Question
Java Project/Class name: Craps.java You will write a program to play craps, in a loop. You will keep a running total of the number of
Java Project/Class name: "Craps.java"
You will write a program to play craps, in a loop. You will keep a running total of the number of rounds won, and rounds lost. Your program should ask the user (with getInt) for the number of rounds to play. The program will then play that many rounds of craps.
When your program finishes, it will print out wins, how many losses, and the percentage of wins.
The methods you will write:
1. getInt(String prompt) returns an int. Function: prompt the user with the prompt argument, and let the user type in a number. Make sure to handle improper input gracefully. A post-test loop is suggested.
2. rollPairOfDice() returns an int in the range of 2-12. Should call rollA_Die
3. rollA_Die() returns an int in the range of 1-6.
4. comeOutRoll() returns an int. If the int is 1, it indicates that the player won the round by throwing a 7 or an 11 (see below for craps rules). If the int is 0, it indicates that the player lost the round by throwing a 2, 3, or 12. If the int is 4, 5, 6, 8, 9 or 10, it indicates the point that the player must try to make.
5. tryToMakeThePoint(int point) returns an int. This routine will repeatedly throw the virtual pair of dice until either the point is thrown (in which case, the int returned will be a 1, indicating that the player won the round), or a seven is thrown (in which case, the int returned will be a 0).
6. reportTheResults(int wins, int rounds) returns nothing. Prints out a summary of how many rounds were played, how many wins, how many losses, and the percentage of wins formatted to look like (for example) 49.33%, with two digits after the decimal points.
You will need to use Math.random() to generate random numbers. It will generate (double) numbers in the interval [0,1). You can turn this number into an integer in the 1 to 6 range by multiplying the random number by 6, and then "casting" it to an integer (this will throw away the fractional part, and result in a number in the interval [0,5]). Then add 1, to get a number in the interval [1,6]. (You could also use Math.ceil to do this - GIYF)
In each round, the player rolls the pair of dice on a "come-out roll".
If the come-out roll is a 7 or 11, then the player wins. This scenario ends the round. If the come-out roll is a 2, 3 or 12, also known as craps, the player loses. This also ends the round. If the come-out roll is a 4, 5, 6, 8, 9 or 10, then that specific number becomes the player's point. The shooter continues to roll the dice until he or she rolls the point number or a 7. If the point number is rolled, then the player wins. If a 7 is rolled, player loses.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
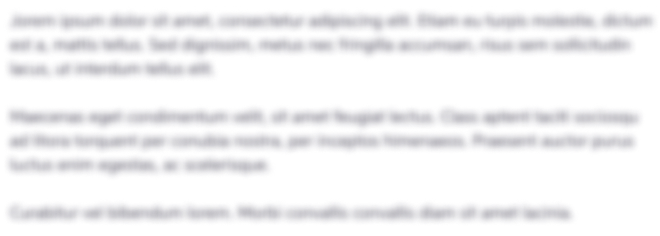
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started