Question
Instructions: Create an application, called BicyclePolyTest, which will be used to create a base class called Bicycle and two derived classes called MountainBike and RoadBike.
Instructions: Create an application, called BicyclePolyTest, which will be used to create a base class called Bicycle and two derived classes called MountainBike and RoadBike.
For class Bicycle:
Create a class called Bicycle. This class should contain:
* three protected instance variables:
- cadence: int
- gear: int
- speed: int
* named constructor accepting three parameters, for initializing values for the three instance variables
* method setCadence for setting the instance variable cadence
* method setGear for setting the instance variable gear
* method applyBrake for decrementing the speed by a parameter to the method.
* method speedUp for incrementing the speed by a parameter to the method.
* method printDescription for outputting the values of the three instance variables
For class MountainBike:
Create a class called MountainBike which uses Bicyle as a base class. This class should contain:
* one private instance variable
- suspension: String
* named constructor accepting one parameter, for initializing values of the base class and the local instance variable
* method getSuspension for retrieving the instance variable suspension
* method setSuspension for setting the instance variable suspension
* method printDescription for calling the printDescription() in the base class as well as output information regarding the suspension of the MountainBike instance variable.
For class RoadBike:
Create a class called RoadBike which uses Bicyle as a base class. This class should contain
* one private instance variable
- tireWidth: int
* named constructor accepting one parameter, for initializing values of the base class and the local instance variable
* method getTireWidth for retrieving the instance variable tireWidth
* method setTireWidth for setting the instance variable tireWidth
* method printDescription for calling the printDescription() in the base class as well as output information regarding the suspension of the RoadBike instance variable.
------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------
In your application BicyclePolyTest(), create three instance variables of super class Bicycle; bike1, bike2 and bike3.
bike1 should be instantiated from class Bicycle.
bike2 should be instantiated from MountainBike.
bike3 should be instantiated from RoadBike.
Each object should call their respective printDescription method
----------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------
Test Harness
You may use the following test harness to test your implementation.
public class BicycleTest
{
public static void main(String[] args)
{
Bicycle bike1, bike2, bike3, bike4;
bike1 = new Bicycle( 20, 10, 1 );
bike2 = new MountainBike( 20, 10, 5, "Dual" );
bike3 = new RoadBike( 40, 20, 8, 23 );
bike1.printDescription();
bike2.printDescription();
bike3.printDescription();
}
}
------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------
will result in the following output:
Bike is in gear 1 with a cadence of 20 and travelling at a speed of 10.
Bike is in gear 5 with a cadence of 20 and travelling at a speed of 10.
The MountainBike has a Dual suspension
Bike is in gear 8 with a cadence of 40 and travelling at a speed of 20.
The RoadBike has 23 MM tires
Step by Step Solution
There are 3 Steps involved in it
Step: 1
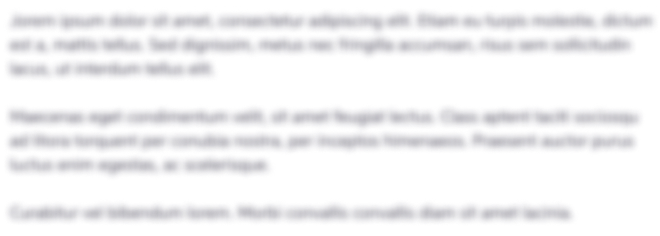
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started