Question
Hello, this is a project for a Data Structures class, I'm new to Java so I don't know a whole lot, so please, I would
Hello, this is a project for a Data Structures class, I'm new to Java so I don't know a whole lot, so please, I would appreciate if you could help me with this one. I just need to finish up the code for CountryData.java and Country.java. Thank you and please, let me know if the problem is not clear, because I wasn't able to include some parts due to the space limitation. Thank you! Note: Since I can't paste the CountryDataset.csv, I will post another question so just look for it pls. It will be called "(JAVA) CountryData.java" . Thank you!
Here is the other question: https://www.chegg.com/homework-help/questions-and-answers/java-countrydatajava-part-countrydatasetcsv-post-part-another-question-country-name-co2-em-q67696632
(JAVA) The code that you write for this assignment will build on top of the List ADT and one of the implementations of List that we've seen in class. The code will also use 1) a library for producing graphs called JFreeChart, 2) one additional class that I provided to make it easy for you to interact with the graphing library, and 3) a dataset containing information about countries, created using data from the World Bank:
- jfreechart-1.5.0.jar
- BarChart.java (link is in the comment)
- CountryDataset.csv (link is in the comments)
ASSIGNMENT
For this assignment, you will write a program that reads from a text file with one line for each country. Your program will need to represent each country as an instance of a class Country. It will need to convert the text file data into a List and display the data in one of two forms:
- As a graph of the top 10 countries for a given indicator, in sorted order (descending). An "indicator" is one of the types of data in the filee.g., CO2 emissions per capita.
- As a text list of all countries, in sorted order for a given indicator.
This will give you practice working with the List ADT, String, File, Scanner, loops, and simple classes. It's also a chance to see how your programming skills might be used to analyze large datasets.
1. The input file format
The input file is a text file that contains 0 or more lines of countries, with an initial line that gives the meaning of each item in the line. After that first line, each line of the input file will represent a single country via a comma-delimited list. Here's an example of the representation of Afghanistan, the alphabetically first country:
Afghanistan,0.31647873497721196,18168.86,82.60891342163086,17.011405995013824,0.103138023195208,3.006609490465427,34295325.14285714,3.900860497092041
Each of the numbers represents some information about the country. Specifically, the meaning of each of the items is as follows:
- Country Name
- CO2 emissions (metric tons per capita)
- Total greenhouse gas emissions (kt of CO2 equivalent)
- Access to electricity (% of population)
- Renewable energy consumption (% of total final energy consumption)
- Terrestrial protected areas (% of total land area)
- Population growth (annual %)
- Population (total)
- Urban population growth (annual %)
Open up the CSV file with a text editor to see all of the data.
The information in the data file you downloaded (CountryDataset.csv) is based off of the World Bank Indicators dataset. The original dataset contains information about each country for each indicator (item) and year from 1960-2019 (although some years are missing for some countries). For each country, the dataset I've given you contains a single number for each indicator. That number is the average value of the indicator from 2012-2019, skipping any years where there wasn't data for that country.
For your testing, you can use the data file I've provided; but you can also test on smaller subsets of the data to make sure that your output is correct and that your program doesn't crash if there are no countries.
2. The command-line syntax
Your main method should be structured so that the program expects two or three command-line arguments. The meaning of the command line arguments is as follows:
- The path to the file containing the country data. This includes the name of the file, and any directory information if the file is not in the same directory as the java program you're making. E.g., if the country file is in a subdirectory of the directory containing the java program and that subdirectory is named data, this argument would be data/CountryDataset.csv. (Note: there's nothing special you have to do to handle CountryDataset.csv versus data/CountryDataset.csv. File will do the right thing in both without you having to think about it.)
- The name of the indicator that we should sort the data on. This should be one of: CO2Emissions, TotalGreenhouseGasEmissions, AccessToElectricity, RenewableEnergy, ProtectedAreas, PopulationGrowth, PopulationTotal, or UrbanPopulationGrowth.
- (may or may not be present) A second indicator that we also want to display. This should be from the same list of indicators represented above. If this second indicator is present, then the program should display a graph (see more below). Otherwise, the program should display text.
3. The expected output
Your program should produce output based on whether two or three command-line arguments were present:
Two command line arguments: Text display
Three command line arguments: Graph display
PROGRAMING STEPS AND TIPS
Step 1: Writing the Country class
I provide the following starter code for the Country class. All you need to do is add the getter and setter for the countryName data field, and finish implementing getFeature, setFeature, and toString.
