Question
help me, please, i want to see the way u do step by step please please Overview For this assignment, write a program that will
help me, please, i want to see the way u do step by step please please
Overview
For this assignment, write a program that will process two sets of random numeric information. The information will be needed for later processing, so it will be stored in arrays that will be displayed, sorted, and displayed (again).
Basic Program Logic
The main function for this program is pretty basic. It should start by creating two arrays. Each array should hold a maximum of 100 double or float elements. There should also be at least two integers. Each integer will hold the number of values that are placed into the arrays (Note: this is needed because it's possible that an entire array is not filled with data and only the part that is used should be processed).
Use srand to set the seed value for the random number generator. The program that is handed in will use srand(0) but any value can be used as the program is being developed.
Call the buildArray function that is described below to fill one of the arrays with a random number of random values. The value that is returned from the function should be saved in (assigned to) one of the integer variables.
Call the printArray function that is described below to display the first array of unsorted values with a title similar to "First Array -- Unsorted Random Numbers".
Call the sortArray function that is described below to put the values in the first array in ascending order.
Call the printArray function a second time to display the sorted values in the first array with a title similar to "First Array -- Sorted Random Numbers".
Call the buildArray function a second time to fill the other array with a random number of random values. The value that is returned from the function should be saved in (assigned to) the other integer variable.
Call the printArray function to display the second array of unsorted values with a title similar to "Second Array -- Unsorted Random Numbers".
Call the sortArray function to put the values in the second array in ascending order.
Finally, call the printArray function to display the sorted values in the second array with a title similar to "Second Array -- Sorted Random Numbers".
The Functions
The following functions are required for the assignment:
double randDouble()
This function will generate a random double value that is within a specific range. It takes no arguments and returns a double: the random number.
The rand function that has been used in previous assignments generates a random integer value. This function will still use that value but it will be used in a formula that changes the value to a double. The formula is:
lower bound + ( random integer / (maximum possible random number / (upper bound - lower bound)))
See the Symbolic Constants section for the "lower bound," "upper bound," and "maximum possible random number" values.
int buildArray( double array[] )
This function will fill an array of doubles with a random number of random values.
It takes as its argument an array of doubles: the array that will hold the numeric information and returns an integer: the number of values that were placed in the array.
The function should start by generating a random integer value that represents the number of values to place into the array. This value should be between 30 and 100. Use the following formula to generate a value within a specific range:
minimum_value + (random integer % (maximum_value - minimum_value + 1))
Once the number of values has been determined, write a loop that will execute that number of times. Inside of the loop, call the randDouble function that was previously described to get a random double value and then put the value that is returned from the function into the array argument.
After the values have been placed into the array, return the number of values that were placed in the array (the random integer from earlier).
void printArray( double array[], int numberOfValues, string title )
This function will display the numeric information in an array of doubles. There should be 8 values displayed per line, with the exception of the last line, which may have fewer than 8 values.
This function takes three arguments: the first argument is an array of doubles that holds the numbers to be displayed, the second argument is an integer that holds the number of values to be displayed, and the third argument is a string that holds a title that should be displayed before displaying the numeric information. The function returns nothing.
The function should first execute a cout statement that will display whatever the string argument is holding and the number of values to be displayed. After that, execute a loop that executes numberOfValues number of times. Within the loop, determine if 8 values have been displayed. If 8 values have been displayed, cout a newline character. After that, display a number from the array.
The values should be displayed with exactly 3 digits after the decimal point and the decimal points should line up from one line to the next.
void sortArray( double array[], int numberOfValues, char sortType )
This function will use the selection sort algorithm that was presented in lecture to sort the array.
This function takes three arguments: the first argument is an array of doubles that holds the numbers to be sorted, the second argument is an integer that holds the number of values to be sorted, and the third argument is a character that holds the type of sort that should be performed. The function returns nothing.
For the sortType argument, a value of 'A' or 'a' indicates that an ascending order sort should be performed. A value of 'D' or 'd' indicates that a descending order sort should be performed. You may assume that the sortType argument will only contain a valid value.
Symbolic Constants
This program requires the use of 6 symbolic constants. One of the constants is predefined and the remaining 5 must be defined by you.
