Question
I'm a beginner in JAVA. Code the HumanResourcesTree class to implement a binary search tree using links. It will store data for employees (use the
I'm a beginner in JAVA.
Code the HumanResourcesTree class to implement a binary search tree using links. It will store data for employees (use the Employee class from assignment #1).
Define a node class that will have three data members (you may want to define constructors for the class):
- a data member of type Employee
- a data member (for the left link) of the node type
- a data member (for the right link) of the node type
The HumanResourcesTree class will have one data member to store the address (reference) of the root node. It will have the following instance methods:
public HumanResourcesTree() : Zero-parameter constructor to instantiate an empty tree
public HumanResourcesTree(HumanResourcesTree): Copy constructor (get a copy of it from Omnivox and adapt it to fit your program).
public isEmpty(): returns true if the tree is empty
public boolean addEmployee(Employee): adds an Employee to the binary search tree. It returns true if the Employee was added. It returns false if the Employee is already in the tree (same employee number).
public boolean deleteEmployee (String): delete the given Employee from the tree; your algorithm for deleting an employee should not unbalance the tree. It returns true if the Employee was deleted. It returns false if the Employee cannot be found in the tree.
public Employee findEmployee(String): Returns an Employee object if the employee with the given number is in the tree. It returns null otherwise.
public boolean changeDepartment(String, String): For the given employee (first parameter), change the department value to the given department (second parameter). It returns true if the employee department was changed, i.e. the employee was present in the list. It returns false otherwise.
public boolean adjustSalary (String, double): For the given employee (first parameter), adjust the salary value by adding the given amount (second parameter) to the existing salary. It returns true if the employee salary was adjusted; it returns false otherwise.
public String toString(): do an inorder traversal of the tree to set up a string with the Employees in increasing order. Each Employee should appear on a separate line.
NOTE: The code to search the tree for an employee to be able to either add, delete, find, change department and adjust salary should not be repeated in every method that needs to search for an employee. Define private methods.
To create a tree with a significant number of employees for testing, use the data in file Employees.txt (found on Omnivox). It has the following format (append the last name and first name to create the employee name):
Employee# Last Name First Name Department Salary
This is the employee class:
public class Employee { private String employeeNum; private String name; private String department; private double salary; /** * Zero parameter constructor */ public Employee() { employeeNum = ""; name = ""; department = ""; salary = 0.0; } /** * * @param employeeN * @param n * @param dept * @param sal */ public Employee(String employeeN, String n, String dept, double sal) { employeeNum = employeeN; name = n; department = dept; salary = sal; } /** * Copy constructor * @param copy */ public Employee(Employee copy) { employeeNum = copy.employeeNum; name = copy.name; department = copy.department; salary = copy.salary; } /** * Getter method for employee number * @return employeeNum */ public String getEmployeeNum() { return employeeNum; } /** * Getter method for employee name * @return name */ public String getName() { return name; } /** * Getter method for employee department * @return department */ public String getDepartment() { return department; } /** * Getter method for employee salary * @return salary */ public double getSalary() { return salary; } /** * Setter method for employee number * @param employeeNum */ public void setEmployeeNum(String employeeNum) { this.employeeNum = employeeNum; } /** * Setter method for employee name * @param name */ public void setName(String name) { this.name = name; } /** * Setter method for employee department * @param department */ public void setDepartment(String department) { this.department = department; } /** * Setter method for employee salary * @param salary */ public void setSalary(double salary) { this.salary = salary; } /** * * @param employeeNum * @param department * @param name * @param salary */ public void setEmployee(String employeeNum, String department, String name, double salary) { this.employeeNum = employeeNum; this.name = name; this.department = department; this.salary = salary; } /** * Method that returns the content of the list * @return String with the information of the list */ public String toString() { String str = String.format("Employee Number:%-6s Name:%-25s Department:%-15s Salary:%9.2f", employeeNum, name, department, salary); return str; } /** * Method that checks if employee number is equal to the parameter object * @param number * @return true if it is equal */ public boolean isEquals(Employee number) { String str = number.getEmployeeNum(); return this.employeeNum.equals(str); } /** * Method that compares employees * @param number * @return value */ public int compareTo(Employee number) { int compare; int value; String str = number.getEmployeeNum(); compare = str.compareTo(employeeNum); if(compare < 0) value = -1; else if(compare > 0) value = 1; else value = 0; return value; } }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
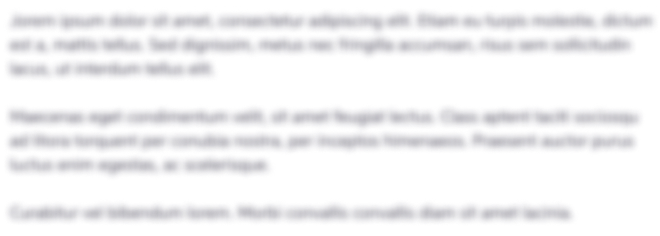
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started