Answered step by step
Verified Expert Solution
Question
1 Approved Answer
Java please help, Lost on this assignment Please show OutPut, Write a program that reads a Java source file and produces an index of all
Java please help, Lost on this assignment
Please show OutPut,
Write a program that reads a Java source file and produces an index of all identifiers in the file. For each identifier, print all lines in which it occurs. For simplicity, we will consider ech string consisting only of letters, numbers, and underscores an identifer. Declare a Scanner infor reading from the source file and call in .useDeliniter("^A-Za-z0-9_]+"). Then each call to next returns an identifier.
Here's the Code i have, AirportTable:
import java.util.ArrayList; import java.util.Collection; import java.util.HashMap; import java.util.LinkedList; import java.util.List; import java.util.Map; import java.util.Set; import java.util.SortedMap; import java.util.TreeMap; public class AirportTable { private MapcodeMap; private Map > stateMap; private Map > cityMap; private SortedMap nameMap; public AirportTable() { codeMap = new HashMap (); stateMap = new HashMap >(); cityMap = new HashMap >(); nameMap = new TreeMap (); } public boolean insert( Airport airport ) { if( airport == null ) { return false; } String code = airport.getCode(); String name = airport.getName(); String city = airport.getCity(); String state = airport.getState(); if( code == null || city == null || state == null ) { return false; } if( name == null ) { name = city + " " + state; } code = code .toUpperCase(); name = name.toUpperCase(); city = city.toUpperCase(); state = state.toUpperCase(); codeMap.put(code, airport); List stateList = stateMap.get(state); if( stateList == null ) { // State is not in the table stateList = new LinkedList (); stateMap.put(state, stateList); } stateList.add(airport); CityKey cityKey = new CityKey( city, state ); List cityList = cityMap.get(cityKey); if( cityList == null ) { // State is not in the table cityList = new LinkedList (); cityMap.put(cityKey, cityList); } cityList.add(airport); nameMap.put(name, airport); return true; } public Airport findByCode( String code ) { if( code == null ) { return null; } Airport airport = codeMap.get(code.toUpperCase()); return airport; } public Set allCodes() { Set codes = codeMap.keySet(); return codes; } public Collection findByState( String state ) { if( state == null ) { return null; } Collection retList = null; List list = stateMap.get(state.toUpperCase()); if( list != null ) { retList = new ArrayList(list); } return retList; } public Set allStates() { Set states = stateMap.keySet(); return states; } public int countByState( String state ) { if( state == null ) { return -1; } int count = 0; List list = stateMap.get(state.toUpperCase()); if( list != null ) { count= list.size(); } return count; } public Collection findByCity( String city, String state ) { if( city == null || state == null ) { return null; } Collection retList = null; CityKey cityKey = new CityKey( city.toUpperCase(), state.toUpperCase()); List list = cityMap.get(cityKey); if( list != null ) { retList = new ArrayList(list); } return retList; } // public Set allCities() { // Set states = stateMap.keySet(); // return states; // } public int countByCity( String city, String state ) { if( city == null || state == null ) { return -1; } int count = 0; CityKey cityKey = new CityKey( city.toUpperCase(), state.toUpperCase()); List list = cityMap.get(cityKey); if( list != null ) { count= list.size(); } return count; } public Collection findByName( String name ) { if( name == null ) { return null; } Collection retList = null; String fromNameKey = name.toUpperCase() + Character.MIN_VALUE; String toNameKey = name.toUpperCase() + Character.MAX_VALUE; SortedMap map = nameMap.subMap(fromNameKey, toNameKey); if( map != null ) { retList = map.values(); } return retList; } private class CityKey implements Comparable { private String city; private String state; public CityKey() { this("",""); } public CityKey( String city, String state ) { this.city = city; this.state = state; } public int compareTo( CityKey other ) { int ret = city.compareTo(other.city); if( ret == 0 ) { ret = state.compareTo(other.state); } return ret; } @Override public boolean equals( Object object ) { if( this == object ) { return true; } if( object == null ) { return false; } if(getClass() != object.getClass()) { return false; } CityKey other = (CityKey) object; return compareTo(other) == 0; } @Override public int hashCode() { int hash = 0; if( city != null ) { hash += 7 * city.hashCode(); } if( state != null ) { hash += 11 * state.hashCode(); } return hash; } } }
