Question
Here are the directions for my C++ assignment: Declare and define a C++ class called odometer that will track miles driven and gallons used by
Here are the directions for my C++ assignment:
Declare and define a C++ class called odometer that will track miles driven and gallons used by a vehicle. The class should have member variables for total miles driven and gallons consumed. Include the following mutator functions in your class:
miles driven and gallons of gas consumed
reset odometer to zero
reset gallons
Include the following accessor functions in your class:
return fuel efficiency in miles per gallon (MPG)
return odometer reading (total miles driven since the last reset)
Your class declaration should be in a header file with inclusion guards. Your class implementation should be in a cpp file.
Create a driver (main) program to test your class. Your driver program should be in a separate cpp file.
You may create a default constructor for this assignment OR rely on the automatically created default constructor
*** I'm attempting to achieve this desired output. It's updating the total miles driven in the first two, and then it resets right before the third update. ***
Here's the the main.cpp file which contains my main driver:
#include
int main() { odometer o; o.setMilesAndGallons(100,10); cout
Here's the odometer.cpp file which contains my function definitions:
#include
odometer::odometer() { totalMilesDriven=0; gallonsUsed=0; } //mutator method to set miles and gallons void odometer::setMilesAndGallons(long milesDriven, long usedGallons) { totalMilesDriven= milesDriven; gallonsUsed= usedGallons; } //reset the miles and gallons to zero void odometer::resetToZero() { gallonsUsed=0; totalMilesDriven=0; } //mutator function to reset the gallons void odometer::resetGallons() { gallonsUsed=0; } //method to check the fuel efficiency double odometer::fuelEff() { return (double)((double)totalMilesDriven / (double)gallonsUsed); } // method to get odometer reading long odometer::odometerReading() { return totalMilesDriven; }
Here's the odometer.h header file which contains my class:
#include
class odometer { //private member variables for storing miles and gallons private: long totalMilesDriven; long gallonsUsed; public: //using default constructor odometer(); //mutator method to set miles and gallons void setMilesAndGallons(long totalMilesDriven, long gallonsUsed); //reset the miles and gallons to zero void resetToZero(); //mutator function to reset the gallons void resetGallons(); //method to check the fuel efficiency double fuelEff(); // method to get odometer reading long odometerReading();
};
#endif // ODOMETER_H
Here's my current output:
Step by Step Solution
There are 3 Steps involved in it
Step: 1
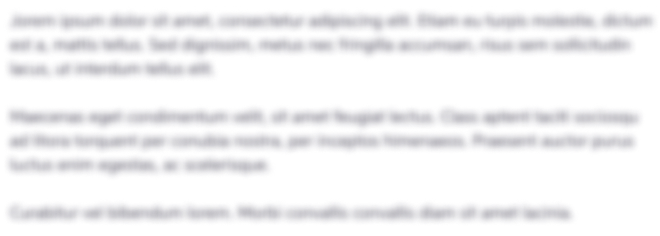
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started