Pre-defined constant
RAND_MAX is a symbolic constant that holds the maximum possible random number that is available on the system that is executing the program. At a minimum, the value will be 32767. Make sure to add #include
Constants that need to be created/defined
The first constant is a double that represents the lower bound of the double values that will be placed into the array of doubles. The value should be 0. (Note: make sure to use the value 0.0 if using #define)
The second constant is a double that represents the upper bound of the double values that will be placed into the array of doubles. The value should be 150. (Note: make sure to use the value 150.0 if using #define)
The third constant is an integer that represents the minimum number of values that the array of doubles can hold. The value should be 30.
The fourth constant is an integer that represents the maximum number of values that the array of doubles can hold. The value should be 100.
The fifth constant is an integer that represents the maximum number of values to display per line of output. The value should be 8.
Programming Requirements:
All 6 of the symbolic constants must be used in the program.
Add #include
Make sure to seed the random number generator by calling the srand function. This MUST be done in main before calling the buildArray function. The file that is handed in for grading must use srand(0);.
As with program 6, each of the functions should have a documentation box that describes the function. This is the last reminder about function documentation that will appear in the assignment write-ups.
Hand in a copy of the source code using Blackboard.
Output
Run 1 (using srand(0))
First Array -- Unsorted Random Numbers -- 68 values 35.336 97.223 11.156 40.536 54.004 38.293 147.794 47.838 140.135 26.794 128.636 5.228 1.286 94.014 72.883 40.948 120.327 13.720 67.202 96.023 145.990 99.132 118.592 84.492 6.056 132.211 10.254 44.519 147.761 11.197 2.701 3.845 85.087 77.396 97.218 108.086 57.758 57.021 3.969 135.195 31.486 129.199 81.883 144.644 94.229 55.904 142.419 34.690 78.124 34.924 134.605 56.211 61.823 111.643 53.327 56.439 32.850 10.671 88.186 101.233 64.711 123.270 5.045 99.118 38.472 111.409 127.519 25.626 First Array -- Sorted Random Numbers -- 68 values 1.286 2.701 3.845 3.969 5.045 5.228 6.056 10.254 10.671 11.156 11.197 13.720 25.626 26.794 31.486 32.850 34.690 34.924 35.336 38.293 38.472 40.536 40.948 44.519 47.838 53.327 54.004 55.904 56.211 56.439 57.021 57.758 61.823 64.711 67.202 72.883 77.396 78.124 81.883 84.492 85.087 88.186 94.014 94.229 96.023 97.218 97.223 99.118 99.132 101.233 108.086 111.409 111.643 118.592 120.327 123.270 127.519 128.636 129.199 132.211 134.605 135.195 140.135 142.419 144.644 145.990 147.761 147.794 Second Array -- Unsorted Random Numbers -- 94 values 64.533 60.486 21.438 76.463 69.857 72.979 12.575 125.317 132.165 82.418 76.325 14.429 54.105 107.596 1.094 19.163 12.836 132.467 13.839 47.311 93.995 97.932 73.336 52.983 118.944 63.910 2.857 32.342 126.205 58.733 110.631 56.014 143.051 108.200 71.500 117.772 58.138 111.345 68.310 104.254 31.179 55.217 16.320 115.319 0.545 85.820 119.251 89.816 74.773 27.320 80.221 16.375 68.877 116.875 66.185 134.001 121.448 