Question
In C++ Inheritance Modification Assuming you have completed Programming Challenges 9 ( SearchableVector Modification ) and Programming Challenges 10 (S ortableVector Class Template ) ,
In C++
Inheritance Modification Assuming you have completed Programming Challenges 9 (SearchableVector Modification) and Programming Challenges 10 (SortableVector Class Template) , modify the inheritance hierarchy of the SearchableVector class template so it is derived from the SortableVector class instead of the SimpleVector class. Implement a member function named sortAndSearch , both a sort and a binary search.
Programming Challenges 9 (SearchableVector Modification
// SimpleVector class template #ifndef SIMPLEVECTOR_H #define SIMPLEVECTOR_H #include // for bad_alloc exception #include // for the exit function #include using namespace std;
template class SimpleVector { private: T *aptr; // To point to the allocated array int arraySize; // Number of elements in the array void memError(); // Handles memory allocation errors void subError(); // Handles subscripts out of range
public: // Default constructor SimpleVector() { aptr = 0; arraySize = 0; }
// Constructor declaration SimpleVector(int);
// Copy constructor declaration SimpleVector(const SimpleVector &);
// Destructor declaration ~SimpleVector();
// Accessor to return the array size int size() const { return arraySize; }
// Accessor to return a specific element T getElementAt(int position);
void setElementAt(int position, T value);
// Overloaded [] operator declaration T &operator[](const int &); };
//************************************************************ // Constructor for SimpleVector class. Sets the size of the * // array and allocates memory for it. * //************************************************************
template SimpleVector::SimpleVector(int s) { arraySize = s; // Allocate memory for the array. try { aptr = new T[s]; } catch (bad_alloc) { memError(); }
// Initialize the array. for (int count = 0; count < arraySize; count++) *(aptr + count) = 0; }
//******************************************* // Copy Constructor for SimpleVector class. * //*******************************************
template SimpleVector::SimpleVector(const SimpleVector &obj) { // Copy the array size. arraySize = obj.arraySize;
// Allocate memory for the array. aptr = new T[arraySize]; if (aptr == 0) memError();
// Copy the elements of obj's array. for (int count = 0; count < arraySize; count++) *(aptr + count) = *(obj.aptr + count); }
//************************************** // Destructor for SimpleVector class. * //**************************************
template SimpleVector::~SimpleVector() { if (arraySize > 0) delete[] aptr; }
//******************************************************** // memError function. Displays an error message and * // terminates the program when memory allocation fails. * //********************************************************
template void SimpleVector::memError() { cout << "ERROR:Cannot allocate memory. "; exit(EXIT_FAILURE); }
//************************************************************ // subError function. Displays an error message and * // terminates the program when a subscript is out of range. * //************************************************************
template void SimpleVector::subError() { cout << "ERROR: Subscript out of range. "; exit(EXIT_FAILURE); }
//******************************************************* // getElementAt function. The argument is a subscript. * // This function returns the value stored at the * // subcript in the array. * //*******************************************************
template T SimpleVector::getElementAt(int sub) { if (sub < 0 || sub >= arraySize) subError(); return aptr[sub]; }
template void SimpleVector::setElementAt(int sub, T value) { if (sub < 0 || sub >= arraySize) subError(); aptr[sub] = value; }
//******************************************************** // Overloaded [] operator. The argument is a subscript. * // This function returns a reference to the element * // in the array indexed by the subscript. * //********************************************************
template T &SimpleVector::operator[](const int &sub) { if (sub < 0 || sub >= arraySize) subError(); return aptr[sub]; }
#endif
/**** SearchableVector.h***/
#ifndef SEARCHABLEVECTOR_H
#define SEARCHABLEVECTOR_H
template
class SearchableVector : public SimpleVector{
public:
SearchableVector(int p) : SimpleVector(p){}
// this is copy constructor. Not required for this program. You can comment out in case needed
//SearchableVector(SearchableVector &);
SearchableVector(SimpleVector &object) : SimpleVector(object){}
int findItem(T);
};
/** copy Constructor ***/
/*
template
SearchableVector::SearchableVector(SearchableVector &object) : SimpleVector(object) {}
*/
// For binary Search
template
int SearchableVector::findItem(T item){
int start= 0;
int end = this->size() - 1;
int middle;
while(start <= end){
middle = (start + end)/2;
if(this->operator[](middle) == item)
return middle;
else if( this->operator[](middle) > item){
end = middle -1;
}
else{
start = middle +1;
}
}
return -1;
}
#endif
/**** SearchableVector.cpp ****/
#include
using namespace std;
int main() {
const int SIZE = 10; // Number of elements
int count; // Loop counter
int result; // To hold search results
// Create two SearchableVector objects.
