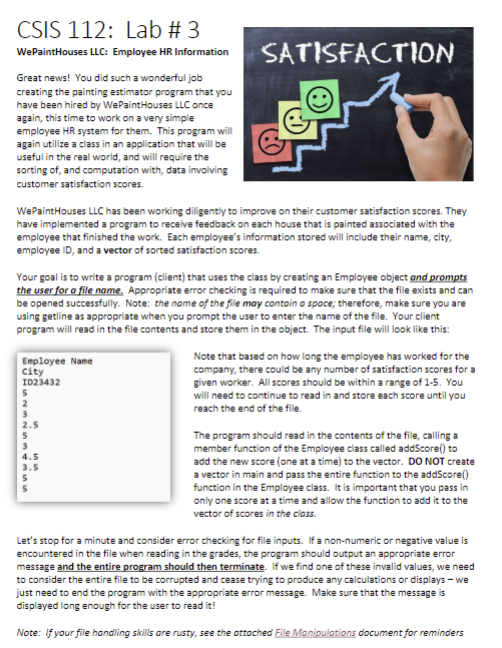
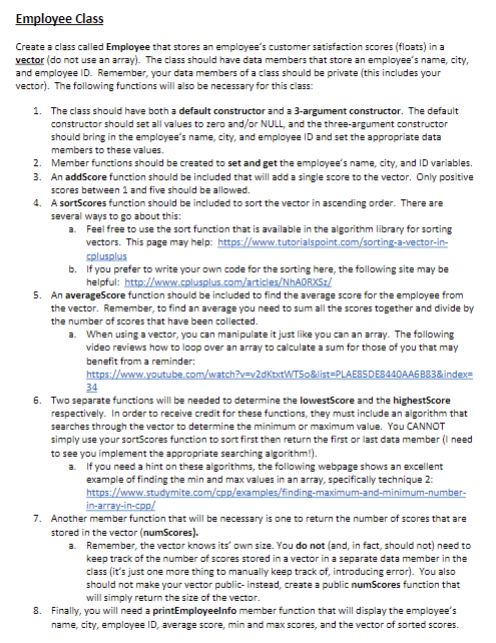
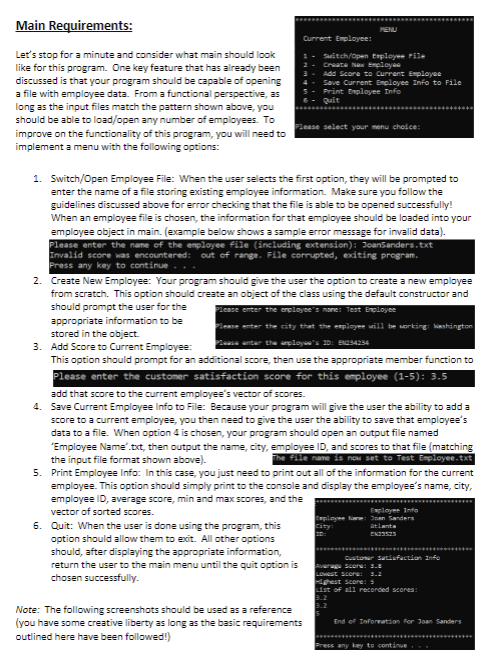
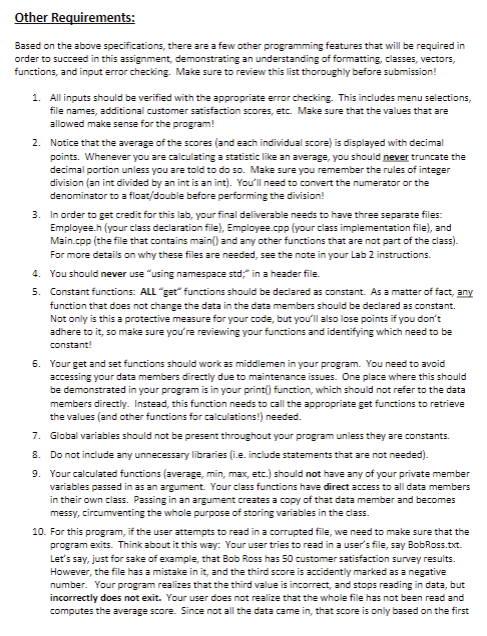
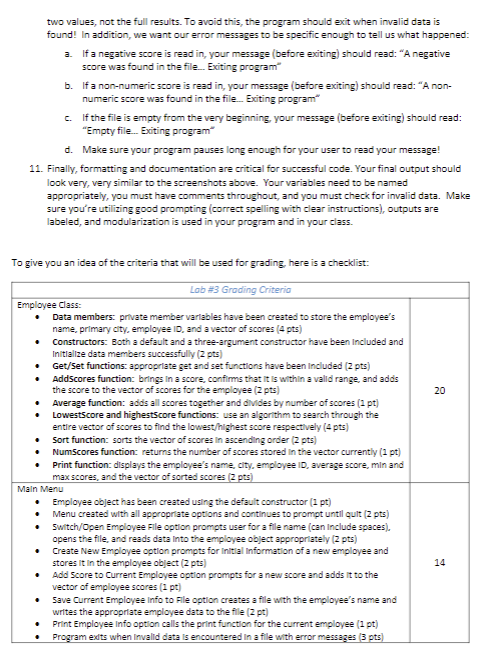
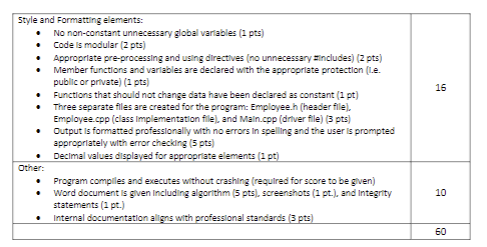
CSIS 112: Lab \# 3 WePaintHouses LLC: Employee HR Information Great news! You did such a wonderful job creating the painting estimator program that you have been hired by WePaintHouses LLC ance again, this time to work on a very simple employee HR system for them. This program will again utilize a class in an application that will be useful in the real world, and will require the sorting of, and computation with, data involving customer satisfaction scores. WePaintHouses LLC has been working diligently to improve on their customer satisfaction scores. They have implemented a program to receive feedback on each house that is painted associated with the employee that finished the work. Each employee's information stored will include their name, city. employee ID, and a vector of sorted satisfaction scores. Your goal is to write a program (client) that uses the class by creating an Employee object and prompts the user for a file name. Appropriate error checking is required to make sure that the file exists and can be opened successfully. Note: the name of the file moy contoin o spoce; therefore, make sure you are using getline as appropriate when you prompt the user to enter the name of the file. Your client program will read in the file contents and store them in the object. The input file will look like this: Note that based on how long the employee has worked for the company, there could be any number of satisfaction scores for a given worker. All scores should be within a range of 1-5. You will need to continue to read in and store each score until you reach the end of the file. The program should read in the contents of the file, calling a member function of the Employee class called addScore() to add the new score (one at a time) to the vector. DO NOT create a vector in main and pass the entire function to the addScore() function in the Employee class. It is important that you pass in only one score at a time and allow the function to add it to the vector of scores in the class. Let's stop for a minute and consider error checking for file inputs. If a non-numeric or negative value is encountered in the file when reading in the grades, the program should output an appropriate error message and the entire program should then terminate. If we find one of these invalid values, we need to consider the entire file to be corrupted and cease trying to produce any calculations or displays - we just need to end the program with the appropriate error message. Make sure that the message is displayed long enough for the user to read it! Note: If your file hondling skills are rusty, see the attoched File Manipulotions document for reminders Create a class called Employee that stores an employee's customer satisfaction scores (floats) in a vector (do not use an array). The class should have data members that store an employee's name, city, and employee ID. Remember, your data members of a class should be private (this includes your vector). The following functions will also be necessary for this class: 1. The class should have both a default constructor and a 3-argument constructor. The default constructor should set all values to zero and/or NULL, and the three-argument constructor should bring in the employee's name, city, and employee ID and set the appropriate data members to these values. 2. Member functions should be created to set and get the employee's name, city, and ID variables. 3. An addScore function should be included that will add a single score to the vector. Only positive scores between 1 and five should be allowed. 4. A sortScores function should be included to sort the vector in ascending order. There are several ways to go about this: a. Feel free to use the sort function that is available in the algorithm library for sorting vectors. This page may help: https://www.tutorialspoint.com/sorting-a-vector-insplusplus b. If you prefer to write your own code for the sorting here, the following site may be helpful: http// www. cplusplus. com/articles/NhAORXSz/ 5. An averageScore function should be included to find the average score for the employee from the vector. Remember, to find an average you need to sum all the scores together and divide by the number of scores that have been collected. a. When using a vector, you can manipulate it just like you can an array. The following video reviews how to loop over an array to calculate a sum for those of you that may benefit from a reminder: https://www.youtube com/watch?vev2dKtotWTSo\&list=PLAE85DES440AA6B838index= 34 6. Two separate functions