Question
For CashRegister.java /** * A cash register totals up sales and computes change due. */ public class CashRegister { private double purchase; private double payment;
For CashRegister.java
/**
* A cash register totals up sales and computes change due.
*/
public class CashRegister {
private double purchase;
private double payment;
public static void main(String[] args) {
CashRegister customer1 = new CashRegister();
CashRegister customer2 = new CashRegister();
//Customer 1 - Payment information
customer1.enterPayment(80);
customer1.recordPurchase(49.95 + 20.40);
customer1.printReceipt();
//Customer 2 - Payment information
customer2.enterPayment(180);
customer2.recordPurchase(99.95 + 35.15 + 50.00);
customer2.printReceipt();
}
/**
* Constructs a cash register with no money in it.
*/
public CashRegister() {
purchase = 0;
payment = 0;
}
/**
* Records the sale of an item.
* @param amount the price of the item
*/
public void recordPurchase(double amount) {
double total = purchase + amount;
purchase = total;
}
/**
* Enters the payment received from the customer.
* @param amount the amount of the payment
*/
public void enterPayment(double amount) {
payment = amount;
}
/**
* Computes the change due and resets the machine for the next customer, and
* Print a receipt.
*/
public void printReceipt() {
double change = payment - purchase;
System.out.printf(" Total Sales: $ %.2f ", purchase);
System.out.printf(" Payment: $ %.2f ", payment);
System.out.printf("========================== ");
if (payment
System.out.printf("Not Enough Payment: $ %.2f ", change);
}
else {
purchase = 0;
payment = 0;
System.out.printf(" Change Due: $ %.2f ", change);
}
/*
* Write Java statement to record following two transaction into
* main method of RunCashRegister class:
*/
}
}
For RunCashRegister.java
public class RunCashRegister {
public static void main(String[] args) { CashRegister customer1 = new CashRegister(); CashRegister customer2 = new CashRegister(); //Customer 1 - Payment information customer1.enterPayment(80); customer1.recordPurchase(49.95 + 20.40); customer1.printReceipt(); //Customer 2 - Payment information customer2.enterPayment(180); customer2.recordPurchase(99.95 + 35.15 + 50.00); customer2.printReceipt(); } }
For BankAccount.java
/**
* A bank account has a balance that can be changed by
* deposits and withdrawals.
*/
public class BankAccount
{
//Instance variable
private double balance;
private String accountName;
/**
* Constructs a bank account with a zero balance
* and with an account name
* @param name the account name
*/
private static void main(String[] args) {
BankAccount customer = new BankAccount("Jen's Checking", 500);
// Write Java main statement method of BankTransaction class:
customer.getBalance();
customer.withdraw(50);
customer.transfer(BankTransaction, 500);
customer.printLineA();
customer.printBalance();
public BankAccount(String name)
{
balance = 0;
accountName = name;
}
/**
* Constructs a bank account with a given balance
* @param name the account name
* @param initialBalance the initial balance
*/
public BankAccount(String name, double initialBalance)
{
balance = initialBalance;
accountName = name;
}
/**
* Deposits money into the bank account.
* @param amount the amount to deposit
*/
public void deposit(double amount)
{
double newBalance = balance + amount;
balance = newBalance;
}
/**
* Withdraws money from the bank account.
* @param amount the amount to withdraw
*/
public void withdraw(double amount)
{
double newBalance = balance - amount;
balance = newBalance;
}
/**
* Gets the current balance of the bank account.
* @return the current balance
*/
public double getBalance()
{
return balance;
}
/**
* Transfer money from other account
* @param ba another BankAccount object
* @param amt the amount to transfer
*/
public void transfer(BankAccount ba, double amt) {
ba.withdraw(amt);
deposit(amt);
}
/**
* Print the balance
*/
public void printBalance() {
System.out.printf(accountName + " Account Balance is $ %.2f ", balance);
}
/**
* Print a line of "="s
*/
public void printLineA() {
System.out.println("==========================================");
}
/**
* Print a line of "&"s
*/
public void printLineB() {
System.out.println("&&&&&&&&&&&&&&&&&&&&&&&&&&&&&&&&&&&&&&&&&&&");
}
}
For BankTransactions.java
public class BankTransactions { private double balance; private String accountName; public static void main(String[] args) { BankTransactions customer = new BankTransactions("Jim's Saving", 0); BankTransactions customer2 = new BankTransactions("Jim's Checking", 0); // Write Java main statement method of BankTransaction class // Jim's Saving account customer.deposit(3000); customer.getBalance(); customer.printLineA(); customer.printBalance(); //Jim's Checking account customer2.getBalance(); customer2.transfer(customer); customer2.withdraw(500); customer2.getBalance(); customer.getBalance(); customer.printLineA(); } public BankTransactions(String name) { balance = 0; accountName = name; }
/** * Constructs a bank account with a given balance * @param name the account name * @param initialBalance the initial balance */ public BankTransactions(String name, double initialBalance) { balance = initialBalance; accountName = name; }
/** * Deposits money into the bank account. * @param amount the amount to deposit */ public void deposit(double amount) { double newBalance = balance + amount; balance = newBalance; }
/** * Withdraws money from the bank account. * @param amount the amount to withdraw */ public void withdraw(double amount) { double newBalance = balance - amount; balance = newBalance; }
/** * Gets the current balance of the bank account. * @return the current balance */ public double getBalance() { return balance; }
/** * Transfer money from other account * @param ba another BankAccount object * @param amt the amount to transfer */ public void transfer(BankAccount ba, double amt) { ba.withdraw(amt); deposit(amt); }
/** * Print the balance */ public void printBalance() { System.out.printf(accountName + " Account Balance is $ %.2f ", balance); }
/** * Print a line of "="s */ public void printLineA() { System.out.println("=========================================="); }
/** * Print a line of "&"s */ public void printLineB() { System.out.println("&&&&&&&&&&&&&&&&&&&&&&&&&&&&&&&&&&&&&&&&&&&"); }
}
PROBLEM #1-Cash Register Using the CashRegister class provided in myCourses, write code in the main method of the RunCashRegister class to display sales transactions similar to that shown below in Figure 1 jGRASP exec: java RunCashRegister Total Sales: $ 70.35 Payment: 80.00 Change Due: $ 9.65 Total Sales: 185.10 Payment: 180.00 Not Enough Payment: $ -5.10 Figure 1 DETAILS 1. Download the CashRegister folder with the HW2 downloads. Save and unzip these downloads into your HW02 folder. The CashRegister class simulates a very simple cash register whose operations include: a. Ring up the sales price for a purchased item. b. Enter the amount of payment. c. Calculate the amount of change due to the customer 2. The CashRegister class contains the following set of methods for you to use to record and print sales transactions: 3Step by Step Solution
There are 3 Steps involved in it
Step: 1
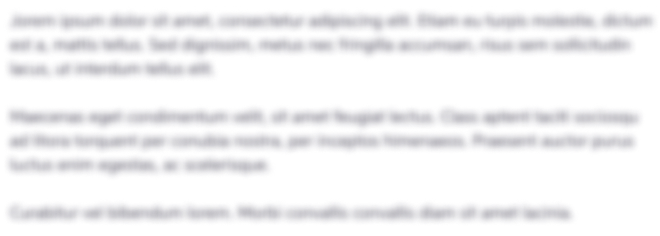
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started