Question
In this assignment, you will be making a program that reads from a file called classes.txt and displaying that information to the user. The goal
In this assignment, you will be making a program that reads from a file called classes.txt and displaying that information to the user. The goal for this assignment is to learn how to handle potential exceptions. Use the given template to implement exception-handling techniques.
Please note: You do not have to edit any parts of the code. Only handle exceptions where the code says /* INSERT CODE HERE */
The four exceptions you have to handle are:
NumberFormatException FileNotFoundException ArrayIndexOutOfBoundsException NoSuchFieldException
classes.txt:
14 CSCE155;3;3:00PM;;Computer Science I CSCE156;4;12:00PM;CSCE155;Computer Science II CSCE230;4;8:30AM;CSCE155;Computer Organization CSCE235;3;9:30AM;CSCE155;Introduction to Discrete Structures CSCE251;1;;CSCE155;Unix Programming Environment CSCE310;3;11:30AM;CSCE156;Data Structures and Algorithms CSCE322;3;4:30PM;CSCE156,CSCE230,CSCE311;Programming Language Concepts CSCE351;3;1:30PM;CSCE230,CSCE310,CSCE311 CSCE???;?;;; CSCE361;3;9:00AM;CSCE310,CSCE311;Software Engineering CSCE423;3;2:00PM;CSCE235,CSCE310,CSCE310;Design and Analysis of Algorithms CSCE428;3;4:30PM;CSCE235,CSCE310,CSCE311;Automata, Computation, and Formal Languages CSCE486;3;1:00PM;CSCE361;Computer Science Professional Development CSCE487;3;10:00AM;CSCE486;Computer Science Senior Design Project
import java.util.*;
import java.io.*;
import java.lang.*;
public class Main
{
public static void main(String[] args)
{
Scanner classesInput = null; // Reads course information from classes.txt
Scanner keyboard = new Scanner(System.in); // Reads input from user
/* INSERT CODE HERE */
try{
// Attempts to create scanner to read from classes.txt
classesInput = new Scanner(new File("classes.txt"));
}
catch(FileNotFoundException e){
}
String line; // Variable used to store each individual line
String[] parts; // Variable used to store the parts of each line (after splitting it)
// The first line of the text file has the number of courses
int numClasses = Integer.parseInt(classesInput.nextLine());
// Arrays to store information about each class
String[] courseNumbers = new String[numClasses];
int[] creditHours = new int[numClasses];
String[] times = new String[numClasses];
String[] prerequisites = new String[numClasses];
String[] courseNames = new String[numClasses];
// Read through all of the classes
for (int index = 0; index < numClasses; index++)
{
// Read one course at a time
line = classesInput.nextLine();
// The course information is split by semi-colons
parts = line.split(";");
// The course will always have a course number
courseNumbers[index] = parts[0];
try{
/* INSERT CODE HERE */
// Try to parse the credit hour amount
creditHours[index] = Integer.parseInt(parts[1]);
}
catch(NumberFormatException e) {
}
try{
times[index] = parts[2];
try{
if (times[index].equals(""))
{
// The times component is empty
throw new NoSuchFieldException();
}
}
catch(NoSuchFieldException e) {
}
/* INSERT CODE HERE */
prerequisites[index] = parts[3];
try{
if (prerequisites[index].equals(""))
{
// The prerequisites component is empty
throw new NoSuchFieldException();
}
}
catch(NoSuchFieldException e) {
}
/* INSERT CODE HERE */
courseNames[index] = parts[4];
}
catch(ArrayIndexOutOfBoundsException e){
}
}
/*Menu:
* P - Print out courses
* B - print all course names
* C - print all course numbers
* Q - Quit
*/
// Initialize 'input' to a known invalid value
char input = '?';
do
{
// Prompt the user
System.out.println("Choose an option: ");
// Read in the input, convert it to uppercase letters, then store ONLY the first letter
input = (keyboard.nextLine()).toUpperCase().charAt(0);
if (input == 'P')
// Print out all course information
printCourses(courseNumbers, creditHours, times, prerequisites, courseNames);
else if (input == 'B')
// Print out all course names
printCourseNames(courseNames);
else if (input == 'C')
// Print out all course numbers
printCourseNumbers(courseNumbers);
else if (input == 'Q')
{
// Quit the program --> It will quit on the while condition check
}
else
{
// User has input an invalid option
System.out.println("Invalid Option");
}
}
while(input != 'Q');
}
public static void printCourseNames(String[] names)
{
System.out.println("COURSE NAMES:");
for (int i = 0; i < names.length; i++)
{
// Print out the individual course name, one per line
System.out.println(names[i]);
}
System.out.println(); // Print empty line after course names
}
/*****************************************************************
* printCourseNumbers(...) takes in an array of course numbers and
* prints each course number on an individual line
*
* @param nums An array of course numbers that were read in
* from classes.txt --> Corresponds to courseNumbers from main(...)
