Question
I have this code and the test driver to run the code, but it will not compile, what is the issue? // Shape.h #include class
I have this code and the test driver to run the code, but it will not compile, what is the issue?
// Shape.h
#include
class Shape {
public:
Shape();
virtual ~Shape() {}
void SetFillColor(std::string fill_color);
std::string GetFillColor();
void SetBorderColor(std::string border_color);
std::string GetBorderColor();
virtual float Area() = 0;
virtual float Perimeter() = 0;
protected:
std::string shape_type_;
int num_sides_;
std::string fill_color_;
std::string border_color_;
void SetShapeType(std::string shape_type);
void SetNumSides(int num_sides);
};
// Shape.cpp
#include "Shape.h"
Shape::Shape() : shape_type_("Shape"), num_sides_(0), fill_color_("White"), border_color_("Black") {}
void Shape::SetFillColor(std::string fill_color) {
fill_color_ = fill_color;
}
std::string Shape::GetFillColor() {
return fill_color_;
}
void Shape::SetBorderColor(std::string border_color) {
border_color_ = border_color;
}
std::string Shape::GetBorderColor() {
return border_color_;
}
void Shape::SetShapeType(std::string shape_type) {
shape_type_ = shape_type;
}
void Shape::SetNumSides(int num_sides) {
num_sides_ = num_sides;
}
// Triangle.h
#include "Shape.h"
class Triangle : public Shape {
public:
Triangle();
Triangle(int side1, int side2, int side3);
void SetSide1(int side);
int GetSide1();
void SetSide2(int side);
int GetSide2();
void SetSide3(int side);
int GetSide3();
float Area() override;
float Perimeter() override;
private:
int side1_;
int side2_;
int side3_;
};
// Triangle.cpp
#include "Triangle.h"
#include
Triangle::Triangle() : Shape(), side1_(0), side2_(0), side3_(0) {
SetShapeType("Triangle");
SetNumSides(3);
}
Triangle::Triangle(int side1, int side2, int side3) : Shape(), side1_(side1), side2_(side2), side3_(side3) {
SetShapeType("Triangle");
SetNumSides(3);
}
void Triangle::SetSide1(int side) {
side1_ = side;
}
int Triangle::GetSide1() {
return side1_;
}
void Triangle::SetSide2(int side) {
side2_ = side;
}
int Triangle::GetSide2() {
return side2_;
}
void Triangle::SetSide3(int side) {
side3_ = side;
}
int Triangle::GetSide3() {
return side3_;
}
float Triangle::Area() {
float s = (side1_ + side2_ + side3_) / 2.0f;
return sqrt(s * (s - side1_) * (s - side2_) * (s - side3_));
}
float Triangle::Perimeter() {
return side1_ + side2_ + side3_;
}
// Circle.h
#include "Shape.h"
class Circle : public Shape {
public:
Circle();
Circle(int radius, std::string fill_color, std::string border_color);
void SetRadius(int radius);
int GetRadius();
void SetFillColor(std::string fill_color) override;
std::string GetFillColor() override;
void SetBorderColor(std::string border_color) override;
std::string GetBorderColor() override;
float Area() override;
float Perimeter() override;
private:
int radius_;
}
// Circle.cpp
#include "Circle.h"
#include
Circle::Circle() : Shape(), radius_(0), fill_color_(""), border_color_("") {
SetShapeType("Circle");
SetNumSides(0);
}
Circle::Circle(int radius, std::string fill_color, std::string border_color) : Shape(), radius_(radius), fill_color_(fill_color), border_color_(border_color) {
SetShapeType("Circle");
SetNumSides(0);
}
void Circle::SetRadius(int radius) {
radius_ = radius;
}
int Circle::GetRadius() {
return radius_;
}
void Circle::SetFillColor(std::string fill_color) {
fill_color_ = fill_color;
}
std::string Circle::GetFillColor() {
return fill_color_;
}
void Circle::SetBorderColor(std::string border_color) {
border_color_ = border_color;
}
std::string Circle::GetBorderColor() {
return border_color_;
}
float Circle::Area() {
return M_PI * radius_ * radius_;
}
float Circle::Perimeter() {
return 2 * M_PI * radius_;
}
Test driver
#include
#include "Triangle.h"
#include "Circle.h"
int main() {
// Test Triangle
Triangle t;
t.SetSide1(3);
t.SetSide2(4);
t.SetSide3(5);
std::cout << "Triangle area: " << t.Area() << std::endl;
std::cout << "Triangle perimeter: " << t.Perimeter() << std::endl;
// Test Circle
Circle c;
c.SetRadius(5);
std::cout << "Circle area: " << c.Area() << std::endl;
std::cout << "Circle perimeter: " << c.Perimeter() << std::endl;
return 0;
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
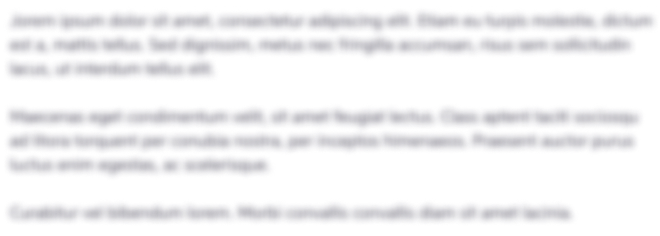
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started