Question
I want to make a C Program that replicates the gameplay of connect4 twist and turn. This version of connect4 allows for the rotation of
I want to make a C Program that replicates the gameplay of connect4 twist and turn. This version of connect4 allows for the rotation of one of the rows desired by the player during their turn. it needs to be memory efficient and use pointers and structures as well. the functions that should be included but not limited to are listed below:
1. struct board_structure is an appropriate structure (which you must devise) to hold the grid in memory. Note that board has been typdefed to be a pointer to such a structure.
2. setup_board() dynamically allocates a struct board_structure and returns a pointer to it. It also does any initial setup, if necessary. It does not assume any particular size of board.
3. cleanup_board(board u) frees the memory that u points at, along with any memory that was allocated inside u.
4. read_in_file(FILE *infile, board u) reads in the file from the file pointer infile and stores the grid in the board u, which has been previously set up by setup_board(). You must use dynamic memory allocation to set aside memory for storing the grid. (Hint: how will you work out how many cells are in each row? You may want to fscanf() with %c. Recall that fscanf() returns EOF when the file ends.)
5. write_out_file(FILE *outfile, board u) writes the content of the grid pointed to by u into the file from the file pointer outfile. If a player has four tokens in a line, this function should print those tokens in capitals (note that this could apply to both players). If a player has more than one line of four tokens, only one of them may be printed in capitals (choose one arbitrarily).
6. char next_player(board u) returns x or o if it is the corresponding players turn to play. Note that you can work out whose turn it is from what is on the board.
7. char current_winner(board u) returns x or o if the corresponding player has won the game on the board u and returns d if the game is a draw. Otherwise it returns ..
8. struct move read_in_move(board u) reads two integers representing a move from stdin. It does not need to check whether it is possible to make this move (see also is_valid_move()). This function must contain the following two lines, which can be found in the template connect4.c file: printf("Player %c enter column to place your token: ",next_player(u)); The function then reads in an integer from the user. Note that columns are numbered 1, 2, . . . from left to right. printf("Player %c enter row to rotate: ",next_player(u)); The function then reads in an integer r from the user. Note that rows are numbered 1, 2, . . . from bottom to top. (If r==0, this indicates that no row is to be rotated. If r>0, this indicates that row r is to be rotated one place to the right. If r<0, this indicates that row -r is to be rotated one place to the left.) The function must not modify the board u.
9. int is_valid_move(struct move m, board u) returns 1 if m is a valid move to make on the board uand 0 otherwise.
10. char is_winning_move(struct move m, board u) returns x or o if playing this move would cause the corresponding player to win. It returns d if playing the move would result in a draw. Otherwise it returns .. It does not actually play the move m.
11. void play_move(struct move m, board u) plays the move m and updates the board u correspondingly. You code should support having more than one board in memory at a time. You may add additional functions to connect4.c if you wish. You may not use any global variables. Consider appropriate error checking and make sure that your code can be used robustly. In case of any errors, print an appropriate message to stderr and exit the current program with a non-zero error code (e.g. use exit(1)). Try to make your code as memory-efficient as possible.
An example main.c file is included, it starts with the board specified in initial_board.txt, which contains a board of five rows and six columns with no tokens present.
.txt file:
........ ........ ........ ........ ........ ........
this program should be compilable through connect4.c and then running ./main
Step by Step Solution
There are 3 Steps involved in it
Step: 1
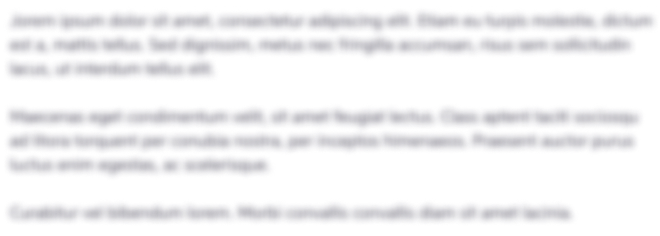
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started