*
* @return void Does not return any values
******************************************************************/
public static void printCourseNumbers(String[] nums)
{
System.out.println("COURSE NUMBERS:");
for (int i = 0; i < nums.length; i++)
{
// Print out the individual course numbers, one per line
System.out.println(nums[i]);
}
System.out.println(); // Print empty line after course numbers
}
/*****************************************************************
* printCourses(...) takes in all information for all courses and
* prints out each course's course number, coures name, credit
* hours, meeting time, and prerequisites
*
* @param num An array of course numbers that were read in
* from classes.txt --> Corresponds to courseNumbers from main(...)
*
* @param hours An array of course credit hours for each course
* that were read in from classes.txt --> Corresponds to
* creditHours from main(...)
*
* @param times An array of meeting times for each course that
* were read in from classes.txt --> Corresponds to times from
* main(...)
*
* @param pre An array of prerequisites for each course, with
* the format of prereq1,prereq2,prereq3,... that was read in from
* classes.txt --> Corresponds to prerequisities from main(...)
*
* @param names An array of course names that were read in from
* classes.txt --> Corresponds to courseNames from main(...)
*
* @return void Does not return any values
******************************************************************/
public static void printCourses(String[] num, int[] hours,String[] times, String[] pre, String[] names)
{
String hoursMessage;
String prereqMessage;
for (int i = 0; i < num.length; i++)
{
// Determine if the credit hours amount is valid
if (hours[i] == -1)
{
// Credit hours amount is invalid
hoursMessage = num[i] + " does not have any credit hours";
}
else
{
// Credit hours amount is valid
hoursMessage = Integer.toString(hours[i]);
}
// Format the prerequisites
String[] parts = pre[i].split(",");
StringBuilder prerequisitesBuilder = new StringBuilder();
String prerequisiteMessage = "";
for (int p = 0; p < parts.length; p++)
{
// Determine th number of prerequisites for each course
if (parts.length > 1)
{
// If there is more than one prerequisite
if (p < parts.length - 1)
{
// If it's not the last prerequisite
prerequisitesBuilder.append(parts[p]); // Add the prerequisite to the string
prerequisitesBuilder.append(", "); // Add a comma to separate the courses
}
else
{
// If it is the last prerequisite
prerequisitesBuilder.append("and ");
prerequisitesBuilder.append(parts[p]); // Add the last prerequisite
}
}
else
{
// If it is the only prerequisite
prerequisitesBuilder.append(parts[p]);
}
System.out.println("Does not have any requisite");
}
// Store the prerequisite information to print later
prerequisiteMessage = prerequisitesBuilder.toString();
// Print the course information
System.out.println("Course Number: " + num[i]);
System.out.println("Name: " + names[i]);
System.out.println("Credit Hours: " + hoursMessage);
System.out.println("Time: " + times[i]);
System.out.println("Prereqs : " + prerequisiteMessage);
System.out.println(); // Print empty line after each course
}
}
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
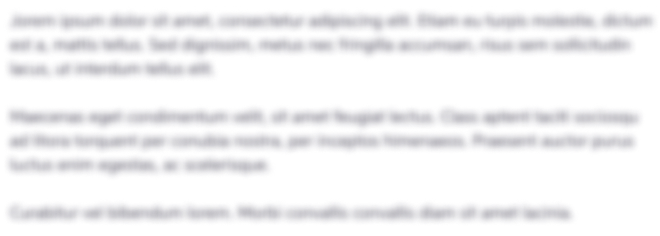
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started