Question
In this assignment you will develop in Python a game of cards called Blackjack. Some of the code is provided (link in Module 7) and
In this assignment you will develop in Python a game of cards called Blackjack. Some of the code is provided (link in Module 7) and you will write the parts that are missing. You need to add code to two of the functions in Python:
- compareHands: this function compares the player and house hands to determine if there is a winner or loser. Someone loses if their hand goes over 21. In this case, a messages should be printed to inform the player who lost or won. Reaching exactly 21 is a blackjack win.
- blackjack: this is starting point of your program and should have the main logic of the game playing. It needs to coordinate well with compareHands, which determines if there is a winner or loser, so the game can end. The player may decide to walk away at any point. In that case, whoever has the highest hand (player or house) wins the game.
All the places where you should write your own code are marked with #####
This is the code provided:
import random
def shuffledDeck(): 'returns shuffled deck' # suits is a set of 4 Unicode symbols: back spade and club, # and white diamond and heart suits = {'\u2660', '\u2661', '\u2662', '\u2663'} ranks = {'A','2','3','4','5','6','7','8','9','10','J','Q','K'} deck = []
# create deck of 52 cards for suit in suits: for rank in ranks: # card is the concatenation deck.append(rank+' '+suit) # of suit and rank
# shuffle the deck and return random.shuffle(deck) return deck
def dealCard(deck, participant): 'deals single card from deck to participant' card = deck.pop() participant.append(card) return card
def total(hand): 'returns the value of the blackjack hand' values = {'2':2, '3':3, '4':4, '5':5, '6':6, '7':7, '8':8, '9':9, '1':10, 'J':10, 'Q':10, 'K':10, 'A':11 } result = 0
# add up the values of the cards in the hand for card in hand: result += values[card[0]] return result
def compareHands(house, player): 'compares house and player hands and prints outcome'
# compute house and player hand total houseTotal, playerTotal = total(house), total(player)
##### your code must: ##### compare player and house hand totals to determine who wins or loses ##### this function should print: ##### 'You lose' or 'You win' ##### The player or house loses if they get over 21. ##### return True if nobody reached 21 yet, meaning the game can continue
def myblackjack(): 'simulates the house in a game of blackjack'
deck = shuffledDeck() # get shuffled deck print("Deck: ") print(deck) house = [] # house hand player = [] # player hand
dealCard(deck, player) # deal to player first dealCard(deck, house) # deal to house second
answer = input('Hit or stand? (default: hit): ') while compareHands(house, player) and answer in {'', 'h', 'hit'}: ##### The code in here should have the logic of the game. ##### You should draw a card from the player's hand ##### and a card from the house, ##### compare the hands and see if there is a winner. ##### If the user wants to stop, whoever has the highest hand wins
answer = input('Hit or stand? (default: hit): ')
# The following starts the game: if __name__ == '__main__': myblackjack()
Feel free to change whatever you need in compareHands and blackjack functions. I don't recommend changing anything else. Just make sure you understand what the other functions do.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
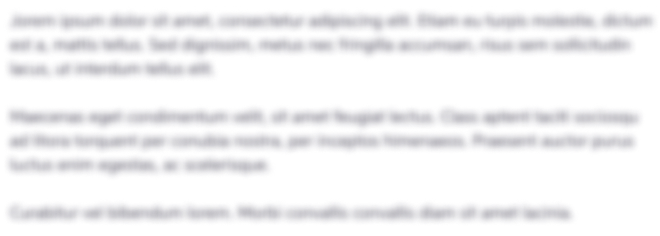
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started