Question
For this assignment, you will implement the provided matrix interface (see Matrix.java). This interface files defines not only which methods you must implement, but gives
For this assignment, you will implement the provided matrix interface (see Matrix.java). This interface files defines not only which methods you must implement, but gives documentation that describes how they should work. Already provided for you is a base file to modify (ser222_01_02_hw02_base.java). The base contains an currently empty matrix class as well as some simple testing code. In order for it to be a reliable type, we will implement it as an immutable type. Creating this ADT will involve creating 9 methods: MyMatrix(int[][] matrix) - a constructor. [4 points] public int getElement(int y, int x) - see interface. [2 points] public int getRows() - see interface. [1 points] public int getColumns() - see interface. [1 points] public MyMatrix scale(int scalar) - see interface. [3 points] public MyMatrix plus(Matrix other) - see interface. [3 points] public MyMatrix minus(Matrix other) - see interface. [3 points] public MyMatrix multiply(Matrix other) - see interface. [5 points] boolean equals(Object other) - see interface. [4 points] (Hint: see Point2D from earlier.) String toString() - see interface. [4 points]. Be aware that some of the methods throw exceptions - this should be implemented.
/** * An implementation of the Matrix ADT. Provides four basic operations over an * immutable type. * * @author (your name), Ruben Acuna * @version (version) */ public class ser222_01_02_hw02_base implements Matrix { //TODO: implement interface. /** * Entry point for matrix testing. * @param args the command line arguments */ public static void main(String[] args) { int[][] data1 = new int[0][0]; int[][] data2 = {{1, 2, 3}, {4, 5, 6}, {7, 8, 9}}; int[][] data3 = {{1, 4, 7}, {2, 5, 8}, {3, 6, 9}}; int[][] data4 = {{1, 4, 7}, {2, 5, 8}, {3, 6, 9}}; Matrix m1 = new ser222_01_02_hw02_base(data1); Matrix m2 = new ser222_01_02_hw02_base(data2); Matrix m3 = new ser222_01_02_hw02_base(data3); Matrix m4 = new ser222_01_02_hw02_base(data4); System.out.println("m1 --> Rows: " + m1.getRows() + " Columns: " + m1.getColumns()); System.out.println("m2 --> Rows: " + m2.getRows() + " Columns: " + m2.getColumns()); System.out.println("m3 --> Rows: " + m3.getRows() + " Columns: " + m3.getColumns()); //check for reference issues System.out.println("m2 --> " + m2); data2[1][1] = 101; System.out.println("m2 --> " + m2); //test equals System.out.println("m2==null: " + m2.equals(null)); //false System.out.println("m3==\"MATRIX\": " + m2.equals("MATRIX")); //false System.out.println("m2==m1: " + m2.equals(m1)); //false System.out.println("m2==m2: " + m2.equals(m2)); //true System.out.println("m2==m3: " + m2.equals(m3)); //false System.out.println("m3==m4: " + m3.equals(m4)); //true //test operations (valid) System.out.println("2 * m2: " + m2.scale(2)); System.out.println("m2 + m3: " + m2.plus(m3)); System.out.println("m2 - m3: " + m2.minus(m3)); //not tested... multiply(). you know what to do. //test operations (invalid) //System.out.println("m1 + m2" + m1.plus(m2)); //System.out.println("m1 - m2" + m1.minus(m2)); } }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
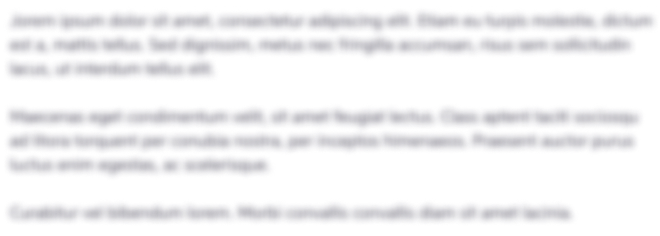
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started