Question
Main import java.io.*; import java.util.ArrayList; import java.util.Collections; import java.util.List; import java.util.Scanner; public class Compile { public static class Work { public static void main(String[] args)
Main
import java.io.*;
import java.util.ArrayList;
import java.util.Collections;
import java.util.List;
import java.util.Scanner;
public class Compile
{
public static class Work {
public static void main(String[] args) throws IOException {
Scanner scanner = new Scanner(System.in);
File inputFile = null;
File outputFile = null;
System.out.println("Please enter input file name:");
while (true) {
String fileName = scanner.nextLine();
inputFile = new File(fileName);
if (inputFile.exists()) {
break;
} else {
System.out.println(fileName + " does not exist. Please enter a valid file name.");
}
}
System.out.println("Please enter output file name:");
String fileName = scanner.nextLine();
outputFile = new File(fileName);
outputFile.createNewFile();
List
BufferedReader bufferedReader = new BufferedReader(new InputStreamReader(new FileInputStream(inputFile)));
String line = null;
while ((line = bufferedReader.readLine()) != null) {
String[] temp = line.split(" ");
for (String word : temp) {
words.add(word);
}
}
List
long start = System.currentTimeMillis();
BubbleSort.doSorting(bubbleSortWords);
long bubbleSortTime = System.currentTimeMillis() - start;
List
start = System.currentTimeMillis();
BubbleSort.doSorting(selectionSortWords);
long selectionSortTime = System.currentTimeMillis() - start;
List
start = System.currentTimeMillis();
MergeSort.doSorting(mergeSortWords, 0, mergeSortWords.size() - 1);
long mergeSortTime = System.currentTimeMillis() - start;
start = System.currentTimeMillis();
Collections.sort(words);
long javaSortTime = System.currentTimeMillis() - start;
FileOutputStream fileOutputStream = new FileOutputStream(outputFile, false);
fileOutputStream.write(("Total Words: " + words.size() + " ").getBytes());
fileOutputStream.write(("Bubble Sort time: " + bubbleSortTime + " ").getBytes());
fileOutputStream.write(("Selection Sort time: " + selectionSortTime + " ").getBytes());
fileOutputStream.write(("Merge Sort time: " + mergeSortTime + " ").getBytes());
fileOutputStream.write(("Java Sort time: " + javaSortTime + " ").getBytes());
fileOutputStream.close();
}
}
}
Classes:
public class SelectionSort
{
static void doSorting(List
for (int i = 0; i
int minIndex = i;
for (int j = i + 1; j
if (words.get(j).compareTo(words.get(minIndex))
minIndex = j;
}
}
String temp = words.get(minIndex);
words.set(minIndex, words.get(i));
words.set(i, temp);
}
}
}
import java.util.List;
public class MergeSort
{
static void doSorting(List
if (left
int middle = (left + right) / 2;
doSorting(words, left, middle);
doSorting(words, middle + 1, right);
merge(words, left, middle, right);
}
}
private static void merge(List
int leftSize = middle - left + 1;
int rightSize = right - middle;
String leftArr[] = new String[leftSize];
String rightArr[] = new String[rightSize];
for (int i = 0; i
leftArr[i] = words.get(left + i);
}
for (int j = 0; j
rightArr[j] = words.get(middle + j + 1);
}
int i = 0, j = 0;
int k = left;
while (i
if (leftArr[i].compareTo(rightArr[j])
words.set(k, leftArr[i]);
i++;
} else {
words.set(k, rightArr[j]);
j++;
}
k++;
}
while (i
words.set(k, leftArr[i]);
i++;
k++;
}
while (j
words.set(k, rightArr[j]);
j++;
k++;
}
}
}
import java.util.List;
public class BubbleSort {
static void doSorting(List
for (int i = 0; i
for (int j = 0; j
if (words.get(j).compareTo(words.get(j + 1)) > 0) {
String temp = words.get(j);
words.set(j, words.get(j + 1));
words.set(j + 1, temp);
}
}
}
}
}
This is my program that reads any input text file, counts how many words there are in the file, and sorts them using these four sorts: Merge sort, Java sort, Bubble sort, and selection sort. After it is done sorting, it displays the results in an output file specified by the user. The output file shows the Total words, and the time that the program took to sort the file using these different sorts. My question is how do I get the wall clock and CPU time for this program? Also, how would I get it to show up on my output file results? Code BelowStep by Step Solution
There are 3 Steps involved in it
Step: 1
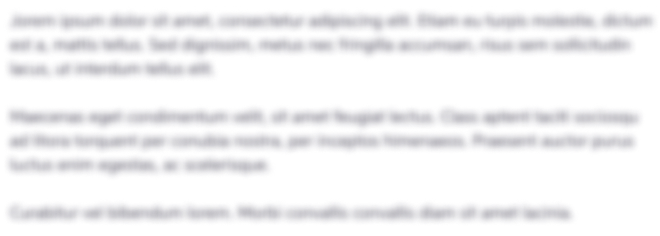
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started