will be needed to determine the lowestScore and the highestScore respectively. In order to receive credit for these functions, they must include an algorithm that searches through the vector to determine the minimum or maximum value. You CANNOT simply use your sortScores function to sort first then return the first or last data member (I need to see you implement the appropriate searching aigorithm!). a. If you need a hint on these algorithms, the following webpage shows an excellent example of finding the min and max values in an array, specifically technique 2 : https://www.studymite.com/cpp/examples/finding-maximum-and-minimum-numberin-array-in-cppl 7. Another member function that will be necessary is one to return the number of scores that are stored in the vector (numScores). a. Remember, the vector knows its' own size. You do not (and, in fact, should not) need to keep track of the number of scores stored in a vector in a separate data member in the class (it's just one more thing to manually keep track of, introducing error). You also should not make your vector public-instead, create a public numScores function that will simply return the size of the vector. 8. Finally, you will need a printEmployeelnfo member function that will display the employee's Main Requirements: Let's stop for a minute and consider what main should look like for this program. One key feature that has already been discussed is that your program should be capable of opening a file with employee data. From a functional perspective, as long as the input files match the pattern shown above, you should be able to load/open any number of employees. To improve on the functionality of this program, you will need to implement a menu with the following options: 1. Switch/Open Employee File: When the user selects the first option, they will be prompted to enter the name of a file storing existing employee information. Make sure you follow the guidelines discussed above for error checking that the file is able to be opened successfully! When an employee file is chosen, the information for that employee should be loaded into your employee object in main. (example below shows a sample error message for invalid data). Please enter the nane of the enployee file (iineluding extension); Jeansanders, txt Invallid score was encountered: out of range. Fille corrupted, exdting progran. Press any key to continue .... 2. Create New Employee: Your program should give the user the option to create a new employee from scratch. This option should create an object of the class using the default constructor and should prompt the user for the appropriate information to be stored in the object. Please enten the arployse'z nane: Test Etployee Add Score to Current Emplayee: This option should prompt for an additional score, then use the appropriate member function to Please enter the customer satisfaction score for this employee (1-5): 3.5 add that score to the current employee's vector of scores. 4. Save Current Employee Info to File: Because your program will give the user the ability to add a score to a current employee, you then need to give the user the ability to save that employee's data to a file. When option 4 is chosen, your program should open an output file named 'Employee Name'.txt, then output the name, city, employee ID, and scores to that file (matching the input file format shown above). 5. Print Employee Info: In this case, you just need to print out all of the information for the current employee. This option should simply print to the console and display the employee's name, city, employee ID, average score, min and max scores, and the vector of sorted scores. 6. Quit: When the user is done using the program, this option should allow them to exit. All other options should, after displaying the appropriate information, return the user to the main menu until the quit option is chosen successtully. Note: The following screenshots should be used as a reference (you have some creative liberty as long as the basic requirements outlined here have been followed!) Other Requirements: Based on the above specifications, there are a few other programming features that will be required in order to succeed in this assignment, demonstrating an understanding of formatting, classes, vectors, functions, and input error checking. Make sure to review this list thoroughly before submission! 1. All inputs should be verified with the appropriate error checking. This includes menu selections, file names, additional customer satisfaction scores, etc. Make sure that the values that are allowed make sense for the program! 