147.102 149.149 1.991 53.409 133.488 122.748 44.139 4.468 148.901 17.501 96.564 8.094 42.317 63.283 64.185 141.028 134.111 122.346 65.293 108.219 4.862 41.415 69.033 49.197 70.168 46.295 5.416 76.719 58.335 77.323 52.617 33.697 133.364 39.241 131.158 129.340 130.329 Second Array -- Sorted Random Numbers -- 94 values 149.149 148.901 147.102 143.051 141.028 134.111 134.001 133.488 133.364 132.467 132.165 131.158 130.329 129.340 126.205 125.317 122.748 122.346 121.448 119.251 118.944 117.772 116.875 115.319 111.345 110.631 108.219 108.200 107.596 104.254 97.932 96.564 93.995 89.816 85.820 82.418 80.221 77.323 76.719 76.463 76.325 74.773 73.336 72.979 71.500 70.168 69.857 69.033 68.877 68.310 66.185 65.293 64.533 64.185 63.910 63.283 60.486 58.733 58.335 58.138 56.014 55.217 54.105 53.409 52.983 52.617 49.197 47.311 46.295 44.139 42.317 41.415 39.241 33.697 32.342 31.179 27.320 21.438 19.163 17.501 16.375 16.320 14.429 13.839 12.836 12.575 8.094 5.416 4.862 4.468 2.857 1.991 1.094 0.545
Run 2 using srand(time(0))
First Array -- Unsorted Random Numbers -- 83 values 85.893 84.954 115.419 93.364 19.730 80.436 92.572 107.331 32.832 52.177 65.966 5.951 26.556 114.257 13.962 17.377 101.682 83.641 52.704 117.022 35.542 92.549 117.850 25.974 55.579 11.577 63.874 56.961 120.167 50.919 129.240 112.563 92.750 31.747 140.913 65.334 120.144 145.042 106.717 24.619 0.989 89.958 118.876 119.590 5.278 9.037 117.237 103.307 130.902 112.320 135.621 57.511 108.104 7.645 79.672 102.725 137.892 29.293 109.738 93.451 25.210 32.118 77.122 53.079 142.914 43.448 137.228 78.275 87.408 39.845 87.967 17.455 111.959 90.988 6.926 53.079 148.563 87.793 35.679 92.444 4.299 147.038 84.707 First Array -- Sorted Random Numbers -- 83 values 0.989 4.299 5.278 5.951 6.926 7.645 9.037 11.577 13.962 17.377 17.455 19.730 24.619 25.210 25.974 26.556 29.293 31.747 32.118 32.832 35.542 35.679 39.845 43.448 50.919 52.177 52.704 53.079 53.079 55.579 56.961 57.511 63.874 65.334 65.966 77.122 78.275 79.672 80.436 83.641 84.707 84.954 85.893 87.408 87.793 87.967 89.958 90.988 92.444 92.549 92.572 92.750 93.364 93.451 101.682 102.725 103.307 106.717 107.331 108.104 109.738 111.959 112.320 112.563 114.257 115.419 117.022 117.237 117.850 118.876 119.590 120.144 120.167 129.240 130.902 135.621 137.228 137.892 140.913 142.914 145.042 147.038 148.563 Second Array -- Unsorted Random Numbers -- 44 values 43.210 9.609 7.370 52.448 89.477 24.633 116.372 116.655 62.967 86.566 41.099 137.796 83.050 17.542 149.368 34.654 101.590 12.159 81.915 136.550 101.086 136.756 123.183 14.763 18.879 50.552 106.690 60.115 133.392 40.747 78.568 36.123 23.283 38.705 117.640 12.571 135.525 109.551 85.430 9.137 23.269 132.266 4.651 3.745 Second Array -- Sorted Random Numbers -- 44 values 149.368 137.796 136.756 136.550 135.525 133.392 132.266 123.183 117.640 116.655 116.372 109.551 106.690 101.590 101.086 89.477 86.566 85.430 83.050 81.915 78.568 62.967 60.115 52.448 50.552 43.210 41.099 40.747 38.705 36.123 34.654 24.633 23.283 23.269 18.879 17.542 14.763 12.571 12.159 9.609 9.137 7.370 4.651 3.745
Step by Step Solution
There are 3 Steps involved in it
Step: 1
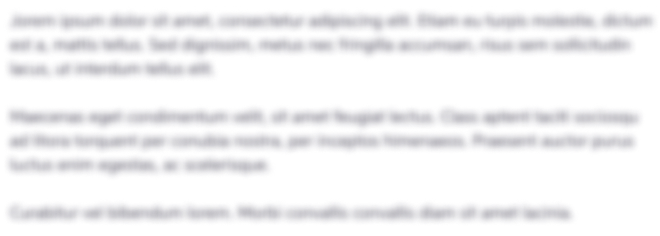
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started