Question
listSearch: In this assignment you are to implement a singly linked list that stores pointers to Person instances. You will implement two classes Node and
listSearch:
In this assignment you are to implement a singly linked list that stores pointers to Person instances.
You will implement two classes Node and LinkedList and two functions listSearch() and listDescriptor().
Instructions (Person.h)
Person
This class is given. It is used to store the personal information of a person (id, first, last).
Instructions (SinglyLinkedList.h)
Inside SinglyLinkedList.h define the two classes Node and LinkedList.
Node
Consists of the public members:
- person - a pointer to a Person instance
- next - a pointer to a Node instance
- Node() - an argument constructor that accepts a pointer to a Person instance. Sets the person field to the parameter.
Initialize both members to nullptr at declaration time.
LinkedList
Consists of the public members:
- head - a pointer to a Node instance
- tail - a pointer to a Node instance
Initialize both members to nullptr at declaration time.
Instructions (main.cpp)
Inside main.cpp define the two functions:
Node * listSearch(LinkedList * list, const int key);
- This function searches the list for the person with an id of id. If found it returns a pointer to its node, else nullptr.
string listDescriptor(LinkedList * list);
- This function builds a string containing all the visited nodes' data, i.e. a string containing all the Person records. Use an ostringstream instance to output each person's description by using the descriptor() method of the Person class. Each record should be newline terminated.
Example:
For the list containing:
{1,"John","Doe"}, {2,"Jane","Doe"}, {3,"Paul","Harvey"}
listSearch(list, 3) returns the third node in the list.
listSearch(list, 5) returns nullptr.
while listDescriptor(list) returns:
{id:1, first:John, last:Doe} {id:2, first:Jane, last:Doe} {id:3, first:Paul, last:Harvey}
If the list is empty there is no output.
CODE:
#ifndef SINGLY_LINKED_LIST #define SINGLY_LINKED_LIST
#include "Person.h" using namespace std;
/* class: Node */ /* Type your code here. */
/* class: LinkedList */ /* Type your code here. */
#endif
________________________________
// File: main.cpp
#include "SinglyLinkedList.h" #include
Node * listSearch(LinkedList * list, const int key); string listDescriptor(LinkedList * list);
int main() { cout << "No implementation given."; return 0; }// end main()
Node * listSearch(LinkedList * list, const int key) { /* Type your code here. */
}
string listDescriptor(LinkedList * list) { /* Type your code here. */ }
_______________________________________
#ifndef PERSON_H #define PERSON_H
#include
/* class: Person * Stores personal information */ class Person { public: int id; string first; string last;
Person(const int id = 0, const string& first = "na", const string& last = "na") : id{id}, first{first}, last{last} {}
string descriptor() const { ostringstream oss;
oss << "{" << "id:" << id << ", " << "first:" << first << ", " << "last:" << last << "}"; return oss.str(); } };
#endif
Step by Step Solution
There are 3 Steps involved in it
Step: 1
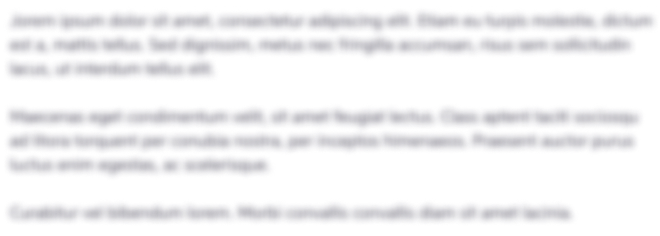
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started