2. Notice that the average of the scores (and each individual score) is displayed with decimal points. Whenever you are calculating a statistic like an average, you should never truncate the decimal portion unless you are told to do so. Make sure you remember the rules of integer division (an int divided by an int is an int). You'll need to convert the numerator or the denominator to a float/double before performing the division! 3. In order to get credit for this lab, your final deliverable needs to have three separate files: Employee.h (your class declaration file). Employee.cpp (your class implementation file), and Main.cpp (the file that contains main( () and any other functions that are not part of the class). For more details on why these files are needed, see the note in your Lab 2 instructions. 4. You should never use "using namespace std," in a header file. 5. Constant functions: ALL "get" functions should be declared as constant. As a matter of fact, any function that does not change the data in the data members should be declared as constant. Not only is this a protective measure for your code, but you'll also lose points if you don't adhere to it, so make sure you're reviewing your functions and identifying which need to be constant! 6. Your get and set functions should work as middlemen in your program. You need to avoid accessing your data members directly due to maintenance issues. One place where this should be demonstrated in your program is in your print(]) function, which should not refer to the data members directly. Instead, this function needs to call the appropriate get functions to retrieve the values (and other functions for calculations!) needed. 7. Global variables should not be present throughout your program unless they are constants. 8. Do not include any unnecessary libraries (i.e. include statements that are not needed). 9. Your calculated functions (average, min, max, etc.) should not have any of your private member variables passed in as an argument. Your class functions have direct access to all data members in their own class. Passing in an argument creates a copy of that data member and becomes messy, circumventing the whole purpose of storing variables in the class. 10. For this program, if the user attempts to read in a corrupted file, we need to make sure that the program exits. Think about it this way: Your user tries to read in a user's file, say BobRoss.txt. Let's say, just for sake of example, that Bob Ross has 50 customer satisfaction survey results. However, the file has a mistake in it, and the third score is accidently marked as a negative number. Your program realizes that the third value is incorrect, and stops reading in data, but incorrectly does not exit. Your user does not realize that the whole file has not been read and computes the average score. Since not all the data came in, that score is only based on the first two values, not the full results. To avoid this, the program should exit when invalid data is found! In addition, we want our error messages to be specific enough to tell us what happened: a. If a negative score is read in, your message (before exiting) should read: "A negative score was found in the file. Exiting program" b. If a non-numeric score is read in, your message (before exiting) should read: "A nonnumeric score was found in the file. Exiting program" c. If the file is empty from the very beginning, your message (before exiting) should read: "Empty file... Exiting program" d. Make sure your program pauses long enough for your user to read your message! 11. Finally, formatting and documentation are critical for successful code. Your final output should look very, very similar to the screenshots above. Your variables need to be named appropriately, you must have comments throughout, and you must check for invalid data. Make sure you're utilizing good prompting (correct spelling with clear instructions), outputs are labeled, and modularization is used in your program and in your class. To give you an idea of the criteria that will be used for grading, here is a checklist: 5tyle and Formatting elements: - No non-constant unnecessary global varlables (1 pts) - Code ls modular ( 2 pts) - Approprlate pre-processing and using diractives (no unnecessary includes) (2 pts) - Member functions and varlables are declared with the approprlate protection (1.e. public or private) (1 pts) - Functions that should not change data have been declared as constant ( 1 pt) - Three separate files are created for the programs Employee.h (header file). Employee.cpp (class Implementation file), and Main.cpp (driver fle) (3 pts) - Output is formatted professionally with no errors in spelling and the user ls prompted approprlately with error checking ( 5 pts) - Decimal values displayed for appropriate elements (1 pt) Other: - Program complles and executes without crashing (requlred for score to be given) - Word document is given Including algorithm (5 