | Constructs a Attendee object by assigning the default string " XXX " to both instance variables, firstName and lastName. |
Attendee (char* attendeeInfo) | Constructs a Attendee object using the string containing attendee's info. Use the strtok function to extract first name and last name, then assign them to each instance variable of the Attendee class. An example of the input string is: Charlie/Brown |
char* getFirstName ( ) | It should return the instance variable firstName. |
char* getLastName ( ) | It should return the instance variable lastName. |
char* toString ( ) | It should constructor a string containing the initial character of the firstName, a period, the initial character of the lastName, and a period, then it returns it. An example of such string for the guest Charlie Brown is: C.B. |
Create a class called Event. Create event.cpp and event.h files. The class EventSeating will contain a 2-dimensional array called "seating" of Attendee objects at its instance variable. The class Event must include the following constructor and methods.
Event (int rowNum, int columnNum) | It instantiates a two-dimensional array of the size "rowNum" by "columnNum" specified by the parameters. Then it initializes each attendee element of this array using the constructor of the class Attendee without any parameter. So, each guest will have default values for its instance variables. |
Attendee* getAttendeeAt (int row, int col) | Returns a attendee at the indexes row and col (specified by the parameters of this method) of the array "seating". |
bool assignAttendeetAt (int row, int col, Attendee *tempAttendee) | The method attempts to assign the "tempAttendee" to the seat at "row" and "col" (specified by the parameters of this method). If the seat has a default attendee, i.e., a attendee with the last name "XXX" and the first name "XXX", then we can assign the new guest "tempAttendee" to that seat and the method returns true. Otherwise, this seat is considered to be taken by someone else, the method does not assign the attendee and returns false. |
bool checkSeating (int row, int col) | Method checks if the parameters row and col are valid. If at least one of the parameters "row" or "col" is less than 0 or larger than the last index of the array (note that the number of rows and columns can be different), then it returns false. Otherwise it returns true. |
char* toString( ) | Returns a String containing information of the "seating". It should show the list of attendee assigned to the seating using the toString method of the class Attendee (it shows initials of each attendee) and the following format: The current seating -------------------- L.J. ?.?. B.T.. ?.?. ?.?. Q.W. T.H. E.T. ?.?. |
Code thus far:
#include guest.h #include event.h void main() { Event* event; Attendee* tempAttendee; int row, col, rowNum, columnNum; char attendeeInfo[30]; cout << "Please enter a number of rows for an event seating."; cin >> rowNum;
cout << "Please enter a number of columns for an event seating."; cin >> columnNum;
event = new Event(rowNum, columnNum); cout <<"Please enter a attendee information or enter \"Q\" to quit.";
cin >> attendeeInfo; while (1 /* fix this condition*/ ){ cout << " A attendee information is read."; // printing info cout << attendeeInfo; tempAttendee = new Attendee (attendeeInfo);
// Ask a user to decide where to seat a attendee
cout <<"Please enter a row number where the attendee wants to sit."; cin >> row; cout << "Please enter a column number where the attendee wants to sit."; cin >> col;
// Checking if the row number and column number are valid (exist in the event created.) if (*event.checkSeating(row, col) == false) { cout << " row or column number is not valid."; cout << "A attendee << (*tempAttendee).getFirstName() << << (*tempAttendee).getLastName() << is not assigned a seat."; } else { // Assigning a seat for a attendee if ((*event).assignAttendeeAt(row, col, tempAttendee) == true){ cout <<" The seat at row << row << and column << is assigned to the attendee, << (*tempAttendee).toString(); (*event).toString(); } else { cout <<" The seat at row << row << and column << col << is taken, sorry."; } } // Read the next attendeeInfo cout <<"Please enter a attendee information or enter \"Q\" to quit."; /*** reading a attendee information ***/ cin >>attendeeInfo; } }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
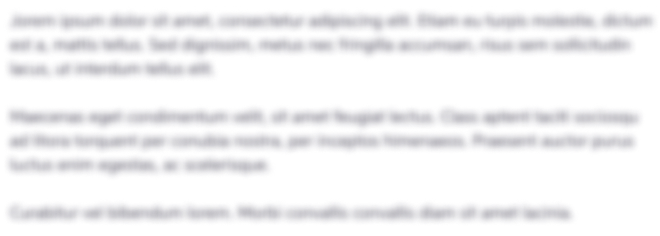
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started