public class Country { private String countryName; private double co2Emissions; private double totalGreenhouseGasEmissions; private double accessToElectricity; private double renewableEnergy; private double protectedAreas; private double populationGrowth; private double populationTotal; private double urbanPopulationGrowth; public Country(String[] countryData) { countryName = countryData[0]; co2Emissions = Double.parseDouble(countryData[1]); totalGreenhouseGasEmissions = Double.parseDouble(countryData[2]); accessToElectricity = Double.parseDouble(countryData[3]); renewableEnergy = Double.parseDouble(countryData[4]); protectedAreas = Double.parseDouble(countryData[5]); populationGrowth = Double.parseDouble(countryData[6]); populationTotal = Double.parseDouble(countryData[7]); urbanPopulationGrowth = Double.parseDouble(countryData[8]); } // add the getter and setter for countryName here... // return the corresponding value of the given featureName ("CO2Emissions", "TotalGreenhouseGasEmissions", etc.) public double getFeature(String featureName) { return 0; // CHANGE ME } // set the corresponding value of the given featureName as newValue. public void setFeature(String featureName, double newValue) { // IMPLEMENT ME } public String toString() { // IMPLEMENT ME return null; } // Test toString: public static void main (String[] args) { String[] data = {"Country1", "1", "2", "3", "4", "5", "6", "7", "8"}; Country country1 = new Country(data); System.out.println(country1); // Or use one line: Country country2 = new Country(new String[] {"Country2", "9", "8", "NaN", "6", "5", "4", "3", "2"}); System.out.println(country2); } }
The parameter String[ ] countryData of the constructor is an array of Strings. Make sure this file compiles and passes the tests before moving to the next step. For example, to test the toString method using the existing code in main:
java Country
shoud display
Country1,1.0,2.0,3.0,4.0,5.0,6.0,7.0,8.0 Country2,9.0,8.0,NaN,6.0,5.0,4.0,3.0,2.0
Step 2: Designing the CountryDisplayer class
CountryDisplayer class has one data field which stores a list of countries. You should make sure that you put major self-contained operations into separate methods. This way, the main method reads like a high-level outline of the process you're implementing, rather than being a mess of all the code in a single method. For example, the following code shows some essential methods in CountryDisplayer (you're welcome to follow this example, but not required to).
public class CountryDisplayer { private List countries; public CountryDisplayer(String filePath) { // Read the country file and load the countries. } public ?? sortCountryList(??) { // ... } public ?? displayTextCountries(??) { // ... } public ?? displayCountryGraph(??) { // ... } public static void main(String[] args) { // If there's no command-line argument, print a usage statement // and exit. Otherwise, use args[0] as the input file path. if (args.length == 0) { // ... } //Call the methods you defined above to load, process, and display the countries } }
Step 3: Implementing the constructor of CountryDisplayer
The constructor takes one argument: a string with the location of the file with the countries (e.g.,"CountryDataset.csv"). Make sure to test that your CountryDisplayer properly loads different files of country data. Your program should never throw an exception or error message if given a valid filename that has a properly formatted country dataset (containing 0 or more countries). However, you don't need to worry about recovering if the user gives you a file that doesn't look like the example one.
Suppose line is a String variable representing one line of text read from the CSV file (data separated by commas), then line.split(",") would return a String array (String[]) where each element is one data entry. You can use this returned String array as the argument for the Country constructor:
Country country = new Country(line.split(","));
Add some testing code in main, and then compile and run it by:
javac CountryDisplayer.java java CountryDisplayer CountryDataset.csv
Depending on your design in Step 2, you might go through the following two steps in slightly different order. Choose the order that makes sense to you.
Step 4: Displaying the countries
Next, implement the method displayTextCountries() that prints out the country list, from the first country to the last country. This method will also help you see the result of sorting in Step 5.
Modify main so that you can have the option of testing this functionality by passing in two command-line arguments. Then, compile and run it by:
javac CountryDisplayer.java java CountryDisplayer CountryDataset.csv
Step 5: Tips for implementing the sorting functionality
Modularity is especially important for sortingyou don't want to have completely different sorting methods for all of the different possible indicators. Think carefully about how to avoid duplicating code. You might need to implement some helper methods to make the sorting part shorter and more readable.
One helper method I highly recommend is a getDataEntry method (implement this first!):
/** * returns the data entry of the Country at the specified index in the list, and with the specified column name. */ private double getDataEntry(int index, String column) { }
For example, getDataEntry(10, "PopulationGrowth") would return the population growth of the 11th country in the list (indexing starts with 0 for lists in Java Class Library). I made this method "private" because I don't intend to allow clients of this class to use itit's for internal use only. Test it before moving on.
Step 6: Implementing displayCountryGraph method
While the BarChart class might look complicated at first, you should only need to use a few methods: a constructor, the addValue method, and displayChart. As mentioned before, you'll create an instance of BarChart for your graph, and you'll add data to a particular series using addValue(String country, double value, String series) method of BarChart.
Modify main so that you can have the option of testing this functionality by passing in three command-line arguments.
As noted above, to compile all of the files in a folder at one time and include the jar file with the graphing classes, you can run javac -classpath .:jfreechart-1.5.0.jar *.java while in that folder. The "*.java" means anything that ends with ".java".
If the user doesn't properly type in an indicator as the second command-line argument, or if they have a third command-line argument that isn't one of the indicators, display a helpful message for them about how to use your classthis is called a usage statement.
REQUIREMENTS
This is a partial list of the things that we'll be looking for when evaluating your work:
- Correct command-line syntax and behavior (including naming the main class CountryDisplayer)
- Program compiles
- Program runs correctly on test input
- CSV File parsing
- Sorting according to criteria described above
- Use of the BarChart class to display a graph of your analyses
- Correct formatting of output
- Correct Country class implementation
- Code uses the ADT implementation correctly: the name of the class implementation list (e.g., ArrayList) appears only in the initialization of List variables, and then only on the right-hand side.
- Good coding style
Step by Step Solution
There are 3 Steps involved in it
Step: 1
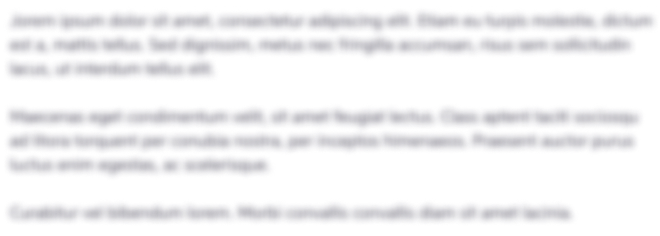
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started