pts), screenshots (1 pt), and lntegrity 10 statements (1 pt.) - Internal documentation aligns with professlonal standards (3 pts) CSIS 112: Lab \# 3 WePaintHouses LLC: Employee HR Information Great news! You did such a wonderful job creating the painting estimator program that you have been hired by WePaintHouses LLC ance again, this time to work on a very simple employee HR system for them. This program will again utilize a class in an application that will be useful in the real world, and will require the sorting of, and computation with, data involving customer satisfaction scores. WePaintHouses LLC has been working diligently to improve on their customer satisfaction scores. They have implemented a program to receive feedback on each house that is painted associated with the employee that finished the work. Each employee's information stored will include their name, city. employee ID, and a vector of sorted satisfaction scores. Your goal is to write a program (client) that uses the class by creating an Employee object and prompts the user for a file name. Appropriate error checking is required to make sure that the file exists and can be opened successfully. Note: the name of the file moy contoin o spoce; therefore, make sure you are using getline as appropriate when you prompt the user to enter the name of the file. Your client program will read in the file contents and store them in the object. The input file will look like this: Note that based on how long the employee has worked for the company, there could be any number of satisfaction scores for a given worker. All scores should be within a range of 1-5. You will need to continue to read in and store each score until you reach the end of the file. The program should read in the contents of the file, calling a member function of the Employee class called addScore() to add the new score (one at a time) to the vector. DO NOT create a vector in main and pass the entire function to the addScore() function in the Employee class. It is important that you pass in only one score at a time and allow the function to add it to the vector of scores in the class. Let's stop for a minute and consider error checking for file inputs. If a non-numeric or negative value is encountered in the file when reading in the grades, the program should output an appropriate error message and the entire program should then terminate. If we find one of these invalid values, we need to consider the entire file to be corrupted and cease trying to produce any calculations or displays - we just need to end the program with the appropriate error message. Make sure that the message is displayed long enough for the user to read it! Note: If your file hondling skills are rusty, see the attoched File Manipulotions document for reminders Create a class called Employee that stores an employee's customer satisfaction scores (floats) in a vector (do not use an array). The class should have data members that store an employee's name, city, and employee ID. Remember, your data members of a class should be private (this includes your vector). The following functions will also be necessary for this class: 1. The class should have both a default constructor and a 3-argument constructor. The default constructor should set all values to zero and/or NULL, and the three-argument constructor should bring in the employee's name, city, and employee ID and set the appropriate data members to these values. 2. Member functions should be created to set and get the employee's name, city, and ID variables. 3. An addScore function should be included that will add a single score to the vector. Only positive scores between 1 and five should be allowed. 4. A sortScores function should be included to sort the vector in ascending order. There are several ways to go about this: a. Feel free to use the sort function that is available in the algorithm library for sorting vectors. This page may help: https://www.tutorialspoint.com/sorting-a-vector-insplusplus b. If you prefer to write your own code for the sorting here, the following site may be helpful: http// www. cplusplus. com/articles/NhAORXSz/ 5. An averageScore function should be included to find the average score for the employee from the vector. Remember, to find an average you need to sum all the scores together and divide by the number of scores that have been collected. a. When using a vector, you can manipulate it just like you can an array. The following video reviews how to loop over an array to calculate a sum for those of you that may benefit from a reminder: https://www.youtube com/watch?vev2dKtotWTSo\&list=PLAE85DES440AA6B838index= 34 6. Two separate functions will be needed to determine the lowestScore and the highestScore respectively. In order to receive credit for these