Question
// /////////// // Employee.java public class Employee { private int empId; private String empName; private double hourlyWage; private int startYear; public Employee(int empId, String empName,
//
///////////
// Employee.java
public class Employee { private int empId; private String empName; private double hourlyWage; private int startYear; public Employee(int empId, String empName, double hourlyWage, int startYear) { this.empId = empId; this.empName = empName; this.hourlyWage = hourlyWage; this.startYear = startYear; } /**Accessors and mutators*/ public int getEmpId(){ return empId; } public String getEmpName(){ return empName; } public void setEmpName(String empName){ this.empName = empName; } public double getHourlyWage(){ return hourlyWage; } public void setHourlyWage(double hourlyWage){ this.hourlyWage = hourlyWage; } public int getStartYear(){ return startYear; } public void setStartYear(int startYear){ this.startYear = startYear; } public String toString(){ String output = empName+" - "+empId+" ("+hourlyWage+")"; return output; } }
//
// Project.java
import java.util.ArrayList; public class Project { /*Data members of a Project - Add the necessary data members*/ private String projectName; private String projectId; private int duration; public Project(){ } /**Accessor methods - no additional accessor methods needed*/ public String getProjectName(){ return projectName; } public String getProjectId(){ return projectId; } public Employee getProjectManager(){ return projectManager; } public int getDuration(){ return duration; } /*********MUTATOR METHODS - ADD THE NECESSARY MUTATORS ****************/ public void setDuration(int duration){ this.duration = duration; } /**removeTeamMember() method removes the given team member from the project*/ public boolean removeTeamMember(Employee emp){ return projectTeam.remove(emp); } /* containsTeamMember() method returns true the Project contains the given team member*/ public boolean containsTeamMember(Employee emp){ return projectTeam.contains(emp); } /*addTeamMember(): adds a team member to the project if does not exist*/ /**estimateProjectCost(): Estimates the human resource cost of a project based on duration*/ /**Returns a String representation of a Project*/ public String toString(){ String project = projectName+"["+projectId+"] "+" "+ "Team Lead: "+projectManager+" "+ "Estimated Cost: "+estimateProjectCost()+" "+ "Team: "; for(Employee emp : projectTeam){ project+="\t"+emp+" "; } return project; } }
// ProjectManagement.java
/** * @(#)ProjectManagement.java */ import java.util.*; import java.io.*;
public class ProjectManagement { //add data members here public ProjectManagement() throws IOException{ } public void run() throws IOException{ //display menu Scanner input = new Scanner(System.in); int choice; System.out.print("1 - Add Project "+ "2 - Assign Employee "+ "3 - Find Employee Project "+ "4 - Display Projects "+ "5 - EXIT "); System.out.print("Enter choice: "); choice = input.nextInt(); while(choice != 5){ switch(choice){ /*******IMPLEMENT SWITCH HERE*******/ } //get next choice System.out.print("1 - Add Project "+ "2 - Assign Employee "+ "3 - Find Employee Project "+ "4 - Display Projects "+ "5 - EXIT "); System.out.print("Enter choice: "); choice = input.nextInt(); } System.out.println("Goodbye...."); } private void loadEmployees() throws IOException{ Scanner inFile = new Scanner(new File("employees.txt")); inFile.useDelimiter(";"); inFile.useLocale(Locale.US); while(inFile.hasNext()){ int id = inFile.nextInt(); String name = inFile.next(); double wage = inFile.nextDouble(); int startYear = inFile.nextInt(); //add new Employee to the list employeeList[empCount] = new Employee(id,name,wage,startYear); empCount++; } inFile.close(); } /**RECURSIVE METHOD that Takes an employee id as parameter and returns the Employee with given id*/ public Employee searchEmployee(int id, int n){ } /**addProject() inputs project data and adds new project to the list*/ public void addProject(){ } /**Searches list of projects to find project with given team member (Employee)*/ public Project findProjectAssignment(){ } /**Assigns employee with input id to project with given project id*/ public void assignEmployee(){ } }
///
/** * @(#)ProjectManagementApp.java * * * @author * @version 1.00 2016/4/15 */ import java.io.*; public class ProjectManagementApp { public static void main(String[] args) throws IOException { ProjectManagement app = new ProjectManagement(); app.run(); } }
Write a java program that uses 4 classes, Employee, Project, ProjectManagement, ProjectManagementApp. The classes are described below. You should name all data members and methods as shown below CLASS: Employee Download this class from Unilica and examine the data members and methods. the same) and false if not). Signature: public boolean equals(Object obi)) .Add an equals() method that compares two employee objects and returns true if they are equal (id is CLASS: Project Download the class from Unilica. The class already contains some data members and methods. Carefully review the code provided to you and complete the class by adding the following: . Data Members o o o static int projectCount- starts at 100, increments when project is created Employee projectManager ArrayList projectTeam (Employees) Methods o Constructor Takes projectName and duration as parameters. Initializes the data members to the parameters. Initializes an empty projectTeam. Sets the projectld and projectManager using the set method. " o Mutators for the following data members public setProjectManager - sets projectManager to Employee passed as parameter " private setProjectld sets the project id to "2017 - projectCount. Ex if projectCount is 120, the projectld is "2017-120". Should increment the projectCount after setting o addTeamMember0 " Takes an Employee as a parameter "If the Employee does not exist in the projectTeam, add the Employee (use the containsTeamMember method in your solution. Returns true if added successfully, false if not. " o estimateProjectCost0 Project cost is found by multiplying the average hourly wage for all Employees in the projectTeam by the duration (in hours) of the project
Step by Step Solution
There are 3 Steps involved in it
Step: 1
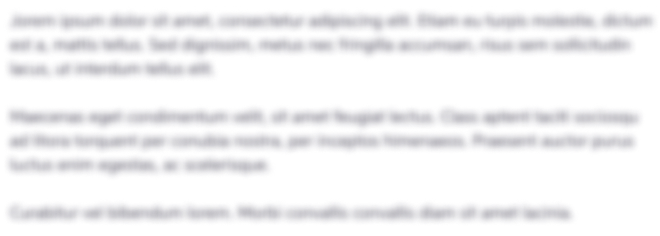
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started