Question
Java Write a method for the class BinaryTree that determines whether an object is in the tree and is a leaf (returns false if the
Java
Write a method for the class BinaryTree that determines whether an object is in the tree and is a leaf (returns false if the object is not in the tree or if the object is in the tree but on a node that is not a leaf).
The method header is: public boolean containsAsLeaf(T anObject)
Hint: think recursively and write a private helper method that takes a parameter of type BinaryNodeInterface
package TreePackage;
/**
* An interface for a node in a binary tree.
*
* @author Frank M. Carrano
* @version 2.0
*/
interface BinaryNodeInterface
/**
* Task: Retrieves the data portion of the node.
*
* @return the object in the data portion of the node
*/
public T getData();
/**
* Task: Sets the data portion of the node.
*
* @param newData
* the data object
*/
public void setData(T newData);
/**
* Task: Retrieves the left child of the node.
*
* @return the node that is this nodes left child
*/
public BinaryNodeInterface
/**
* Task: Retrieves the right child of the node.
*
* @return the node that is this nodes right child
*/
public BinaryNodeInterface
/**
* Task: Sets the nodes left child to a given node.
*
* @param leftChild
* a node that will be the left child
*/
public void setLeftChild(BinaryNodeInterface
/**
* Task: Sets the nodes right child to a given node.
*
* @param rightChild
* a node that will be the right child
*/
public void setRightChild(BinaryNodeInterface
/**
* Task: Detects whether the node has a left child.
*
* @return true if the node has a left child
*/
public boolean hasLeftChild();
/**
* Task: Detects whether the node has a right child.
*
* @return true if the node has a right child
*/
public boolean hasRightChild();
/**
* Task: Detects whether the node is a leaf.
*
* @return true if the node is a leaf
*/
public boolean isLeaf();
/**
* Task: Counts the nodes in the subtree rooted at this node.
*
* @return the number of nodes in the subtree rooted at this node
*/
public int getNumberOfNodes();
/**
* Task: Computes the height of the subtree rooted at this node.
*
* @return the height of the subtree rooted at this node
*/
public int getHeight();
/**
* Task: Copies the subtree rooted at this node.
*
* @return the root of a copy of the subtree rooted at this node
*/
public BinaryNodeInterface
} // end BinaryNodeInterface
Step by Step Solution
There are 3 Steps involved in it
Step: 1
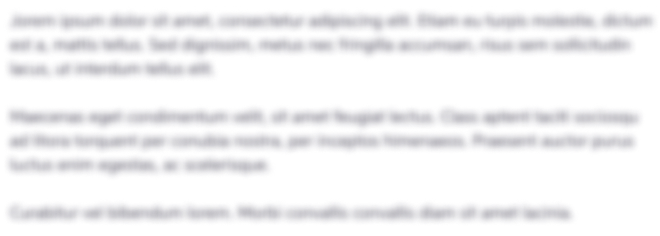
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started