functions, they must include an algorithm that searches through the vector to determine the minimum or maximum value. You CANNOT simply use your sortScores function to sort first then return the first or last data member (I need to see you implement the appropriate searching aigorithm!). a. If you need a hint on these algorithms, the following webpage shows an excellent example of finding the min and max values in an array, specifically technique 2 : https://www.studymite.com/cpp/examples/finding-maximum-and-minimum-numberin-array-in-cppl 7. Another member function that will be necessary is one to return the number of scores that are stored in the vector (numScores). a. Remember, the vector knows its' own size. You do not (and, in fact, should not) need to keep track of the number of scores stored in a vector in a separate data member in the class (it's just one more thing to manually keep track of, introducing error). You also should not make your vector public-instead, create a public numScores function that will simply return the size of the vector. 8. Finally, you will need a printEmployeelnfo member function that will display the employee's Main Requirements: Let's stop for a minute and consider what main should look like for this program. One key feature that has already been discussed is that your program should be capable of opening a file with employee data. From a functional perspective, as long as the input files match the pattern shown above, you should be able to load/open any number of employees. To improve on the functionality of this program, you will need to implement a menu with the following options: 1. Switch/Open Employee File: When the user selects the first option, they will be prompted to enter the name of a file storing existing employee information. Make sure you follow the guidelines discussed above for error checking that the file is able to be opened successfully! When an employee file is chosen, the information for that employee should be loaded into your employee object in main. (example below shows a sample error message for invalid data). Please enter the nane of the enployee file (iineluding extension); Jeansanders, txt Invallid score was encountered: out of range. Fille corrupted, exdting progran. Press any key to continue .... 2. Create New Employee: Your program should give the user the option to create a new employee from scratch. This option should create an object of the class using the default constructor and should prompt the user for the appropriate information to be stored in the object. Please enten the arployse'z nane: Test Etployee Add Score to Current Emplayee: This option should prompt for an additional score, then use the appropriate member function to Please enter the customer satisfaction score for this employee (1-5): 3.5 add that score to the current employee's vector of scores. 4. Save Current Employee Info to File: Because your program will give the user the ability to add a score to a current employee, you then need to give the user the ability to save that employee's data to a file. When option 4 is chosen, your program should open an output file named 'Employee Name'.txt, then output the name, city, employee ID, and scores to that file (matching the input file format shown above). 5. Print Employee Info: In this case, you just need to print out all of the information for the current employee. This option should simply print to the console and display the employee's name, city, employee ID, average score, min and max scores, and the vector of sorted scores. 6. Quit: When the user is done using the program, this option should allow them to exit. All other options should, after displaying the appropriate information, return the user to the main menu until the quit option is chosen successtully. Note: The following screenshots should be used as a reference (you have some creative liberty as long as the basic requirements outlined here have been followed!) Other Requirements: Based on the above specifications, there are a few other programming features that will be required in order to succeed in this assignment, demonstrating an understanding of formatting, classes, vectors, functions, and input error checking. Make sure to review this list thoroughly before submission! 1. All inputs should be verified with the appropriate error checking. This includes menu selections, file names, additional customer satisfaction scores, etc. Make sure that the values that are allowed make sense for the program! 2. Notice that the average of the scores (and each individual score) is displayed with decimal points. Whenever you are calculating a statistic like an average, you should never truncate the decimal portion unless you are told to do so. Make sure you remember the rules of integer division (an int divided by an int is an int). You'll need to convert the numerator or the denominator to a float/double before performing the division! 