Here's the other code, AirportTester:
import java.io.FileNotFoundException; import java.io.IOException; import java.util.Collection; import java.util.Map; import java.util.Scanner; import java.util.Set; import java.util.TreeMap; public class AirportTester { public static void main( String[] args ) { CollectionairportData = loadAirportData( "us_airports.csv"); displayAirportData(airportData); AirportTable airportTable = buildAirportTable( airportData ); Scanner inp = new Scanner( System.in ); System.out.print("command> "); while( inp.hasNextLine()) { String line = inp.nextLine(); if(line == null || line.isEmpty()) { continue; } String[] param = line.split(" "); if( param.length <= 1 ) { continue; } String command = param[0].toLowerCase(); if("findbycode".equals(command)) { findByCode( airportTable, param[1] ); } else if("findbystate".equals(command)) { findByState( airportTable, param[1] ); } else if("findbyname".equals(command)) { findByName( airportTable, param[1] ); } else if("findbycity".equals(command)) { findByCity( airportTable, param[1], param[2]); } else if( "countbystate".equals(command)) { countByState( airportTable, param[1] ); } else if( "quit".equals(command)) { break; } System.out.println(); System.out.print("command> "); } } private static Collection loadAirportData( String filename ) { Collection airportData = null; try { airportData = AirportDataLoader.load(filename); } catch (FileNotFoundException e ) { System.out.println( e.getMessage()); } catch( IOException e ) { System.out.println( e.getMessage()); } return airportData; } private static AirportTable buildAirportTable( Collection airportData ) { AirportTable airportTable = new AirportTable(); for( Airport airport : airportData ) { boolean inserted = airportTable.insert(airport); if( ! inserted ) { System.out.printf( "Insert Failed: %d%n", airport); } } return airportTable; } private static void countByState( AirportTable airportTable, String state) { Collection list = null; if( !"*".equals(state) ) { int count = airportTable.countByState(state); System.out.printf("%d airports%n", count); } else { Set states = airportTable.allStates(); Map stateCounts = new TreeMap (); int total = 0; for( String stateAbbrev : states ) { int count = airportTable.countByState(stateAbbrev); total += count; stateCounts.put(stateAbbrev, count); } int perLineCount = 0; Set sortedStates = stateCounts.keySet(); for( String stateAbbrev : sortedStates ) { System.out.printf(" %s %4d", stateAbbrev, stateCounts.get(stateAbbrev).intValue()); ++ perLineCount; if( perLineCount % 4 == 0) { System.out.println(); perLineCount = 0; } } System.out.println(); } } private static void findByCity( AirportTable airportTable, String city, String state) { Collection list = airportTable.findByCity(city, state); if( list == null ) { System.out.println("No airports found"); } else { displayAirportData( list ); } } private static void findByCode( AirportTable airportTable, String code ) { Airport airport = airportTable.findByCode(code); if( airport == null ) { System.out.println("No airports found"); } else { System.out.println(airport); } } private static void findByName( AirportTable airportTable, String name ) { Collection list = airportTable.findByName(name); if( list == null ) { System.out.println("No airports found"); } else { displayAirportData( list ); } } private static void findByState( AirportTable airportTable, String state) { Collection list = airportTable.findByState(state); if( list == null ) { System.out.println("No airports found"); } else { displayAirportData( list ); } } private static void displayAirportData(Collection airportData) { for( Airport airport : airportData ) { System.out.println(airport); } } }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
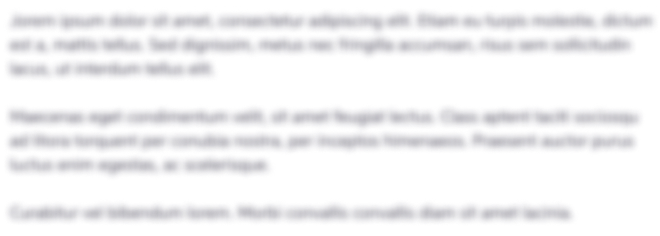
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started