Question
I have done the coding part but i need help in commenting the methods, how we add comments with/*...///. The program should implement the following
how we add comments with/*...///. The program should implement the following high-level algorithm: Program: DNA Processor Steps: 1 Display the program's name 2 Prompt the user to enter a DNA sequence (any non-whitespace characters are allowed) 3 Convert that String to upper case, replace all non-DNA characters with a dot (.) 4 Display the error rate (details below) 5 Do 5-1 | Display a menu of operations for working with that DNA sequence 6 While the use has not selected quit After step 3, only the 'cleaned' sequence of characters will be used. For example, if the user typed 'gaxTT/aca' then it will be displayed later as 'GA.TT.ACA'. For each operation that displays some information the program should present that information with some suitable prefix, such as 'Sequence: '. The available operations are: Display the sequence, which should display the current value of the sequence. Display the error rate, which is the proportion of invalid characters in the sequence, expressed as a percentage. In the example above this is approximately 22%. (It's OK if the program displays this with too much precision, that is, too many digits after the decimal place. We don't cover number formatting in the unit.) Transcribe the entire sequence, which should display the mRNA equivalent of the entire sequence (i.e., the sequence with all Ts converted to Us) Transcribe a section of the sequence, between two points. The user should be asked to give the start and end of the section to transcribe, which will be values between 1 and the length of the sequence, inclusive. Both the start and end should be included in the transcribed section so, given the example above, if the user asked to transcribe between positions 2 and 5 the program will produce 'A.UU'. Switch to the sequence's complement, which should change the current value of the sequence to its complementary sequence and then display the new value. The rules to apply are: A becomes T, T becomes A, G becomes C, C becomes G, . remains unchanged. For example, the sequence above would change to CT.AA.TGT (until the user chooses to switch to the complement again, sometime later) Implementation advice Cleaning the input Strings have a replaceAll(String regex, String replacement) method that will replace all substrings that match the given regular expression (a pattern that can match a variety of String values) with the given replacement. As we don't cover regular expressions in the unit, here are some patterns that you will find useful: "[XYZ]" matches any single character from the set 'X', 'Y' or 'Z' "[^XYZ]" matches any single character that is not in the the set 'X', 'Y' or 'Z' You should not write a loop to clean the input text. Calculating the error rate The error rate can be calculated either with a loop (which is conceptually easier) or using a combination of String methods (which is a little shorter). Transcribing the sequence This is most easily done using a String method (mentioned above) instead of a loop. Remember you only need to change one of the characters. There's another String method, discussed in the notes, that will help with transcribing a section, but remember that the user will describe positions in the sequence with values 1 and above (not the zero-based positions you use in your code). Constructing the sequence's complement This is possible with the use of several String methods, but is actually easier if you write a loop to construct a new String from the current value. Remember that, given a String variable s, initialised as "", s += "some text"; will append some text to the end of s.
import java.util.Scanner; /** * DNA PROCESSOR (9.1PP Divide and Conquer) * * @author Nitish Gaire */ public class DNASequence { /*To take the input from the user as per the algorithm */ String updatedSequence; public void operationsOptions() { System.out.println("1:Display DNA Sequence: " + // to display the sequence of entered DNA// "2:Display Error Rate: " + // to display the % proportion of invalid charaters// "3:Transcribe Entire Sequence: " + // to transcribe the entire sequence// "4:Transcribe Section of a Sequence: " + // to describe the certain portion between points within length of sequence// "5:Switch to complement of a Sequence: " + // to show ew value switchung the contemporary with current one// "6:Quit: "); // to opt out // } /** first operation display method to display the sequence of entered DNA */ public void displaySequence(String dnaSequence) { if(updatedSequence == null) { // conditional statement to verify updated sequence// System.out.println("Your current DNA Sequence is: " + dnaSequence); } else { System.out.println("Your current DNA Sequence is: " + updatedSequence); } } /** method to display the percentage proportion of the invalid characters */ public void displayError(String dnaSequence) { int originalLength, dotLength, error; originalLength = dnaSequence.length(); dotLength = dnaSequence.replaceAll("[^.]", "").length(); error = dotLength * 100 / originalLength; // error calculation// System.out.println(error); System.out.println("dotlength is: " + dotLength + "originalLength is: " + originalLength + " Your error is: " + error + "%"); } public void transcribeAll(String dnaSequence) { System.out.println("Entire Transcribe"); dnaSequence = dnaSequence.replace("T", "U"); System.out.println(dnaSequence); } public void transcribeSection(String dnaSequence, Scanner sc) { int start, end; String selectedString; System.out.println("Select your starting point"); start = sc.nextInt(); System.out.println("Select your end point"); end = sc.nextInt(); selectedString = dnaSequence.substring(start - 1, end); selectedString = selectedString.replaceAll("T", "U"); System.out.println((selectedString)); } public void sequenceComplement(String dnaSequence) { if (updatedSequence!=null) { dnaSequence = updatedSequence; } dnaSequence = dnaSequence.replaceAll("A", "B") .replaceAll("T", "A") .replaceAll("C", "D") .replaceAll("G", "C") .replaceAll("B", "T") .replaceAll("D", "G"); System.out.println("complement is: " + dnaSequence); updatedSequence = dnaSequence; } public static void main(String[] args) { System.out.println("***---*** Welcome to DNA Processor Program ***---***"); Scanner sc = new Scanner(System.in); System.out.println("Enter DNA Sequence: "); String userInput = sc.nextLine().trim().toUpperCase(); int choice; String dnaSequence; DNASequence dSequence = new DNASequence(); dnaSequence = userInput.replaceAll("[s]", ""); dnaSequence = dnaSequence.replaceAll("[^actgACTG]", "."); dSequence.operationsOptions(); do { choice = sc.nextInt(); switch (choice) { case 1: dSequence.displaySequence(dnaSequence); break; case 2: dSequence.displayError(dnaSequence); break; case 3: dSequence.transcribeAll(dnaSequence); break; case 4: dSequence.transcribeSection(dnaSequence, sc); break; case 5: dSequence.sequenceComplement(dnaSequence); break; case 6: break; } } while (choice != 6); } }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
import javautilScanner DNA Processor program 91PP Divide and Conquer This program simulates a DNA processor with various functionalities author Nitish Gaire public class DNASequence private static Str...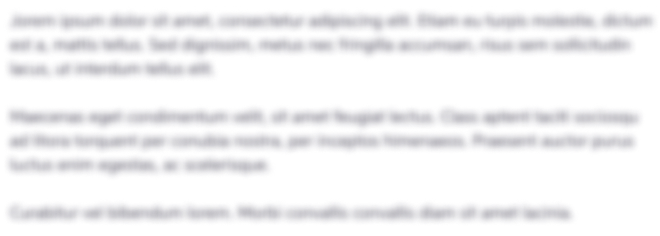
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started