Question
How to declare C++ program that plays tic-tac-toe. The tic-tac-toe game is played on a 3x3 grid as in O X O The game is
How to declare C++ program that plays tic-tac-toe. The tic-tac-toe game is played on a 3x3 grid as in O X O
The game is played by two players, who take turns. The first player marks move with an O, the second with an X. The player who has formed a horizontal, vertical, or diagonal sequence of three marks wins. Your program should draw the game board, accept numbers to indicate the players move, change player after every successful move, and pronounce the winner.
7 Home 8 9 PgUp 4 5 6 1 End 2 3 PgDn
The program should flip a coin to see who goes first (Player 1 or Player 2)
Please, use the following functions & code:
#include
#include
#include
#include
#include
using namespace std;
const int ROWS = 3;
const int COLUMNS = 3;
void randomize();
int random(int a, int b);
void draw_board(string b[][COLUMNS], int rows);
void reset_board(string b[][COLUMNS], int rows);
bool upper_left_to_lower_right(string b[ROWS][COLUMNS]);
bool lower_left_to_upper_right(string b[ROWS][COLUMNS]);
bool check_col(string b[ROWS][COLUMNS], int col);
bool check_row(string b[ROWS][COLUMNS], int row);
bool check_for_winner(string b[ROWS][COLUMNS]);
bool is_game_tied(string b[ROWS][COLUMNS]);
bool move_legit(string b[ROWS][COLUMNS], int loc);
void get_move(string b[ROWS][COLUMNS], int& player);
int main()
{
{
string board[ROWS][COLUMNS] = { {"X", "O", "X"}, // B[2][0] B[2][1] B[2][2]
{"O", "O", " "}, // B[1][0] B[1][1] B[1][2]
{" ", "X", " "} }; // B[0][0] B[0][1] B[0][2]
string choice;
int coin;
int current_player = 0;
bool someone_won = false;
reset_board(board, 3);
cout << " Welcome to TIC-TAC-TOE!" << endl;
cout << " Do you like to play Tic-tac-toe? (Yes or No) ";
cout << " We will flip a coin to see who go first!" << endl;
cout << " Enter (H)eads or (T)ails: ";
cin >> choice;
coin = random(0, 1);
if ((coin == 0 && choice == "H") || (coin == 1 && choice == "T"))
{
cout << " You won! You go first as Player 1!" << endl;
}
else
{
cout << " Player 2 won! Player 2 go first!" << endl;
}
current_player = 1;
cout << " Press Enter to continue ... ";
getline(cin, choice);
getline(cin, choice);
while (!is_game_tied(board) && !someone_won)
{
draw_board(board, 3);
cout << endl;
cout << " Player " << current_player << ". It's your turn." << endl;
get_move(board, current_player);
someone_won = check_for_winner(board);
}
if (someone_won)
{
draw_board(board, 3);
if (current_player == 1)
cout << " Player 2 won!! " << endl;
else
cout << " Player 1 won!! " << endl;
}
else
{
draw_board(board, 3);
cout << " Game Tied!" << endl;
}
system("pause");
return 0;
}
void randomize()
{
srand(static_cast
}
int random(int a, int b)
{
return a + rand() % (b - a + 1);
}
void reset_board(string b[][COLUMNS], int rows)
{
for (int i = 0; i < rows; i++)
for (int j = 0; j < COLUMNS; j++)
b[i][j] = " ";
}
void draw_board(string b[][COLUMNS], int rows)
{
system("cls");
string line = " +---+---+---+ ";
cout << " TIC-TAC-TOE" << endl;
for (int i = rows - 1; i >= 0; i--)
{
cout << line << endl;
for (int j = 0; j < COLUMNS; j++)
{
cout << " | " << b[i][j];
}
cout << " | " << endl;
}
cout << line << endl;
}
/**
* Return true if all elements on the diagonal joining upper-left
* and the lower right corners all the same.
* @param b the board a 3 x 3 array of String
* @return true if all elements are the same.
*/
bool upper_left_to_lower_right(string b[ROWS][COLUMNS])
{
}
/**
* Return true if all elements on the diagonal joining lower-left
* and the upper-right corners all the same.
* @param b the board a 3 x 3 array of String
* @return true if all elements are the same.
*/
bool lower_left_to_upper_right(string b[ROWS][COLUMNS])
{
}
/**
* Return true if all elements in column col are the same.
* @param b the board a 3 x 3 array of String
* @param col the column to check
* @return true if all elements are the same.
*/
bool check_col(string b[ROWS][COLUMNS], int col)
{
}
/**
* Return true if all elements in row row are the same.
* @param b the board a 3 x 3 array of String
* @param row the row to check
* @return true if all elements are the same.
*/
bool check_row(string b[ROWS][COLUMNS], int row)
{
}
/**
* Return true if there is a winner.
* @param b the board a 3 x 3 array of String
* @return true if there is a winner, false otherwise
*/
bool check_for_winner(string b[ROWS][COLUMNS])
{
}
/**
* Return false if there is a blank space in the board.
* @param b the board a 3 x 3 array of String
* @return false if there is a blank space in the board,
* true if there are no moves to be made.
*/
bool is_game_tied(string b[ROWS][COLUMNS])
{
}
/**
* Return true if there is a blank space in the location loc.
* @param b the board a 3 x 3 array of String
* @param loc the location number of the move as given by
* 6 | 7 | 8
* -----------
* 3 | 4 | 5
* -----------
* 0 | 1 | 2
* @return true if there is a blank space in the location loc.
*/
bool move_legit(string b[ROWS][COLUMNS], int loc)
{
}
/**
* Gets the inputted move from the player, determines if it is a legitimate move
* then puts the correct symbol in the spot and updates the player.
* The move is based on the number keypad:
* 7 | 8 | 9
* -----------
* 4 | 5 | 6
* -----------
* 1 | 2 | 3
* @param b the board a 3 x 3 array of String
* @param player the current player number
*/
void get_move(string b[ROWS][COLUMNS], int& player)
{
int move;
do
{
cout << " Enter your move according to the number keypad: ";
cin >> move;
move--;
} while (!move_legit(b, move));
if (player == 1)
{
b[move / COLUMNS][move % COLUMNS] = "O";
player = 2;
}
else
{
b[move / COLUMNS][move % COLUMNS] = "X";
player = 1;
}
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
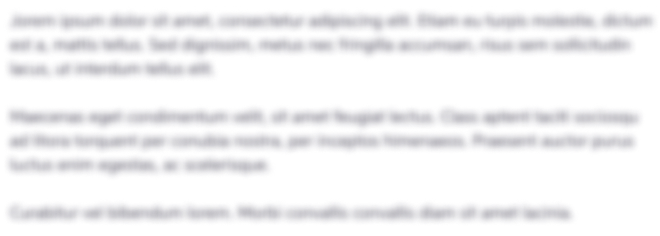
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started