3. In order to get credit for this lab, your final deliverable needs to have three separate files: Employee.h (your class declaration file). Employee.cpp (your class implementation file), and Main.cpp (the file that contains main( () and any other functions that are not part of the class). For more details on why these files are needed, see the note in your Lab 2 instructions. 4. You should never use "using namespace std," in a header file. 5. Constant functions: ALL "get" functions should be declared as constant. As a matter of fact, any function that does not change the data in the data members should be declared as constant. Not only is this a protective measure for your code, but you'll also lose points if you don't adhere to it, so make sure you're reviewing your functions and identifying which need to be constant! 6. Your get and set functions should work as middlemen in your program. You need to avoid accessing your data members directly due to maintenance issues. One place where this should be demonstrated in your program is in your print(]) function, which should not refer to the data members directly. Instead, this function needs to call the appropriate get functions to retrieve the values (and other functions for calculations!) needed. 7. Global variables should not be present throughout your program unless they are constants. 8. Do not include any unnecessary libraries (i.e. include statements that are not needed). 9. Your calculated functions (average, min, max, etc.) should not have any of your private member variables passed in as an argument. Your class functions have direct access to all data members in their own class. Passing in an argument creates a copy of that data member and becomes messy, circumventing the whole purpose of storing variables in the class. 10. For this program, if the user attempts to read in a corrupted file, we need to make sure that the program exits. Think about it this way: Your user tries to read in a user's file, say BobRoss.txt. Let's say, just for sake of example, that Bob Ross has 50 customer satisfaction survey results. However, the file has a mistake in it, and the third score is accidently marked as a negative number. Your program realizes that the third value is incorrect, and stops reading in data, but incorrectly does not exit. Your user does not realize that the whole file has not been read and computes the average score. Since not all the data came in, that score is only based on the first two values, not the full results. To avoid this, the program should exit when invalid data is found! In addition, we want our error messages to be specific enough to tell us what happened: a. If a negative score is read in, your message (before exiting) should read: "A negative score was found in the file. Exiting program" b. If a non-numeric score is read in, your message (before exiting) should read: "A nonnumeric score was found in the file. Exiting program" c. If the file is empty from the very beginning, your message (before exiting) should read: "Empty file... Exiting program" d. Make sure your program pauses long enough for your user to read your message! 11. Finally, formatting and documentation are critical for successful code. Your final output should look very, very similar to the screenshots above. Your variables need to be named appropriately, you must have comments throughout, and you must check for invalid data. Make sure you're utilizing good prompting (correct spelling with clear instructions), outputs are labeled, and modularization is used in your program and in your class. To give you an idea of the criteria that will be used for grading, here is a checklist: 5tyle and Formatting elements: - No non-constant unnecessary global varlables (1 pts) - Code ls modular ( 2 pts) - Approprlate pre-processing and using diractives (no unnecessary includes) (2 pts) - Member functions and varlables are declared with the approprlate protection (1.e. public or private) (1 pts) - Functions that should not change data have been declared as constant ( 1 pt) - Three separate files are created for the programs Employee.h (header file). Employee.cpp (class Implementation file), and Main.cpp (driver fle) (3 pts) - Output is formatted professionally with no errors in spelling and the user ls prompted approprlately with error checking ( 5 pts) - Decimal values displayed for appropriate elements (1 pt) Other: - Program complles and executes without crashing (requlred for score to be given) - Word document is given Including algorithm (5 pts), screenshots (1 pt), and lntegrity 10 statements (1 pt.) - Internal documentation aligns with professlonal standards (3 pts)