SearchableVector intTable(SIZE);
SearchableVector doubleTable(SIZE);
// Store values in the objects.
for (count = 0; count < SIZE; count++) {
intTable[count] = (count * 2);
doubleTable[count] = (count * 2.14);
}
// Display the values in the objects.
cout << "These values are in intTable: ";
for (count = 0; count < SIZE; count++)
cout << intTable[count] << " ";
cout << endl << endl;
cout << "These values are in doubleTable: ";
for (count = 0; count < SIZE; count++)
cout << doubleTable[count] << " ";
cout << endl;
// Search for the value 6 in intTable.
cout << " Searching for 6 in intTable. ";
result = intTable.findItem(6);
if (result == -1)
cout << "6 was not found in intTable. ";
else
cout << "6 was found at subscript " << result << endl;
// Search for the value 12.84 in doubleTable.
cout << " Searching for 12.84 in doubleTable. ";
result = doubleTable.findItem(12.84);
if (result == -1)
cout << "12.84 was not found in doubleTable. ";
else
cout << "12.84 was found at subscript " << result << endl;
return 0;
}
Programming Challenges 10 (SortableVector Class Template)
// SimpleVector class template #ifndef SIMPLEVECTOR_H #define SIMPLEVECTOR_H #include
template
public: // Default constructor SimpleVector() { aptr = 0; arraySize = 0; }
// Constructor declaration SimpleVector(int);
// Copy constructor declaration SimpleVector(const SimpleVector &);
// Destructor declaration ~SimpleVector();
// Accessor to return the array size int size() const { return arraySize; }
// Accessor to return a specific element T getElementAt(int position);
void setElementAt(int position, T value);
// Overloaded [] operator declaration T &operator[](const int &); };
//************************************************************ // Constructor for SimpleVector class. Sets the size of the * // array and allocates memory for it. * //************************************************************
template
// Initialize the array. for (int count = 0; count < arraySize; count++) *(aptr + count) = 0; }
//******************************************* // Copy Constructor for SimpleVector class. * //*******************************************
template
// Allocate memory for the array. aptr = new T[arraySize]; if (aptr == 0) memError();
// Copy the elements of obj's array. for (int count = 0; count < arraySize; count++) *(aptr + count) = *(obj.aptr + count); }
//************************************** // Destructor for SimpleVector class. * //**************************************
template
//******************************************************** // memError function. Displays an error message and * // terminates the program when memory allocation fails. * //********************************************************
template
//************************************************************ // subError function. Displays an error message and * // terminates the program when a subscript is out of range. * //************************************************************
template
//******************************************************* // getElementAt function. The argument is a subscript. * // This function returns the value stored at the * // subcript in the array. * //*******************************************************
template
template
template
#endif
/*** sortableVector.h ***/
#ifndef SORTABLEVECTOR_H
#define SORTABLEVECTOR_H
#include
#include
template
class SortableVector : public SimpleVector
public:
SortableVector(int t) : SimpleVector
SortableVector(SimpleVector
void sortAscendingOrder();
};
template
void SortableVector
bool swaping;
do{
swaping = false;
for(int i =0; i< (this->size() - 1); i++){
if(this->operator[](i) > this->operator[](i+ 1)){
swap(this->operator[](i) , this->operator[](i+ 1));
swaping = true;
}
}
} while(swaping);
}
#endif
/*** sortableVector.cpp **/
#include
using namespace std;
int main(){
const int SIZE = 10;
SortableVector
SortableVector
int intArr[SIZE] = {56,1,45,2,23,87,14,0,5,3};
double doubleArr[SIZE] = {15.3, 23.1, 29.4, 22.25, 90.90, 11.14, 20.4, 80.4, 28.6, 21.7};
for(int i =0 ; i intVector[i] = intArr[i]; doubleVector[i] = doubleArr[i]; } cout<<"Before Sorting intVector is : "< for(int i=0; i< SIZE; i++){ cout< } cout< cout<<"After sorting doubleVector is: "< for(int i=0; i< SIZE; i++){ cout< } cout< cout< intVector.sortAscendingOrder(); doubleVector.sortAscendingOrder(); cout<<"After Sorting intVector is : "< for(int i=0; i< SIZE; i++){ cout< } cout< cout<<"After sorting doubleVector is: "< for(int i=0; i< SIZE; i++){ cout< } cout< return 0; } REPORTING FORMAT: Source Code & Testing Results
Step by Step Solution
There are 3 Steps involved in it
Step: 1
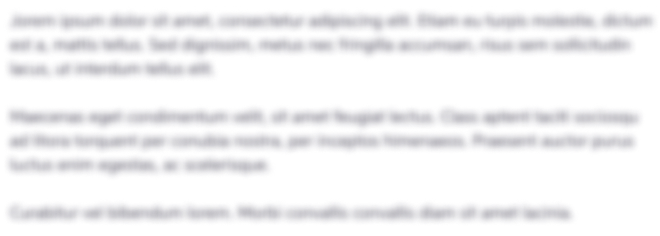
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started