Question
Help answering these questions with respect to the code. C++ 1) In what data type can you store something like the problem set name that
Help answering these questions with respect to the code. C++
1) In what data type can you store something like the problem set name that might be anything from one character to a whole bunch of text?
2) How can you detect that the problem set name is a quoted string? How can you read in the whole thing to a single variable? (Don't worry that the user has missed a close quote.)
3) If the user's problem set name is a quoted string, how can you remove the quotes from it? (Just like C++ won't leave your quotes on a string when it prints it, you shouldn't leave the user's quotes on their string. They are just there to separate the problem set name from the problem list.)
4) When placing items (say problem numbers?) into a list, how can you keep those items sorted from the beginning?
5) How can you avoid placing a duplicate item into a list? (Note: not how can you remove duplicates from a list -- avoid having any duplicates in the list in the first place!)
6) If the problem list is long (like in math or physics), how can you wrap the long output line to multiple lines without too much difficulty? (Hint: The output line can be around 70-75 chars long, we saw in 121 how to tell how many digits long an integer is, we know we are outputting a comma and space between the problem numbers, and we know how many chars we are adding before the list and after the list. Just don't forget the 'and' before the last element. So, knowing all that, especially that you need to drop to a new line after every 70-ish chars you've output, how do you tell if total chars printed is 70*n?)
#include
#include
#include
using namespace std;
int main()
{
//declaring necessary variables
string input;
list
list
//1st character of the input must be L
cout << "Enter hyphenated assignment list in comma seperated manner(in the form L1-3,6): "< getline (cin,input);//taking input input.erase(0,1);//erasing L istringstream s1(input); string tokenOut,tokenIn; int count=0,fnum,lnum; //extracting numbers from string input while(getline(s1, tokenOut, ',')) { count=0; istringstream s2(tokenOut); while(getline(s2,tokenIn,'-')) { count++; if(count==1) fnum = stoi(tokenIn); if(count == 2) lnum = stoi(tokenIn); } if(count == 2) { for(int i=fnum;i<=lnum;i++) { mylist.push_back(i);//populating mylist with numbers } } if(count==1) mylist.push_back(fnum); //populating mylist with numbers } mylist.sort();//sorting mylist mylist.unique();//removing duplicate from mylist //displaying mylist in a formatted way int num = 0; if(mylist.size()==1) cout<<"Do problem"; else cout<<"Do problems"; for (it=mylist.begin(); it!=mylist.end(); ++it) { num++; if(num==1) { cout << " " << *it; continue; } else if(num== mylist.size()) { cout << ", and " << *it; } else cout << ", " << *it; } cout<<" of L."; return 0; }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
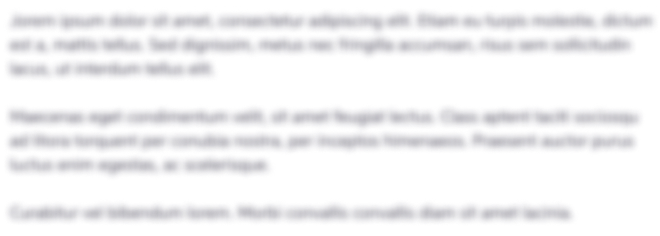
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started