Question
Modify the compareTo method so it orders Student objects based on the lexicographic ordering of the name variable. Without modification to the main method, the
Modify the compareTo method so it orders Student objects based on the lexicographic ordering of the name variable. Without modification to the main method, the program should now output the students ordered by name and number
import java.util.Arrays;
public class StudentTest
{
public static void main(String[] args)
{
Scanner170 keyboard = new Scanner170(System.in);
Student[] s = new Student[5];
s[0] = new Student("Mark" , 329);
s[1] = new Student("Roy" , 543);
s[2] = new Student("Bob" , 237);
s[3] = new Student("Sam" , 764);
s[4] = new Student("John" , 642);
Student2[] ss = new Student2[5];
ss[0] = new Student2("Mark" , 329);
ss[1] = new Student2("Roy" , 543);
ss[2] = new Student2("Bob" , 237);
ss[3] = new Student2("Sam" , 764);
ss[4] = new Student2("John" , 642);
Arrays.sort(s);
for(Student s1:s )
System.out.println(s1);
System.out.println(" ");
Arrays.sort(ss);
for(Student2 ss1:ss )
System.out.println(ss1);
}
}
______
public class Student extends Person2 implements Comparable
{
private int studentNumber;
public Student( )
{
super( ); // discussed later - calls a Person constructor
studentNumber = 0;//Indicating no number yet
}
public Student(String name, int initialStudentNumber)
{
super(name); // discussed later - calls a Person constructor
studentNumber = initialStudentNumber;
}
public void reset(String newName, int newStudentNumber)
{
setName(newName); // setName inherited from Person
studentNumber = newStudentNumber;
}
public int getStudentNumber( )
{
return studentNumber;
}
public void setStudentNumber(int newStudentNumber)
{
studentNumber = newStudentNumber;
}
@Override // have Java check that the writeOutput signature is correct for overriding
public void writeOutput( ) // method that overrides Person's writeOutput
{
System.out.println("Name: " + getName( )); // getName inherited from Person
System.out.println("Student Number: " + studentNumber);
}
public boolean equals(Student otherStudent)
{
return this.hasSameName(otherStudent) && // hasSameName inherited from Person
(this.studentNumber == otherStudent.studentNumber);
}
@Override // have Java check the equals method signature
public boolean equals(Object object)
{
if (object == null)
return false;
else if (!(object instanceof Student))
return false;
else
{
Student otherStudent = (Student) object;
return (this.hasSameName(otherStudent)
&& (this.studentNumber == otherStudent.studentNumber));
}
}
public int compareTo(Object object)
{
if (object != null && object instanceof Student)
{
Student otherStudent = (Student) object;
if (getName().compareTo(otherStudent.getName())>0)
{return 1;}
else if (getName().compareTo(otherStudent.getName())>0)
{ return -1;}
else {return 0;}
}
return -1;
}
@Override
public String toString( )
{
return "Name: " + getName( ) + // getName inherited from Person
" Student number: " + studentNumber;
}
}
__________
public class Student2 extends Person2 implements Comparable
{
private int studentNumber;
public Student2( )
{
super( ); // discussed later - calls a Person constructor
studentNumber = 0;//Indicating no number yet
}
public Student2(String name, int initialStudentNumber)
{
super(name); // discussed later - calls a Person constructor
studentNumber = initialStudentNumber;
}
public void reset(String newName, int newStudentNumber)
{
setName(newName); // setName inherited from Person
studentNumber = newStudentNumber;
}
public int getStudentNumber( )
{
return studentNumber;
}
public void setStudentNumber(int newStudentNumber)
{
studentNumber = newStudentNumber;
}
@Override // have Java check that the writeOutput signature is correct for overriding
public void writeOutput( ) // method that overrides Person's writeOutput
{
System.out.println("Name: " + getName( )); // getName inherited from Person
System.out.println("Student Number: " + studentNumber);
}
public boolean equals(Student2 otherStudent)
{
return this.hasSameName(otherStudent) && // hasSameName inherited from Person
(this.studentNumber == otherStudent.studentNumber);
}
@Override
public String toString( )
{
return "Name: " + getName( ) + // getName inherited from Person
" Student number: " + studentNumber;
}
public boolean equals2(Object object) // This can be overriden if it is the only equals method
{
boolean isEqual = false;
if ((object != null) && (object instanceof Student))
{
Student2 otherStudent = (Student2) object;
isEqual = this.hasSameName(otherStudent) &&
(this.studentNumber ==
otherStudent.studentNumber);
// check instance variable equality to determine true/false
}
return isEqual;
}
public int compareTo(Object object)
{
if (object != null && object instanceof Student)
{
Student2 otherStudent = (Student2) object;
if (studentNumber>otherStudent.studentNumber)
return 1;
else if (studentNumber return -1; else return 0; } return -1; } }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
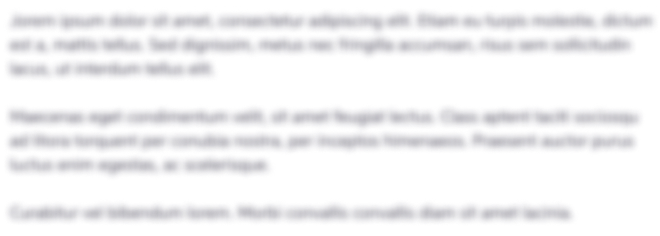
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started