Question
Gaussian random values. Experiment with the following function for generating random variables from the Gaussian distribution, which is based on generating a random point in
Gaussian random values. Experiment with the following function for generating random variables from the Gaussian distribution, which is based on generating a random point in the unit circle and using a form of the Box-Muller formula.
def gaussian(): r = 0.0 while (r >= 1.0) or (r == 0.0): x = -1.0 + 2.0 * random.random() y = -1.0 + 2.0 * random.random() r = x*x + y*y return x * math.sqrt(-2.0 * math.log(r) / r)
Take a command-line argument n and generate n random numbers, using an array a [ ] of 20 integers to count the numbers generated that fall between i * .05 and (i+1) * .05 for i from 0 to 19. Then use stddraw to plot the values and to compare your result with the normal bell curve.
Here's the Python code for the program so far:
import sys import stdio import random import math import numpy as np import matplotlib.pyplot as plt plt.rcParams["patch.force_edgecolor"] = True import statistics
# function header def gaussian(): r = 0.0 while (r >= 1.0) or (r == 0.0): x = -1.0 + 2.0 * random.random() y = -1.0 + 2.0 * random.random() r = x*x + y*y return x * math.sqrt(-2.0 * math.log(r) / r)
# command line for n integers n = int(sys.argv[1])
# store n random numbers using gaussian() distribution random_numbers = [] for i in range(n): random_numbers.append(gaussian())
# print random_numbers array stdio.writeln(random_numbers)
a = [] for i in range(20): count = 0 for j in random_numbers: if i*0.5 < j < (i+1)*0.5: count += 1 a.append(count)
stdio.writeln(a)
# mean emp_mean = np.mean(a) # standard deviation emp_stdev = np.std(a) # Plot a histogram of your sample with the absolute number of counts for each bin. Choosing 25 bins. m, bins, patches = plt.hist(a, 25, facecolor='g', alpha=0.5) plt.xlabel('Probability of success') plt.ylabel('Number of trials') plt.title('Histogram') plt.grid(True) plt.show()
# Plot a histogram of your standard sample with the normal counts to form a probability density. # Compare your histrogram with the density of the standard normal distribution by inserting its density into the histogram plot. Choosing 25 bins. # b = [] Needed??? for i in range(0, len(a)): a.append((a[i] - emp_mean)/emp_stdev)
m, bins, patches = plt.hist(a, 25,facecolor='g', alpha=0.5,density = True,label='standardized sample') stnm = np.random.standard_normal(100000) m, bins, patches = plt.hist(stnm, 25,facecolor='r', alpha=0.5,density = True,label='standard normal dist') plt.legend() plt.xlabel('Standard Deviation') plt.ylabel('Normalized counts') plt.title('Histogram') plt.grid(True) plt.show()
It appears as if I can run the code using the command line argument, an array a[] of 20 integers between the given condition from 0 to 19. The matplotlib module shows the bell curve comparison. I wasn't able to implement stddraw module. That's as far as I can solve for this assignment. I appreciate your coding assistance to produce the assignment's result!
Step by Step Solution
There are 3 Steps involved in it
Step: 1
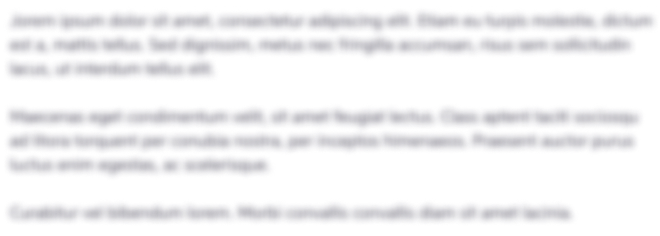
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started