Question
In this lab you will write a spell check program. The program has two input files: one is the dictionary (a list of valid words)
In this lab you will write a spell check program. The program has two input files: one is the dictionary (a list of valid words) and the other is the document to be spellchecked. The program will read in the words for the dictionary, then will read the document and check whether each word is found in the dictionary. If not, the user will be prompted to leave the word as is or type in a replacement word and add the replacement word to the dictionary.
Dictionary
The Dictionary will have a field to hold the words; the type of this field will be an ArrayList of Strings.
Create the following interface and use it when implementing the Dictionary class.
public interface DictionaryInterface { void addWord(String word); //adds a new word to this dictionary boolean isWord(String word); //returns true if word is in the dictionary and false otherwise int getSize(); //number of words in the dictionary String toString(); }
The toString() method should return a String containing all the words in the Dictionary, with each word on a separate line.
Create a constructor with one parm, a Scanner for the file containing the words for the dictionary. The constructor will read the words using the Scanner and store them in the Dictionary. Remember there is one word on each line of the dictionary file.
About Spellchecking
To spellcheck the file, your program will read each line of the document. It will compare every word in the input line with the words in the dictionary.
Words in the document will be separated by white space, and it is possible that there will be more than one whitespace character separating the words. Use the split method to access the individual words in the input file. Any white space or punctuation at the beginning or end of a word should be removed, and the lower case version of the word should be checked against the dictionary. More information on this is found below.
Before looking up a word in the dictionary it must be cleaned up: it must be converted to lowercase, and any punctuation mark at the end of the word (like . , ! or ?) must be removed. More information on this is found below.
After it is spellchecked, each word should be written to an output file called results.txt. Don't put each word on a separate line; the arrangement of words on a line should be the same in the document and in results.txt. The difference between the document and results.txt is that in results.txt each word is in lowercase and there is no punctuation. For example, suppose the document contains the following:
The Hunter Moon waxed round in the night sky, and put to flight all the lesser stars. But low in the South one star shone red.
Then results.txt will contain:
the hunter moon waxed round in the night sky and put to flight all the lesser stars but low in the south one star shone red
If a word is not found in the dictionary, the program will print a message indicating that the word was not found. Then it will ask the user whether to add the word to the dictionary, whether the word should be changed, or whether processing should continue with no change.
For example, suppose the text file contains the word "yikes", and "yikes" is not in the ditionary:
yikes not found in dictionary 1 : replace in text file 2 : add to dictionary 3 : continue ==> 1 enter word to replace yikes ==> yippee [yippee will appear in results.txt instead of yikes yippee will be added to the dictionary if it is not already there] yikes not found in dictionary 1 : replace in text file 2 : add to dictionary 3 : continue ==> 2 yikes added to dictionary [yikes will appear in results.txt] yikes not found in dictionary 1 : replace in text file 2 : add to dictionary 3 : continue ==> 3 [yikes will appear in results.txt and the dictionary is unchanged]
In the first case, yikes will be replaced by yippee in the output file results.txt. In the second case yikes will appear in results.txt and it will be added to the dictionary.
The Spellcheck Class
The Dictionary is a field in the Spellcheck class.
Spellcheck will have a method to perform the spellchecking: read each line of the file, extract each word, clean it up (remove any punctuation at the end of the word), and see if it is in the dictionary. It also needs to handle the processing for words that are not found. Do not write one giant method to do all the spellchecking! Create some private methods that the spellcheck method will call to do parts of the processing.
Reading words from the input file: The spellcheck method will read a line from the input file and store it in a String. Each line will contain an unknown number of words, separated by one or more whitespace characters. To separate the words within the input line, we will use the split method in the String class. Look up the String class and read about the split method. You may not have heard of regular expressions, so don't worry if that part is confusing.
The split method (String[] split(String regex)) separates the items (called "tokens") in a String and stores them in an array of Strings. The tokens can be separated by whitespace, commas, slashes, or any other character(s). One of the parms to split specifies how to separate the tokens. In our input the tokens are words and they are separated by one or more whitespace characters. The value we will use to specify how to separate is "\\s+". Here is an explanation of "\\s+": '\s' represents any whitespace character. The extra \ indicates that '\s' represents one character, not the character '\' followed by the character 's'. The + means "one or more", so all together "\\s+" means "one or more whitespace character. When we call split with this parm, it returns an array of Strings where each element of the array is one word of this.
Cleaning up words before spellchecking: There is a String method that returns a lowercase version of a String. You can use other String and Character methods to check whether the last character of a word is a letter, and if not, create a String with that character removed.
Client Code
The main method will prompt for the name of the file containing the dictionary and the name of the input file to be spellchecked. Create the Scanner for the dictionary file and pass it to the Dictionary constructor. Create the Scanner for the input file, perform the spellcheck, and write out the updated dictionary.
Use exception handling in the main method to ensure the input files were found and opened properly. If not handle the situation by prompting the user again for the correct file name.
Do not use any set or get methods.
- If user chooses to change the word, then the user will be prompted for the new word and the new word will replace the original word in results.txt. If the new word is not in the dictionary, it will be added to the dictionary.
- If the user chooses to add the word to the dictionary, the word is added to the dictionary and it is not changed in results.txt.
- If the user chooses to continue, no change is made. The word is unchanged and the dictionary is unchanged.
Java code please and Reading words from the input file: The spellcheck method will read a line from the input file and store it in a String. Each line will contain an unknown number of words, separated by one or more whitespace characters. To separate the words within the input line, we will use the split method in the String class.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
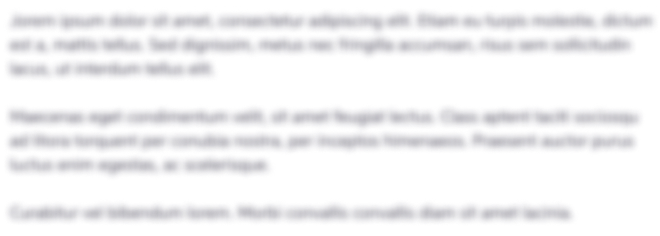
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started