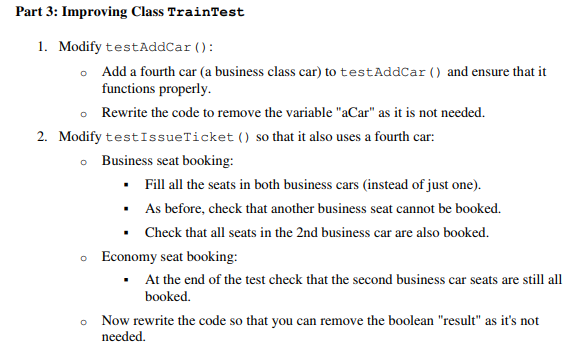
import java.util.ArrayList;
/** * The test class TrainTest. */ public class TrainTest extends junit.framework.TestCase { /** * Default constructor for test class TrainTest */ public TrainTest() { }
/** * Sets up the test fixture. * * Called before every test case method. */ protected void setUp() { }
/** * Tears down the test fixture. * * Called after every test case method. */ protected void tearDown() { } public void testCreateEmptyTrain() { Train aTrain = new Train(); /* Verify that a new train has no cars. */ assertEquals(0, aTrain.cars().size()); } public void testAddCar() { Train aTrain = new Train(); Car car1 = new Car(1250, true); aTrain.addCar(car1); Car car2 = new Car(1300, false); aTrain.addCar(car2); Car car3 = new Car(1740, false); aTrain.addCar(car3); ArrayList cars = aTrain.cars(); assertEquals(3, cars.size()); /* Verify that each car added to the train was placed at * the end of the list. */ Car aCar; aCar = cars.get(0); assertSame(car1, aCar); aCar = cars.get(1); assertSame(car2, aCar); aCar = cars.get(2); assertSame(car3, aCar); } public void testIssueTicket() { Train aTrain = new Train(); Car car1 = new Car(1250, true); aTrain.addCar(car1); Car car2 = new Car(1300, false); aTrain.addCar(car2); Car car3 = new Car(1740, false); aTrain.addCar(car3); boolean result; /* Book all the seats in the business-class car. */ for (int i = 0; i cars = aTrain.cars(); for (int i = 0; i cars = aTrain.cars(); result = aTrain.cancelTicket(1300, 4); assertTrue(result); assertFalse(cars.get(1).seats()[3].isBooked()); /* Cancel ticket in a non-existent car. */ result = aTrain.cancelTicket(1500, 7); assertFalse(result); /* Cancel ticket in a non-existent seat. */ result = aTrain.cancelTicket(1300, 54); assertFalse(result); /* Cancel ticket for a seat that hasn't been booked. */ result = aTrain.cancelTicket(1740, 21); assertFalse(result); assertFalse(cars.get(2).seats()[20].isBooked()); /* Attempt to cancel the same ticket twice. */ result = aTrain.cancelTicket(1250, 11); assertTrue(result); assertFalse(cars.get(0).seats()[10].isBooked()); result = aTrain.cancelTicket(1250, 11); assertFalse(result); assertFalse(cars.get(0).seats()[10].isBooked()); } }
import java.util.ArrayList; public class Train { /** The cars in this train. */ private ArrayList cars; /** * Constructs an empty train; i.e., one that has no cars. */ public Train() { cars = new ArrayList(); } /** * Adds the specified car to this train. */ public void addCar(Car car) { cars.add(car); } public ArrayList cars() { return cars; } public boolean issueTicket(boolean businessClassSeat) { for(Car car:cars) { if(businessClassSeat) { if(car.isBusinessClass()) { if(car.bookNextSeat()) { return true; } } } else { if(!car.isBusinessClass()) { if(car.bookNextSeat()) { return true; } } } } return false; } /** * Cancels the ticket for the specified seat in the specified car. * Returns true if successful, false otherwise. */ public boolean cancelTicket(int carId, int seatNo) { for (Car car : cars) { if (carId == car.id()) { return car.cancelSeat(seatNo); } } return false; } }
Part 3: Improving Class TrainTest 1. Modify testAddCar ): Add a fourth car (a business class car) to testAddCar() and ensure that it functions properly. o o Rewrite the code to remove the variable "aCar" as it is not needed. 2. Modify testIssueTicket () so that it also uses a fourth car o Business seat booking: Fill all the seats in both business cars (instead of just one). .As before, check that another business seat cannot be booked. .Check that all seats in the 2nd business car are also booked Economy seat booking: o At the end of the test check that the second business car seats are still all booked. Now rewrite the code so that you can remove the boolean "result" as it's not needed. o